mirror of
https://github.com/torvalds/linux
synced 2024-09-20 02:57:25 +00:00
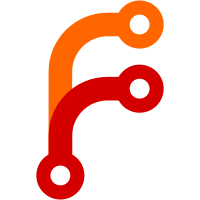
This reverts commit e66485d747
, since
Rafael Wysocki noticed that the change only works for his in -mm, not in
mainline (and that both "noapictimer" _and_ "apicmaintimer" are broken
on his hardware, but that's apparently not a regression, just a symptom
of the same issue that causes the automatic apic timer disable to not
work).
It turns out that it really doesn't work correctly on x86-64, since
x86-64 doesn't use the generic clock events for timers yet.
Thanks to Rafal for testing, and here's the ugly details on x86-64 as
per Thomas:
"I just looked into the code and the logic vs. noapictimer on SMP is
completely broken.
On i386 the noapictimer option not only disables the local APIC
timer, it also registers the CPUs for broadcasting via IPI on SMP
systems.
The x86-64 code uses the broadcast only when the local apic timer is
active, i.e. "noapictimer" is not on the command line. This defeats
the whole purpose of "noapictimer". It should be there to make boxen
work, where the local APIC timer actually has a hardware problem,
e.g. the nx6325.
The current implementation of x86_64 only fixes the ACPI c-states
related problem where the APIC timer stops in C3(2), nothing else.
On nx6325 and other AMD X2 equipped systems which have the C1E
enabled we run into the following:
PIT keeps jiffies (and the system) running, but the local APIC timer
interrupts can get out of sync due to this C1E effect.
I don't think this is a critical problem, but it is wrong
nevertheless.
I think it's safe to revert the C1E patch and postpone the fix to the
clock events conversion."
On further reflection, Thomas noted:
"It's even worse than I thought on the first check:
"noapictimer" on the command line of an SMP box prevents _ONLY_ the
boot CPU apic timer from being used. But the secondary CPU is still
unconditionally setting up the APIC timer and uses the non
calibrated variable calibration_result, which is of course 0, to
setup the APIC timer. Wreckage guaranteed."
so we'll just have to wait for the x86 merge to hopefully fix this up
for x86-64.
Tested-and-requested-by: Rafael J. Wysocki <rjw@sisk.pl>
Acked-by: Thomas Gleixner <tglx@linutronix.de>
Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
108 lines
2.7 KiB
C
108 lines
2.7 KiB
C
#ifndef __ASM_APIC_H
|
|
#define __ASM_APIC_H
|
|
|
|
#include <linux/pm.h>
|
|
#include <linux/delay.h>
|
|
#include <asm/fixmap.h>
|
|
#include <asm/apicdef.h>
|
|
#include <asm/system.h>
|
|
|
|
#define Dprintk(x...)
|
|
|
|
/*
|
|
* Debugging macros
|
|
*/
|
|
#define APIC_QUIET 0
|
|
#define APIC_VERBOSE 1
|
|
#define APIC_DEBUG 2
|
|
|
|
extern int apic_verbosity;
|
|
extern int apic_runs_main_timer;
|
|
extern int ioapic_force;
|
|
extern int apic_mapped;
|
|
|
|
/*
|
|
* Define the default level of output to be very little
|
|
* This can be turned up by using apic=verbose for more
|
|
* information and apic=debug for _lots_ of information.
|
|
* apic_verbosity is defined in apic.c
|
|
*/
|
|
#define apic_printk(v, s, a...) do { \
|
|
if ((v) <= apic_verbosity) \
|
|
printk(s, ##a); \
|
|
} while (0)
|
|
|
|
struct pt_regs;
|
|
|
|
/*
|
|
* Basic functions accessing APICs.
|
|
*/
|
|
|
|
static __inline void apic_write(unsigned long reg, unsigned int v)
|
|
{
|
|
*((volatile unsigned int *)(APIC_BASE+reg)) = v;
|
|
}
|
|
|
|
static __inline unsigned int apic_read(unsigned long reg)
|
|
{
|
|
return *((volatile unsigned int *)(APIC_BASE+reg));
|
|
}
|
|
|
|
extern void apic_wait_icr_idle(void);
|
|
extern unsigned int safe_apic_wait_icr_idle(void);
|
|
|
|
static inline void ack_APIC_irq(void)
|
|
{
|
|
/*
|
|
* ack_APIC_irq() actually gets compiled as a single instruction:
|
|
* - a single rmw on Pentium/82489DX
|
|
* - a single write on P6+ cores (CONFIG_X86_GOOD_APIC)
|
|
* ... yummie.
|
|
*/
|
|
|
|
/* Docs say use 0 for future compatibility */
|
|
apic_write(APIC_EOI, 0);
|
|
}
|
|
|
|
extern int get_maxlvt (void);
|
|
extern void clear_local_APIC (void);
|
|
extern void connect_bsp_APIC (void);
|
|
extern void disconnect_bsp_APIC (int virt_wire_setup);
|
|
extern void disable_local_APIC (void);
|
|
extern int verify_local_APIC (void);
|
|
extern void cache_APIC_registers (void);
|
|
extern void sync_Arb_IDs (void);
|
|
extern void init_bsp_APIC (void);
|
|
extern void setup_local_APIC (void);
|
|
extern void init_apic_mappings (void);
|
|
extern void smp_local_timer_interrupt (void);
|
|
extern void setup_boot_APIC_clock (void);
|
|
extern void setup_secondary_APIC_clock (void);
|
|
extern int APIC_init_uniprocessor (void);
|
|
extern void disable_APIC_timer(void);
|
|
extern void enable_APIC_timer(void);
|
|
extern void setup_apic_routing(void);
|
|
|
|
extern void setup_APIC_extended_lvt(unsigned char lvt_off, unsigned char vector,
|
|
unsigned char msg_type, unsigned char mask);
|
|
|
|
extern int apic_is_clustered_box(void);
|
|
|
|
#define K8_APIC_EXT_LVT_BASE 0x500
|
|
#define K8_APIC_EXT_INT_MSG_FIX 0x0
|
|
#define K8_APIC_EXT_INT_MSG_SMI 0x2
|
|
#define K8_APIC_EXT_INT_MSG_NMI 0x4
|
|
#define K8_APIC_EXT_INT_MSG_EXT 0x7
|
|
#define K8_APIC_EXT_LVT_ENTRY_THRESHOLD 0
|
|
|
|
void smp_send_timer_broadcast_ipi(void);
|
|
void switch_APIC_timer_to_ipi(void *cpumask);
|
|
void switch_ipi_to_APIC_timer(void *cpumask);
|
|
|
|
#define ARCH_APICTIMER_STOPS_ON_C3 1
|
|
|
|
extern unsigned boot_cpu_id;
|
|
extern int local_apic_timer_c2_ok;
|
|
|
|
#endif /* __ASM_APIC_H */
|