mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
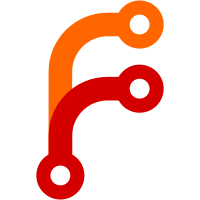
Fix all files in samples/bpf to include libbpf header files with the bpf/
prefix, to be consistent with external users of the library. Also ensure
that all includes of exported libbpf header files (those that are exported
on 'make install' of the library) use bracketed includes instead of quoted.
To make sure no new files are introduced that doesn't include the bpf/
prefix in its include, remove tools/lib/bpf from the include path entirely,
and use tools/lib instead.
Fixes: 6910d7d386
("selftests/bpf: Ensure bpf_helper_defs.h are taken from selftests dir")
Signed-off-by: Toke Høiland-Jørgensen <toke@redhat.com>
Signed-off-by: Alexei Starovoitov <ast@kernel.org>
Acked-by: Jesper Dangaard Brouer <brouer@redhat.com>
Acked-by: Andrii Nakryiko <andriin@fb.com>
Link: https://lore.kernel.org/bpf/157952560911.1683545.8795966751309534150.stgit@toke.dk
92 lines
2.6 KiB
C
92 lines
2.6 KiB
C
#define KBUILD_MODNAME "foo"
|
|
#include <uapi/linux/bpf.h>
|
|
#include <uapi/linux/if_ether.h>
|
|
#include <uapi/linux/if_packet.h>
|
|
#include <uapi/linux/ip.h>
|
|
#include <uapi/linux/in.h>
|
|
#include <uapi/linux/tcp.h>
|
|
#include <uapi/linux/filter.h>
|
|
#include <uapi/linux/pkt_cls.h>
|
|
#include <bpf/bpf_helpers.h>
|
|
#include "bpf_legacy.h"
|
|
|
|
/* compiler workaround */
|
|
#define _htonl __builtin_bswap32
|
|
|
|
static inline void set_dst_mac(struct __sk_buff *skb, char *mac)
|
|
{
|
|
bpf_skb_store_bytes(skb, 0, mac, ETH_ALEN, 1);
|
|
}
|
|
|
|
#define IP_CSUM_OFF (ETH_HLEN + offsetof(struct iphdr, check))
|
|
#define TOS_OFF (ETH_HLEN + offsetof(struct iphdr, tos))
|
|
|
|
static inline void set_ip_tos(struct __sk_buff *skb, __u8 new_tos)
|
|
{
|
|
__u8 old_tos = load_byte(skb, TOS_OFF);
|
|
|
|
bpf_l3_csum_replace(skb, IP_CSUM_OFF, htons(old_tos), htons(new_tos), 2);
|
|
bpf_skb_store_bytes(skb, TOS_OFF, &new_tos, sizeof(new_tos), 0);
|
|
}
|
|
|
|
#define TCP_CSUM_OFF (ETH_HLEN + sizeof(struct iphdr) + offsetof(struct tcphdr, check))
|
|
#define IP_SRC_OFF (ETH_HLEN + offsetof(struct iphdr, saddr))
|
|
|
|
#define IS_PSEUDO 0x10
|
|
|
|
static inline void set_tcp_ip_src(struct __sk_buff *skb, __u32 new_ip)
|
|
{
|
|
__u32 old_ip = _htonl(load_word(skb, IP_SRC_OFF));
|
|
|
|
bpf_l4_csum_replace(skb, TCP_CSUM_OFF, old_ip, new_ip, IS_PSEUDO | sizeof(new_ip));
|
|
bpf_l3_csum_replace(skb, IP_CSUM_OFF, old_ip, new_ip, sizeof(new_ip));
|
|
bpf_skb_store_bytes(skb, IP_SRC_OFF, &new_ip, sizeof(new_ip), 0);
|
|
}
|
|
|
|
#define TCP_DPORT_OFF (ETH_HLEN + sizeof(struct iphdr) + offsetof(struct tcphdr, dest))
|
|
static inline void set_tcp_dest_port(struct __sk_buff *skb, __u16 new_port)
|
|
{
|
|
__u16 old_port = htons(load_half(skb, TCP_DPORT_OFF));
|
|
|
|
bpf_l4_csum_replace(skb, TCP_CSUM_OFF, old_port, new_port, sizeof(new_port));
|
|
bpf_skb_store_bytes(skb, TCP_DPORT_OFF, &new_port, sizeof(new_port), 0);
|
|
}
|
|
|
|
SEC("classifier")
|
|
int bpf_prog1(struct __sk_buff *skb)
|
|
{
|
|
__u8 proto = load_byte(skb, ETH_HLEN + offsetof(struct iphdr, protocol));
|
|
long *value;
|
|
|
|
if (proto == IPPROTO_TCP) {
|
|
set_ip_tos(skb, 8);
|
|
set_tcp_ip_src(skb, 0xA010101);
|
|
set_tcp_dest_port(skb, 5001);
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
SEC("redirect_xmit")
|
|
int _redirect_xmit(struct __sk_buff *skb)
|
|
{
|
|
return bpf_redirect(skb->ifindex + 1, 0);
|
|
}
|
|
SEC("redirect_recv")
|
|
int _redirect_recv(struct __sk_buff *skb)
|
|
{
|
|
return bpf_redirect(skb->ifindex + 1, 1);
|
|
}
|
|
SEC("clone_redirect_xmit")
|
|
int _clone_redirect_xmit(struct __sk_buff *skb)
|
|
{
|
|
bpf_clone_redirect(skb, skb->ifindex + 1, 0);
|
|
return TC_ACT_SHOT;
|
|
}
|
|
SEC("clone_redirect_recv")
|
|
int _clone_redirect_recv(struct __sk_buff *skb)
|
|
{
|
|
bpf_clone_redirect(skb, skb->ifindex + 1, 1);
|
|
return TC_ACT_SHOT;
|
|
}
|
|
char _license[] SEC("license") = "GPL";
|