mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
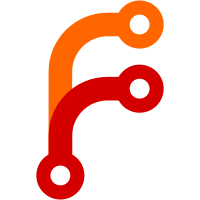
Convert to struct mnt_idmap.
Remove legacy file_mnt_user_ns() and mnt_user_ns().
Last cycle we merged the necessary infrastructure in
256c8aed2b
("fs: introduce dedicated idmap type for mounts").
This is just the conversion to struct mnt_idmap.
Currently we still pass around the plain namespace that was attached to a
mount. This is in general pretty convenient but it makes it easy to
conflate namespaces that are relevant on the filesystem with namespaces
that are relevent on the mount level. Especially for non-vfs developers
without detailed knowledge in this area this can be a potential source for
bugs.
Once the conversion to struct mnt_idmap is done all helpers down to the
really low-level helpers will take a struct mnt_idmap argument instead of
two namespace arguments. This way it becomes impossible to conflate the two
eliminating the possibility of any bugs. All of the vfs and all filesystems
only operate on struct mnt_idmap.
Acked-by: Dave Chinner <dchinner@redhat.com>
Reviewed-by: Christoph Hellwig <hch@lst.de>
Signed-off-by: Christian Brauner (Microsoft) <brauner@kernel.org>
69 lines
2.1 KiB
C
69 lines
2.1 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2000-2001 Silicon Graphics, Inc. All Rights Reserved.
|
|
*/
|
|
#ifndef __XFS_ITABLE_H__
|
|
#define __XFS_ITABLE_H__
|
|
|
|
/* In-memory representation of a userspace request for batch inode data. */
|
|
struct xfs_ibulk {
|
|
struct xfs_mount *mp;
|
|
struct mnt_idmap *idmap;
|
|
void __user *ubuffer; /* user output buffer */
|
|
xfs_ino_t startino; /* start with this inode */
|
|
unsigned int icount; /* number of elements in ubuffer */
|
|
unsigned int ocount; /* number of records returned */
|
|
unsigned int flags; /* see XFS_IBULK_FLAG_* */
|
|
};
|
|
|
|
/* Only iterate within the same AG as startino */
|
|
#define XFS_IBULK_SAME_AG (1U << 0)
|
|
|
|
/* Fill out the bs_extents64 field if set. */
|
|
#define XFS_IBULK_NREXT64 (1U << 1)
|
|
|
|
/*
|
|
* Advance the user buffer pointer by one record of the given size. If the
|
|
* buffer is now full, return the appropriate error code.
|
|
*/
|
|
static inline int
|
|
xfs_ibulk_advance(
|
|
struct xfs_ibulk *breq,
|
|
size_t bytes)
|
|
{
|
|
char __user *b = breq->ubuffer;
|
|
|
|
breq->ubuffer = b + bytes;
|
|
breq->ocount++;
|
|
return breq->ocount == breq->icount ? -ECANCELED : 0;
|
|
}
|
|
|
|
/*
|
|
* Return stat information in bulk (by-inode) for the filesystem.
|
|
*/
|
|
|
|
/*
|
|
* Return codes for the formatter function are 0 to continue iterating, and
|
|
* non-zero to stop iterating. Any non-zero value will be passed up to the
|
|
* bulkstat/inumbers caller. The special value -ECANCELED can be used to stop
|
|
* iteration, as neither bulkstat nor inumbers will ever generate that error
|
|
* code on their own.
|
|
*/
|
|
|
|
typedef int (*bulkstat_one_fmt_pf)(struct xfs_ibulk *breq,
|
|
const struct xfs_bulkstat *bstat);
|
|
|
|
int xfs_bulkstat_one(struct xfs_ibulk *breq, bulkstat_one_fmt_pf formatter);
|
|
int xfs_bulkstat(struct xfs_ibulk *breq, bulkstat_one_fmt_pf formatter);
|
|
void xfs_bulkstat_to_bstat(struct xfs_mount *mp, struct xfs_bstat *bs1,
|
|
const struct xfs_bulkstat *bstat);
|
|
|
|
typedef int (*inumbers_fmt_pf)(struct xfs_ibulk *breq,
|
|
const struct xfs_inumbers *igrp);
|
|
|
|
int xfs_inumbers(struct xfs_ibulk *breq, inumbers_fmt_pf formatter);
|
|
void xfs_inumbers_to_inogrp(struct xfs_inogrp *ig1,
|
|
const struct xfs_inumbers *ig);
|
|
|
|
#endif /* __XFS_ITABLE_H__ */
|