mirror of
https://github.com/torvalds/linux
synced 2024-10-19 09:49:29 +00:00
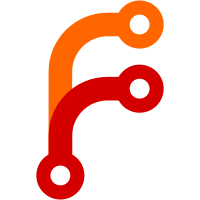
The replacement of <asm/pgrable.h> with <linux/pgtable.h> made the include of the latter in the middle of asm includes. Fix this up with the aid of the below script and manual adjustments here and there. import sys import re if len(sys.argv) is not 3: print "USAGE: %s <file> <header>" % (sys.argv[0]) sys.exit(1) hdr_to_move="#include <linux/%s>" % sys.argv[2] moved = False in_hdrs = False with open(sys.argv[1], "r") as f: lines = f.readlines() for _line in lines: line = _line.rstrip(' ') if line == hdr_to_move: continue if line.startswith("#include <linux/"): in_hdrs = True elif not moved and in_hdrs: moved = True print hdr_to_move print line Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Cc: Arnd Bergmann <arnd@arndb.de> Cc: Borislav Petkov <bp@alien8.de> Cc: Brian Cain <bcain@codeaurora.org> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Chris Zankel <chris@zankel.net> Cc: "David S. Miller" <davem@davemloft.net> Cc: Geert Uytterhoeven <geert@linux-m68k.org> Cc: Greentime Hu <green.hu@gmail.com> Cc: Greg Ungerer <gerg@linux-m68k.org> Cc: Guan Xuetao <gxt@pku.edu.cn> Cc: Guo Ren <guoren@kernel.org> Cc: Heiko Carstens <heiko.carstens@de.ibm.com> Cc: Helge Deller <deller@gmx.de> Cc: Ingo Molnar <mingo@redhat.com> Cc: Ley Foon Tan <ley.foon.tan@intel.com> Cc: Mark Salter <msalter@redhat.com> Cc: Matthew Wilcox <willy@infradead.org> Cc: Matt Turner <mattst88@gmail.com> Cc: Max Filippov <jcmvbkbc@gmail.com> Cc: Michael Ellerman <mpe@ellerman.id.au> Cc: Michal Simek <monstr@monstr.eu> Cc: Nick Hu <nickhu@andestech.com> Cc: Paul Walmsley <paul.walmsley@sifive.com> Cc: Richard Weinberger <richard@nod.at> Cc: Rich Felker <dalias@libc.org> Cc: Russell King <linux@armlinux.org.uk> Cc: Stafford Horne <shorne@gmail.com> Cc: Thomas Bogendoerfer <tsbogend@alpha.franken.de> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Tony Luck <tony.luck@intel.com> Cc: Vincent Chen <deanbo422@gmail.com> Cc: Vineet Gupta <vgupta@synopsys.com> Cc: Will Deacon <will@kernel.org> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Link: http://lkml.kernel.org/r/20200514170327.31389-4-rppt@kernel.org Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
60 lines
2.4 KiB
C
60 lines
2.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/* io-unit.h: Definitions for the sun4d IO-UNIT.
|
|
*
|
|
* Copyright (C) 1997,1998 Jakub Jelinek (jj@sunsite.mff.cuni.cz)
|
|
*/
|
|
#ifndef _SPARC_IO_UNIT_H
|
|
#define _SPARC_IO_UNIT_H
|
|
|
|
#include <linux/spinlock.h>
|
|
#include <linux/pgtable.h>
|
|
#include <asm/page.h>
|
|
|
|
/* The io-unit handles all virtual to physical address translations
|
|
* that occur between the SBUS and physical memory. Access by
|
|
* the cpu to IO registers and similar go over the xdbus so are
|
|
* translated by the on chip SRMMU. The io-unit and the srmmu do
|
|
* not need to have the same translations at all, in fact most
|
|
* of the time the translations they handle are a disjunct set.
|
|
* Basically the io-unit handles all dvma sbus activity.
|
|
*/
|
|
|
|
/* AIEEE, unlike the nice sun4m, these monsters have
|
|
fixed DMA range 64M */
|
|
|
|
#define IOUNIT_DMA_BASE 0xfc000000 /* TOP - 64M */
|
|
#define IOUNIT_DMA_SIZE 0x04000000 /* 64M */
|
|
/* We use last 1M for sparc_dvma_malloc */
|
|
#define IOUNIT_DVMA_SIZE 0x00100000 /* 1M */
|
|
|
|
/* The format of an iopte in the external page tables */
|
|
#define IOUPTE_PAGE 0xffffff00 /* Physical page number (PA[35:12]) */
|
|
#define IOUPTE_CACHE 0x00000080 /* Cached (in Viking/MXCC) */
|
|
/* XXX Jakub, find out how to program SBUS streaming cache on XDBUS/sun4d.
|
|
* XXX Actually, all you should need to do is find out where the registers
|
|
* XXX are and copy over the sparc64 implementation I wrote. There may be
|
|
* XXX some horrible hwbugs though, so be careful. -DaveM
|
|
*/
|
|
#define IOUPTE_STREAM 0x00000040 /* Translation can use streaming cache */
|
|
#define IOUPTE_INTRA 0x00000008 /* SBUS direct slot->slot transfer */
|
|
#define IOUPTE_WRITE 0x00000004 /* Writeable */
|
|
#define IOUPTE_VALID 0x00000002 /* IOPTE is valid */
|
|
#define IOUPTE_PARITY 0x00000001 /* Parity is checked during DVMA */
|
|
|
|
struct iounit_struct {
|
|
unsigned long bmap[(IOUNIT_DMA_SIZE >> (PAGE_SHIFT + 3)) / sizeof(unsigned long)];
|
|
spinlock_t lock;
|
|
iopte_t __iomem *page_table;
|
|
unsigned long rotor[3];
|
|
unsigned long limit[4];
|
|
};
|
|
|
|
#define IOUNIT_BMAP1_START 0x00000000
|
|
#define IOUNIT_BMAP1_END (IOUNIT_DMA_SIZE >> (PAGE_SHIFT + 1))
|
|
#define IOUNIT_BMAP2_START IOUNIT_BMAP1_END
|
|
#define IOUNIT_BMAP2_END IOUNIT_BMAP2_START + (IOUNIT_DMA_SIZE >> (PAGE_SHIFT + 2))
|
|
#define IOUNIT_BMAPM_START IOUNIT_BMAP2_END
|
|
#define IOUNIT_BMAPM_END ((IOUNIT_DMA_SIZE - IOUNIT_DVMA_SIZE) >> PAGE_SHIFT)
|
|
|
|
#endif /* !(_SPARC_IO_UNIT_H) */
|