mirror of
https://github.com/torvalds/linux
synced 2024-07-21 18:51:47 +00:00
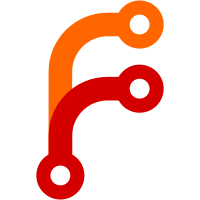
Each sample script sources functions.sh before parameters.sh which makes $APPEND undefined when trapping EXIT no matter in append mode or not. Due to this when sample scripts finished they always do "pgctrl reset" which resets pktgen config. So move trap to each script after sourcing parameters.sh and trap EXIT explicitly. Signed-off-by: J.J. Martzki <mars14850@gmail.com> Signed-off-by: David S. Miller <davem@davemloft.net>
104 lines
2.8 KiB
Bash
Executable file
104 lines
2.8 KiB
Bash
Executable file
#!/bin/bash
|
|
# SPDX-License-Identifier: GPL-2.0
|
|
#
|
|
# Simple example:
|
|
# * pktgen sending with single thread and single interface
|
|
# * flow variation via random UDP source port
|
|
#
|
|
basedir=`dirname $0`
|
|
source ${basedir}/functions.sh
|
|
root_check_run_with_sudo "$@"
|
|
|
|
# Parameter parsing via include
|
|
# - go look in parameters.sh to see which setting are avail
|
|
# - required param is the interface "-i" stored in $DEV
|
|
source ${basedir}/parameters.sh
|
|
|
|
# Trap EXIT first
|
|
trap_exit
|
|
|
|
#
|
|
# Set some default params, if they didn't get set
|
|
if [ -z "$DEST_IP" ]; then
|
|
[ -z "$IP6" ] && DEST_IP="198.18.0.42" || DEST_IP="FD00::1"
|
|
fi
|
|
[ -z "$CLONE_SKB" ] && CLONE_SKB="0"
|
|
# Example enforce param "-m" for dst_mac
|
|
[ -z "$DST_MAC" ] && usage && err 2 "Must specify -m dst_mac"
|
|
[ -z "$COUNT" ] && COUNT="100000" # Zero means indefinitely
|
|
if [ -n "$DEST_IP" ]; then
|
|
validate_addr${IP6} $DEST_IP
|
|
read -r DST_MIN DST_MAX <<< $(parse_addr${IP6} $DEST_IP)
|
|
fi
|
|
if [ -n "$DST_PORT" ]; then
|
|
read -r UDP_DST_MIN UDP_DST_MAX <<< $(parse_ports $DST_PORT)
|
|
validate_ports $UDP_DST_MIN $UDP_DST_MAX
|
|
fi
|
|
|
|
# Flow variation random source port between min and max
|
|
UDP_SRC_MIN=9
|
|
UDP_SRC_MAX=109
|
|
|
|
# General cleanup everything since last run
|
|
# (especially important if other threads were configured by other scripts)
|
|
[ -z "$APPEND" ] && pg_ctrl "reset"
|
|
|
|
# Add remove all other devices and add_device $DEV to thread 0
|
|
thread=0
|
|
[ -z "$APPEND" ] && pg_thread $thread "rem_device_all"
|
|
pg_thread $thread "add_device" $DEV
|
|
|
|
# How many packets to send (zero means indefinitely)
|
|
pg_set $DEV "count $COUNT"
|
|
|
|
# Reduce alloc cost by sending same SKB many times
|
|
# - this obviously affects the randomness within the packet
|
|
pg_set $DEV "clone_skb $CLONE_SKB"
|
|
|
|
# Set packet size
|
|
pg_set $DEV "pkt_size $PKT_SIZE"
|
|
|
|
# Delay between packets (zero means max speed)
|
|
pg_set $DEV "delay $DELAY"
|
|
|
|
# Flag example disabling timestamping
|
|
pg_set $DEV "flag NO_TIMESTAMP"
|
|
|
|
# Destination
|
|
pg_set $DEV "dst_mac $DST_MAC"
|
|
pg_set $DEV "dst${IP6}_min $DST_MIN"
|
|
pg_set $DEV "dst${IP6}_max $DST_MAX"
|
|
|
|
if [ -n "$DST_PORT" ]; then
|
|
# Single destination port or random port range
|
|
pg_set $DEV "flag UDPDST_RND"
|
|
pg_set $DEV "udp_dst_min $UDP_DST_MIN"
|
|
pg_set $DEV "udp_dst_max $UDP_DST_MAX"
|
|
fi
|
|
|
|
[ ! -z "$UDP_CSUM" ] && pg_set $dev "flag UDPCSUM"
|
|
|
|
# Setup random UDP port src range
|
|
pg_set $DEV "flag UDPSRC_RND"
|
|
pg_set $DEV "udp_src_min $UDP_SRC_MIN"
|
|
pg_set $DEV "udp_src_max $UDP_SRC_MAX"
|
|
|
|
# Run if user hits control-c
|
|
function print_result() {
|
|
# Print results
|
|
echo "Result device: $DEV"
|
|
cat /proc/net/pktgen/$DEV
|
|
}
|
|
# trap keyboard interrupt (Ctrl-C)
|
|
trap true SIGINT
|
|
|
|
if [ -z "$APPEND" ]; then
|
|
# start_run
|
|
echo "Running... ctrl^C to stop" >&2
|
|
pg_ctrl "start"
|
|
echo "Done" >&2
|
|
|
|
print_result
|
|
else
|
|
echo "Append mode: config done. Do more or use 'pg_ctrl start' to run"
|
|
fi |