mirror of
https://github.com/torvalds/linux
synced 2024-07-21 18:51:47 +00:00
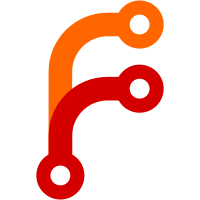
This patch changes to use __le types directly in the dlm message structure which is casted at the right dlm message buffer positions. The main goal what is reached here is to remove sparse warnings regarding to host to little byte order conversion or vice versa. Leaving those sparse issues ignored and always do it in out/in functionality tends to leave it unknown in which byte order the variable is being handled. Signed-off-by: Alexander Aring <aahringo@redhat.com> Signed-off-by: David Teigland <teigland@redhat.com>
67 lines
1.6 KiB
C
67 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/******************************************************************************
|
|
*******************************************************************************
|
|
**
|
|
** Copyright (C) 2005-2008 Red Hat, Inc. All rights reserved.
|
|
**
|
|
**
|
|
*******************************************************************************
|
|
******************************************************************************/
|
|
|
|
#include "dlm_internal.h"
|
|
#include "rcom.h"
|
|
#include "util.h"
|
|
|
|
#define DLM_ERRNO_EDEADLK 35
|
|
#define DLM_ERRNO_EBADR 53
|
|
#define DLM_ERRNO_EBADSLT 57
|
|
#define DLM_ERRNO_EPROTO 71
|
|
#define DLM_ERRNO_EOPNOTSUPP 95
|
|
#define DLM_ERRNO_ETIMEDOUT 110
|
|
#define DLM_ERRNO_EINPROGRESS 115
|
|
|
|
/* higher errno values are inconsistent across architectures, so select
|
|
one set of values for on the wire */
|
|
|
|
int to_dlm_errno(int err)
|
|
{
|
|
switch (err) {
|
|
case -EDEADLK:
|
|
return -DLM_ERRNO_EDEADLK;
|
|
case -EBADR:
|
|
return -DLM_ERRNO_EBADR;
|
|
case -EBADSLT:
|
|
return -DLM_ERRNO_EBADSLT;
|
|
case -EPROTO:
|
|
return -DLM_ERRNO_EPROTO;
|
|
case -EOPNOTSUPP:
|
|
return -DLM_ERRNO_EOPNOTSUPP;
|
|
case -ETIMEDOUT:
|
|
return -DLM_ERRNO_ETIMEDOUT;
|
|
case -EINPROGRESS:
|
|
return -DLM_ERRNO_EINPROGRESS;
|
|
}
|
|
return err;
|
|
}
|
|
|
|
int from_dlm_errno(int err)
|
|
{
|
|
switch (err) {
|
|
case -DLM_ERRNO_EDEADLK:
|
|
return -EDEADLK;
|
|
case -DLM_ERRNO_EBADR:
|
|
return -EBADR;
|
|
case -DLM_ERRNO_EBADSLT:
|
|
return -EBADSLT;
|
|
case -DLM_ERRNO_EPROTO:
|
|
return -EPROTO;
|
|
case -DLM_ERRNO_EOPNOTSUPP:
|
|
return -EOPNOTSUPP;
|
|
case -DLM_ERRNO_ETIMEDOUT:
|
|
return -ETIMEDOUT;
|
|
case -DLM_ERRNO_EINPROGRESS:
|
|
return -EINPROGRESS;
|
|
}
|
|
return err;
|
|
}
|