mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:35:42 +00:00
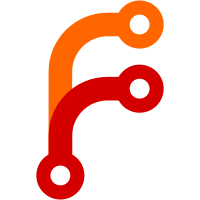
Make the user_namespace.o compilation depend on this option and move the init_user_ns into user.c file to make the kernel compile and work without the namespaces support. This make the user namespace code be organized similar to other namespaces'. Also mask the USER_NS option as "depend on NAMESPACES". [akpm@linux-foundation.org: coding-style fixes] Signed-off-by: Pavel Emelyanov <xemul@openvz.org> Acked-by: Serge Hallyn <serue@us.ibm.com> Cc: Cedric Le Goater <clg@fr.ibm.com> Cc: "Eric W. Biederman" <ebiederm@xmission.com> Cc: Herbert Poetzl <herbert@13thfloor.at> Cc: Kirill Korotaev <dev@sw.ru> Cc: Sukadev Bhattiprolu <sukadev@us.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
76 lines
1.6 KiB
C
76 lines
1.6 KiB
C
/*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation, version 2 of the
|
|
* License.
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/version.h>
|
|
#include <linux/nsproxy.h>
|
|
#include <linux/user_namespace.h>
|
|
|
|
/*
|
|
* Clone a new ns copying an original user ns, setting refcount to 1
|
|
* @old_ns: namespace to clone
|
|
* Return NULL on error (failure to kmalloc), new ns otherwise
|
|
*/
|
|
static struct user_namespace *clone_user_ns(struct user_namespace *old_ns)
|
|
{
|
|
struct user_namespace *ns;
|
|
struct user_struct *new_user;
|
|
int n;
|
|
|
|
ns = kmalloc(sizeof(struct user_namespace), GFP_KERNEL);
|
|
if (!ns)
|
|
return ERR_PTR(-ENOMEM);
|
|
|
|
kref_init(&ns->kref);
|
|
|
|
for (n = 0; n < UIDHASH_SZ; ++n)
|
|
INIT_HLIST_HEAD(ns->uidhash_table + n);
|
|
|
|
/* Insert new root user. */
|
|
ns->root_user = alloc_uid(ns, 0);
|
|
if (!ns->root_user) {
|
|
kfree(ns);
|
|
return ERR_PTR(-ENOMEM);
|
|
}
|
|
|
|
/* Reset current->user with a new one */
|
|
new_user = alloc_uid(ns, current->uid);
|
|
if (!new_user) {
|
|
free_uid(ns->root_user);
|
|
kfree(ns);
|
|
return ERR_PTR(-ENOMEM);
|
|
}
|
|
|
|
switch_uid(new_user);
|
|
return ns;
|
|
}
|
|
|
|
struct user_namespace * copy_user_ns(int flags, struct user_namespace *old_ns)
|
|
{
|
|
struct user_namespace *new_ns;
|
|
|
|
BUG_ON(!old_ns);
|
|
get_user_ns(old_ns);
|
|
|
|
if (!(flags & CLONE_NEWUSER))
|
|
return old_ns;
|
|
|
|
new_ns = clone_user_ns(old_ns);
|
|
|
|
put_user_ns(old_ns);
|
|
return new_ns;
|
|
}
|
|
|
|
void free_user_ns(struct kref *kref)
|
|
{
|
|
struct user_namespace *ns;
|
|
|
|
ns = container_of(kref, struct user_namespace, kref);
|
|
release_uids(ns);
|
|
kfree(ns);
|
|
}
|