mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
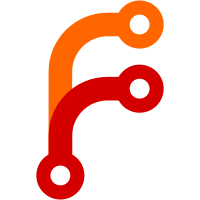
Increase the parallelism level for pwork clients to the workqueue defaults so that we can take advantage of computers with a lot of CPUs and a lot of hardware. On fast systems this will speed up quotacheck by a large factor, and the following posteof/cowblocks cleanup series will use the functionality presented in this patch to run garbage collection as quickly as possible. We do this by switching the pwork workqueue to unbounded, since the current user (quotacheck) runs lengthy scans for each work item and we don't care about dispatching the work on a warm cpu cache or anything like that. Also set WQ_SYSFS so that we can monitor where the wq is running. Signed-off-by: Darrick J. Wong <djwong@kernel.org> Reviewed-by: Christoph Hellwig <hch@lst.de> Reviewed-by: Brian Foster <bfoster@redhat.com>
60 lines
1.4 KiB
C
60 lines
1.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
* Copyright (C) 2019 Oracle. All Rights Reserved.
|
|
* Author: Darrick J. Wong <darrick.wong@oracle.com>
|
|
*/
|
|
#ifndef __XFS_PWORK_H__
|
|
#define __XFS_PWORK_H__
|
|
|
|
struct xfs_pwork;
|
|
struct xfs_mount;
|
|
|
|
typedef int (*xfs_pwork_work_fn)(struct xfs_mount *mp, struct xfs_pwork *pwork);
|
|
|
|
/*
|
|
* Parallel work coordination structure.
|
|
*/
|
|
struct xfs_pwork_ctl {
|
|
struct workqueue_struct *wq;
|
|
struct xfs_mount *mp;
|
|
xfs_pwork_work_fn work_fn;
|
|
struct wait_queue_head poll_wait;
|
|
atomic_t nr_work;
|
|
int error;
|
|
};
|
|
|
|
/*
|
|
* Embed this parallel work control item inside your own work structure,
|
|
* then queue work with it.
|
|
*/
|
|
struct xfs_pwork {
|
|
struct work_struct work;
|
|
struct xfs_pwork_ctl *pctl;
|
|
};
|
|
|
|
#define XFS_PWORK_SINGLE_THREADED { .pctl = NULL }
|
|
|
|
/* Have we been told to abort? */
|
|
static inline bool
|
|
xfs_pwork_ctl_want_abort(
|
|
struct xfs_pwork_ctl *pctl)
|
|
{
|
|
return pctl && pctl->error;
|
|
}
|
|
|
|
/* Have we been told to abort? */
|
|
static inline bool
|
|
xfs_pwork_want_abort(
|
|
struct xfs_pwork *pwork)
|
|
{
|
|
return xfs_pwork_ctl_want_abort(pwork->pctl);
|
|
}
|
|
|
|
int xfs_pwork_init(struct xfs_mount *mp, struct xfs_pwork_ctl *pctl,
|
|
xfs_pwork_work_fn work_fn, const char *tag);
|
|
void xfs_pwork_queue(struct xfs_pwork_ctl *pctl, struct xfs_pwork *pwork);
|
|
int xfs_pwork_destroy(struct xfs_pwork_ctl *pctl);
|
|
void xfs_pwork_poll(struct xfs_pwork_ctl *pctl);
|
|
|
|
#endif /* __XFS_PWORK_H__ */
|