mirror of
https://github.com/torvalds/linux
synced 2024-09-21 03:28:37 +00:00
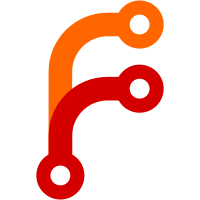
Remove the verbose license text from XFS files and replace them with SPDX tags. This does not change the license of any of the code, merely refers to the common, up-to-date license files in LICENSES/ This change was mostly scripted. fs/xfs/Makefile and fs/xfs/libxfs/xfs_fs.h were modified by hand, the rest were detected and modified by the following command: for f in `git grep -l "GNU General" fs/xfs/` ; do echo $f cat $f | awk -f hdr.awk > $f.new mv -f $f.new $f done And the hdr.awk script that did the modification (including detecting the difference between GPL-2.0 and GPL-2.0+ licenses) is as follows: $ cat hdr.awk BEGIN { hdr = 1.0 tag = "GPL-2.0" str = "" } /^ \* This program is free software/ { hdr = 2.0; next } /any later version./ { tag = "GPL-2.0+" next } /^ \*\// { if (hdr > 0.0) { print "// SPDX-License-Identifier: " tag print str print $0 str="" hdr = 0.0 next } print $0 next } /^ \* / { if (hdr > 1.0) next if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 next } /^ \*/ { if (hdr > 0.0) next print $0 next } // { if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 } END { } $ Signed-off-by: Dave Chinner <dchinner@redhat.com> Reviewed-by: Darrick J. Wong <darrick.wong@oracle.com> Signed-off-by: Darrick J. Wong <darrick.wong@oracle.com>
53 lines
1.6 KiB
C
53 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (C) 2017 Oracle. All Rights Reserved.
|
|
* Author: Darrick J. Wong <darrick.wong@oracle.com>
|
|
*/
|
|
#ifndef __XFS_SCRUB_BTREE_H__
|
|
#define __XFS_SCRUB_BTREE_H__
|
|
|
|
/* btree scrub */
|
|
|
|
/* Check for btree operation errors. */
|
|
bool xfs_scrub_btree_process_error(struct xfs_scrub_context *sc,
|
|
struct xfs_btree_cur *cur, int level, int *error);
|
|
|
|
/* Check for btree xref operation errors. */
|
|
bool xfs_scrub_btree_xref_process_error(struct xfs_scrub_context *sc,
|
|
struct xfs_btree_cur *cur, int level,
|
|
int *error);
|
|
|
|
/* Check for btree corruption. */
|
|
void xfs_scrub_btree_set_corrupt(struct xfs_scrub_context *sc,
|
|
struct xfs_btree_cur *cur, int level);
|
|
|
|
/* Check for btree xref discrepancies. */
|
|
void xfs_scrub_btree_xref_set_corrupt(struct xfs_scrub_context *sc,
|
|
struct xfs_btree_cur *cur, int level);
|
|
|
|
struct xfs_scrub_btree;
|
|
typedef int (*xfs_scrub_btree_rec_fn)(
|
|
struct xfs_scrub_btree *bs,
|
|
union xfs_btree_rec *rec);
|
|
|
|
struct xfs_scrub_btree {
|
|
/* caller-provided scrub state */
|
|
struct xfs_scrub_context *sc;
|
|
struct xfs_btree_cur *cur;
|
|
xfs_scrub_btree_rec_fn scrub_rec;
|
|
struct xfs_owner_info *oinfo;
|
|
void *private;
|
|
|
|
/* internal scrub state */
|
|
union xfs_btree_rec lastrec;
|
|
bool firstrec;
|
|
union xfs_btree_key lastkey[XFS_BTREE_MAXLEVELS];
|
|
bool firstkey[XFS_BTREE_MAXLEVELS];
|
|
struct list_head to_check;
|
|
};
|
|
int xfs_scrub_btree(struct xfs_scrub_context *sc, struct xfs_btree_cur *cur,
|
|
xfs_scrub_btree_rec_fn scrub_fn,
|
|
struct xfs_owner_info *oinfo, void *private);
|
|
|
|
#endif /* __XFS_SCRUB_BTREE_H__ */
|