mirror of
https://github.com/torvalds/linux
synced 2024-10-23 11:46:42 +00:00
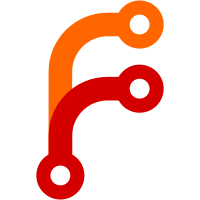
We were always looking at the running machine /proc/modules, even when processing a perf.data file, which only makes sense when we're doing 'perf record' and 'perf report' on the same machine, and in close sucession, or if we don't use modules at all, right Peter? ;-) Now, at 'perf record' time we read /proc/modules, find the long path for modules, and put them as PERF_MMAP events, just like we did to encode the reloc reference symbol for vmlinux. Talking about that now it is encoded in .pgoff, so that we can use .{start,len} to store the address boundaries for the kernel so that when we reconstruct the kmaps tree we can do lookups right away, without having to fixup the end of the kernel maps like we did in the past (and now only in perf record). One more step in the 'perf archive' direction when we'll finally be able to collect data in one machine and analyse in another. Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Frédéric Weisbecker <fweisbec@gmail.com> Cc: Mike Galbraith <efault@gmx.de> Cc: Peter Zijlstra <a.p.zijlstra@chello.nl> Cc: Paul Mackerras <paulus@samba.org> LKML-Reference: <1263396139-4798-1-git-send-email-acme@infradead.org> Signed-off-by: Ingo Molnar <mingo@elte.hu>
129 lines
2.6 KiB
C
129 lines
2.6 KiB
C
#ifndef __PERF_RECORD_H
|
|
#define __PERF_RECORD_H
|
|
|
|
#include <limits.h>
|
|
|
|
#include "../perf.h"
|
|
#include "map.h"
|
|
|
|
/*
|
|
* PERF_SAMPLE_IP | PERF_SAMPLE_TID | *
|
|
*/
|
|
struct ip_event {
|
|
struct perf_event_header header;
|
|
u64 ip;
|
|
u32 pid, tid;
|
|
unsigned char __more_data[];
|
|
};
|
|
|
|
struct mmap_event {
|
|
struct perf_event_header header;
|
|
u32 pid, tid;
|
|
u64 start;
|
|
u64 len;
|
|
u64 pgoff;
|
|
char filename[PATH_MAX];
|
|
};
|
|
|
|
struct comm_event {
|
|
struct perf_event_header header;
|
|
u32 pid, tid;
|
|
char comm[16];
|
|
};
|
|
|
|
struct fork_event {
|
|
struct perf_event_header header;
|
|
u32 pid, ppid;
|
|
u32 tid, ptid;
|
|
u64 time;
|
|
};
|
|
|
|
struct lost_event {
|
|
struct perf_event_header header;
|
|
u64 id;
|
|
u64 lost;
|
|
};
|
|
|
|
/*
|
|
* PERF_FORMAT_ENABLED | PERF_FORMAT_RUNNING | PERF_FORMAT_ID
|
|
*/
|
|
struct read_event {
|
|
struct perf_event_header header;
|
|
u32 pid, tid;
|
|
u64 value;
|
|
u64 time_enabled;
|
|
u64 time_running;
|
|
u64 id;
|
|
};
|
|
|
|
struct sample_event {
|
|
struct perf_event_header header;
|
|
u64 array[];
|
|
};
|
|
|
|
struct sample_data {
|
|
u64 ip;
|
|
u32 pid, tid;
|
|
u64 time;
|
|
u64 addr;
|
|
u64 id;
|
|
u64 stream_id;
|
|
u32 cpu;
|
|
u64 period;
|
|
struct ip_callchain *callchain;
|
|
u32 raw_size;
|
|
void *raw_data;
|
|
};
|
|
|
|
#define BUILD_ID_SIZE 20
|
|
|
|
struct build_id_event {
|
|
struct perf_event_header header;
|
|
u8 build_id[ALIGN(BUILD_ID_SIZE, sizeof(u64))];
|
|
char filename[];
|
|
};
|
|
|
|
typedef union event_union {
|
|
struct perf_event_header header;
|
|
struct ip_event ip;
|
|
struct mmap_event mmap;
|
|
struct comm_event comm;
|
|
struct fork_event fork;
|
|
struct lost_event lost;
|
|
struct read_event read;
|
|
struct sample_event sample;
|
|
} event_t;
|
|
|
|
struct events_stats {
|
|
u64 total;
|
|
u64 lost;
|
|
};
|
|
|
|
void event__print_totals(void);
|
|
|
|
struct perf_session;
|
|
|
|
typedef int (*event__handler_t)(event_t *event, struct perf_session *session);
|
|
|
|
int event__synthesize_thread(pid_t pid, event__handler_t process,
|
|
struct perf_session *session);
|
|
void event__synthesize_threads(event__handler_t process,
|
|
struct perf_session *session);
|
|
int event__synthesize_kernel_mmap(event__handler_t process,
|
|
struct perf_session *session,
|
|
const char *symbol_name);
|
|
int event__synthesize_modules(event__handler_t process,
|
|
struct perf_session *session);
|
|
|
|
int event__process_comm(event_t *self, struct perf_session *session);
|
|
int event__process_lost(event_t *self, struct perf_session *session);
|
|
int event__process_mmap(event_t *self, struct perf_session *session);
|
|
int event__process_task(event_t *self, struct perf_session *session);
|
|
|
|
struct addr_location;
|
|
int event__preprocess_sample(const event_t *self, struct perf_session *session,
|
|
struct addr_location *al, symbol_filter_t filter);
|
|
int event__parse_sample(event_t *event, u64 type, struct sample_data *data);
|
|
|
|
#endif /* __PERF_RECORD_H */
|