mirror of
https://github.com/torvalds/linux
synced 2024-07-21 18:51:47 +00:00
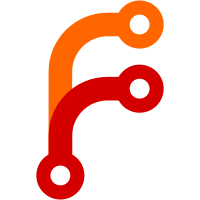
Pull poll annotations from Al Viro: "This introduces a __bitwise type for POLL### bitmap, and propagates the annotations through the tree. Most of that stuff is as simple as 'make ->poll() instances return __poll_t and do the same to local variables used to hold the future return value'. Some of the obvious brainos found in process are fixed (e.g. POLLIN misspelled as POLL_IN). At that point the amount of sparse warnings is low and most of them are for genuine bugs - e.g. ->poll() instance deciding to return -EINVAL instead of a bitmap. I hadn't touched those in this series - it's large enough as it is. Another problem it has caught was eventpoll() ABI mess; select.c and eventpoll.c assumed that corresponding POLL### and EPOLL### were equal. That's true for some, but not all of them - EPOLL### are arch-independent, but POLL### are not. The last commit in this series separates userland POLL### values from the (now arch-independent) kernel-side ones, converting between them in the few places where they are copied to/from userland. AFAICS, this is the least disruptive fix preserving poll(2) ABI and making epoll() work on all architectures. As it is, it's simply broken on sparc - try to give it EPOLLWRNORM and it will trigger only on what would've triggered EPOLLWRBAND on other architectures. EPOLLWRBAND and EPOLLRDHUP, OTOH, are never triggered at all on sparc. With this patch they should work consistently on all architectures" * 'misc.poll' of git://git.kernel.org/pub/scm/linux/kernel/git/viro/vfs: (37 commits) make kernel-side POLL... arch-independent eventpoll: no need to mask the result of epi_item_poll() again eventpoll: constify struct epoll_event pointers debugging printk in sg_poll() uses %x to print POLL... bitmap annotate poll(2) guts 9p: untangle ->poll() mess ->si_band gets POLL... bitmap stored into a user-visible long field ring_buffer_poll_wait() return value used as return value of ->poll() the rest of drivers/*: annotate ->poll() instances media: annotate ->poll() instances fs: annotate ->poll() instances ipc, kernel, mm: annotate ->poll() instances net: annotate ->poll() instances apparmor: annotate ->poll() instances tomoyo: annotate ->poll() instances sound: annotate ->poll() instances acpi: annotate ->poll() instances crypto: annotate ->poll() instances block: annotate ->poll() instances x86: annotate ->poll() instances ...
340 lines
7.8 KiB
C
340 lines
7.8 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* fs/proc_namespace.c - handling of /proc/<pid>/{mounts,mountinfo,mountstats}
|
|
*
|
|
* In fact, that's a piece of procfs; it's *almost* isolated from
|
|
* the rest of fs/proc, but has rather close relationships with
|
|
* fs/namespace.c, thus here instead of fs/proc
|
|
*
|
|
*/
|
|
#include <linux/mnt_namespace.h>
|
|
#include <linux/nsproxy.h>
|
|
#include <linux/security.h>
|
|
#include <linux/fs_struct.h>
|
|
#include <linux/sched/task.h>
|
|
|
|
#include "proc/internal.h" /* only for get_proc_task() in ->open() */
|
|
|
|
#include "pnode.h"
|
|
#include "internal.h"
|
|
|
|
static __poll_t mounts_poll(struct file *file, poll_table *wait)
|
|
{
|
|
struct seq_file *m = file->private_data;
|
|
struct proc_mounts *p = m->private;
|
|
struct mnt_namespace *ns = p->ns;
|
|
__poll_t res = POLLIN | POLLRDNORM;
|
|
int event;
|
|
|
|
poll_wait(file, &p->ns->poll, wait);
|
|
|
|
event = READ_ONCE(ns->event);
|
|
if (m->poll_event != event) {
|
|
m->poll_event = event;
|
|
res |= POLLERR | POLLPRI;
|
|
}
|
|
|
|
return res;
|
|
}
|
|
|
|
struct proc_fs_info {
|
|
int flag;
|
|
const char *str;
|
|
};
|
|
|
|
static int show_sb_opts(struct seq_file *m, struct super_block *sb)
|
|
{
|
|
static const struct proc_fs_info fs_info[] = {
|
|
{ SB_SYNCHRONOUS, ",sync" },
|
|
{ SB_DIRSYNC, ",dirsync" },
|
|
{ SB_MANDLOCK, ",mand" },
|
|
{ SB_LAZYTIME, ",lazytime" },
|
|
{ 0, NULL }
|
|
};
|
|
const struct proc_fs_info *fs_infop;
|
|
|
|
for (fs_infop = fs_info; fs_infop->flag; fs_infop++) {
|
|
if (sb->s_flags & fs_infop->flag)
|
|
seq_puts(m, fs_infop->str);
|
|
}
|
|
|
|
return security_sb_show_options(m, sb);
|
|
}
|
|
|
|
static void show_mnt_opts(struct seq_file *m, struct vfsmount *mnt)
|
|
{
|
|
static const struct proc_fs_info mnt_info[] = {
|
|
{ MNT_NOSUID, ",nosuid" },
|
|
{ MNT_NODEV, ",nodev" },
|
|
{ MNT_NOEXEC, ",noexec" },
|
|
{ MNT_NOATIME, ",noatime" },
|
|
{ MNT_NODIRATIME, ",nodiratime" },
|
|
{ MNT_RELATIME, ",relatime" },
|
|
{ 0, NULL }
|
|
};
|
|
const struct proc_fs_info *fs_infop;
|
|
|
|
for (fs_infop = mnt_info; fs_infop->flag; fs_infop++) {
|
|
if (mnt->mnt_flags & fs_infop->flag)
|
|
seq_puts(m, fs_infop->str);
|
|
}
|
|
}
|
|
|
|
static inline void mangle(struct seq_file *m, const char *s)
|
|
{
|
|
seq_escape(m, s, " \t\n\\");
|
|
}
|
|
|
|
static void show_type(struct seq_file *m, struct super_block *sb)
|
|
{
|
|
mangle(m, sb->s_type->name);
|
|
if (sb->s_subtype && sb->s_subtype[0]) {
|
|
seq_putc(m, '.');
|
|
mangle(m, sb->s_subtype);
|
|
}
|
|
}
|
|
|
|
static int show_vfsmnt(struct seq_file *m, struct vfsmount *mnt)
|
|
{
|
|
struct proc_mounts *p = m->private;
|
|
struct mount *r = real_mount(mnt);
|
|
struct path mnt_path = { .dentry = mnt->mnt_root, .mnt = mnt };
|
|
struct super_block *sb = mnt_path.dentry->d_sb;
|
|
int err;
|
|
|
|
if (sb->s_op->show_devname) {
|
|
err = sb->s_op->show_devname(m, mnt_path.dentry);
|
|
if (err)
|
|
goto out;
|
|
} else {
|
|
mangle(m, r->mnt_devname ? r->mnt_devname : "none");
|
|
}
|
|
seq_putc(m, ' ');
|
|
/* mountpoints outside of chroot jail will give SEQ_SKIP on this */
|
|
err = seq_path_root(m, &mnt_path, &p->root, " \t\n\\");
|
|
if (err)
|
|
goto out;
|
|
seq_putc(m, ' ');
|
|
show_type(m, sb);
|
|
seq_puts(m, __mnt_is_readonly(mnt) ? " ro" : " rw");
|
|
err = show_sb_opts(m, sb);
|
|
if (err)
|
|
goto out;
|
|
show_mnt_opts(m, mnt);
|
|
if (sb->s_op->show_options)
|
|
err = sb->s_op->show_options(m, mnt_path.dentry);
|
|
seq_puts(m, " 0 0\n");
|
|
out:
|
|
return err;
|
|
}
|
|
|
|
static int show_mountinfo(struct seq_file *m, struct vfsmount *mnt)
|
|
{
|
|
struct proc_mounts *p = m->private;
|
|
struct mount *r = real_mount(mnt);
|
|
struct super_block *sb = mnt->mnt_sb;
|
|
struct path mnt_path = { .dentry = mnt->mnt_root, .mnt = mnt };
|
|
int err;
|
|
|
|
seq_printf(m, "%i %i %u:%u ", r->mnt_id, r->mnt_parent->mnt_id,
|
|
MAJOR(sb->s_dev), MINOR(sb->s_dev));
|
|
if (sb->s_op->show_path) {
|
|
err = sb->s_op->show_path(m, mnt->mnt_root);
|
|
if (err)
|
|
goto out;
|
|
} else {
|
|
seq_dentry(m, mnt->mnt_root, " \t\n\\");
|
|
}
|
|
seq_putc(m, ' ');
|
|
|
|
/* mountpoints outside of chroot jail will give SEQ_SKIP on this */
|
|
err = seq_path_root(m, &mnt_path, &p->root, " \t\n\\");
|
|
if (err)
|
|
goto out;
|
|
|
|
seq_puts(m, mnt->mnt_flags & MNT_READONLY ? " ro" : " rw");
|
|
show_mnt_opts(m, mnt);
|
|
|
|
/* Tagged fields ("foo:X" or "bar") */
|
|
if (IS_MNT_SHARED(r))
|
|
seq_printf(m, " shared:%i", r->mnt_group_id);
|
|
if (IS_MNT_SLAVE(r)) {
|
|
int master = r->mnt_master->mnt_group_id;
|
|
int dom = get_dominating_id(r, &p->root);
|
|
seq_printf(m, " master:%i", master);
|
|
if (dom && dom != master)
|
|
seq_printf(m, " propagate_from:%i", dom);
|
|
}
|
|
if (IS_MNT_UNBINDABLE(r))
|
|
seq_puts(m, " unbindable");
|
|
|
|
/* Filesystem specific data */
|
|
seq_puts(m, " - ");
|
|
show_type(m, sb);
|
|
seq_putc(m, ' ');
|
|
if (sb->s_op->show_devname) {
|
|
err = sb->s_op->show_devname(m, mnt->mnt_root);
|
|
if (err)
|
|
goto out;
|
|
} else {
|
|
mangle(m, r->mnt_devname ? r->mnt_devname : "none");
|
|
}
|
|
seq_puts(m, sb_rdonly(sb) ? " ro" : " rw");
|
|
err = show_sb_opts(m, sb);
|
|
if (err)
|
|
goto out;
|
|
if (sb->s_op->show_options)
|
|
err = sb->s_op->show_options(m, mnt->mnt_root);
|
|
seq_putc(m, '\n');
|
|
out:
|
|
return err;
|
|
}
|
|
|
|
static int show_vfsstat(struct seq_file *m, struct vfsmount *mnt)
|
|
{
|
|
struct proc_mounts *p = m->private;
|
|
struct mount *r = real_mount(mnt);
|
|
struct path mnt_path = { .dentry = mnt->mnt_root, .mnt = mnt };
|
|
struct super_block *sb = mnt_path.dentry->d_sb;
|
|
int err;
|
|
|
|
/* device */
|
|
if (sb->s_op->show_devname) {
|
|
seq_puts(m, "device ");
|
|
err = sb->s_op->show_devname(m, mnt_path.dentry);
|
|
if (err)
|
|
goto out;
|
|
} else {
|
|
if (r->mnt_devname) {
|
|
seq_puts(m, "device ");
|
|
mangle(m, r->mnt_devname);
|
|
} else
|
|
seq_puts(m, "no device");
|
|
}
|
|
|
|
/* mount point */
|
|
seq_puts(m, " mounted on ");
|
|
/* mountpoints outside of chroot jail will give SEQ_SKIP on this */
|
|
err = seq_path_root(m, &mnt_path, &p->root, " \t\n\\");
|
|
if (err)
|
|
goto out;
|
|
seq_putc(m, ' ');
|
|
|
|
/* file system type */
|
|
seq_puts(m, "with fstype ");
|
|
show_type(m, sb);
|
|
|
|
/* optional statistics */
|
|
if (sb->s_op->show_stats) {
|
|
seq_putc(m, ' ');
|
|
err = sb->s_op->show_stats(m, mnt_path.dentry);
|
|
}
|
|
|
|
seq_putc(m, '\n');
|
|
out:
|
|
return err;
|
|
}
|
|
|
|
static int mounts_open_common(struct inode *inode, struct file *file,
|
|
int (*show)(struct seq_file *, struct vfsmount *))
|
|
{
|
|
struct task_struct *task = get_proc_task(inode);
|
|
struct nsproxy *nsp;
|
|
struct mnt_namespace *ns = NULL;
|
|
struct path root;
|
|
struct proc_mounts *p;
|
|
struct seq_file *m;
|
|
int ret = -EINVAL;
|
|
|
|
if (!task)
|
|
goto err;
|
|
|
|
task_lock(task);
|
|
nsp = task->nsproxy;
|
|
if (!nsp || !nsp->mnt_ns) {
|
|
task_unlock(task);
|
|
put_task_struct(task);
|
|
goto err;
|
|
}
|
|
ns = nsp->mnt_ns;
|
|
get_mnt_ns(ns);
|
|
if (!task->fs) {
|
|
task_unlock(task);
|
|
put_task_struct(task);
|
|
ret = -ENOENT;
|
|
goto err_put_ns;
|
|
}
|
|
get_fs_root(task->fs, &root);
|
|
task_unlock(task);
|
|
put_task_struct(task);
|
|
|
|
ret = seq_open_private(file, &mounts_op, sizeof(struct proc_mounts));
|
|
if (ret)
|
|
goto err_put_path;
|
|
|
|
m = file->private_data;
|
|
m->poll_event = ns->event;
|
|
|
|
p = m->private;
|
|
p->ns = ns;
|
|
p->root = root;
|
|
p->show = show;
|
|
p->cached_event = ~0ULL;
|
|
|
|
return 0;
|
|
|
|
err_put_path:
|
|
path_put(&root);
|
|
err_put_ns:
|
|
put_mnt_ns(ns);
|
|
err:
|
|
return ret;
|
|
}
|
|
|
|
static int mounts_release(struct inode *inode, struct file *file)
|
|
{
|
|
struct seq_file *m = file->private_data;
|
|
struct proc_mounts *p = m->private;
|
|
path_put(&p->root);
|
|
put_mnt_ns(p->ns);
|
|
return seq_release_private(inode, file);
|
|
}
|
|
|
|
static int mounts_open(struct inode *inode, struct file *file)
|
|
{
|
|
return mounts_open_common(inode, file, show_vfsmnt);
|
|
}
|
|
|
|
static int mountinfo_open(struct inode *inode, struct file *file)
|
|
{
|
|
return mounts_open_common(inode, file, show_mountinfo);
|
|
}
|
|
|
|
static int mountstats_open(struct inode *inode, struct file *file)
|
|
{
|
|
return mounts_open_common(inode, file, show_vfsstat);
|
|
}
|
|
|
|
const struct file_operations proc_mounts_operations = {
|
|
.open = mounts_open,
|
|
.read = seq_read,
|
|
.llseek = seq_lseek,
|
|
.release = mounts_release,
|
|
.poll = mounts_poll,
|
|
};
|
|
|
|
const struct file_operations proc_mountinfo_operations = {
|
|
.open = mountinfo_open,
|
|
.read = seq_read,
|
|
.llseek = seq_lseek,
|
|
.release = mounts_release,
|
|
.poll = mounts_poll,
|
|
};
|
|
|
|
const struct file_operations proc_mountstats_operations = {
|
|
.open = mountstats_open,
|
|
.read = seq_read,
|
|
.llseek = seq_lseek,
|
|
.release = mounts_release,
|
|
};
|