mirror of
https://github.com/freebsd/freebsd-src
synced 2024-07-22 02:37:15 +00:00
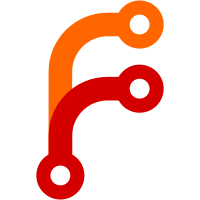
patch to fix half-cycle trigonometric functions Paul Zimmermann, a MPFR developer, contacted me about large errors in the half-cycle trigonometric functions. I've have investigated these issues and developed the attached patch. The float, double, and ld80 (long double) changes have been tested. Caveat emptor: The ld128 changes have not been compiled. The ld128 changes have not been tested. I do not have access to a system that uses ld128 floating point. Here is an itemized list of changes: * lib/msun/src/math_private.h: . Add fast floor macros to compute the integer part of |x| for 0 <= |x| 01xp(N-1), where N is the precision of the type of x. These macros are used in the half-cycle trigonometric functions (e.g., sinpi(x)). . The FFLOOR80 macros is used with the Intel 80-bit extended double functions. This macors corrects an off-by-one error, which led to enormous error for |x| > 0x1p32. * lib/msun/src/s_cospif.c: * lib/msun/src/s_cospi.c: * lib/msun/ld80/s_cospil.c: . Update Copyright years. . Use FFLOOR*() macro to get integer part of |x|. . Correct handle the range 0x1p(N-1) <= |x| < 0x1pN. Here, one needs to determine if the integral value of |x| is even or odd to choose +1 or -1. If |x| >= 0x1pN, always return +1. * lib/msun/src/s_sinpif.c: * lib/msun/src/s_sinpi.c: * lib/msun/ld80/s_sinpil.c: . Update Copyright years. . Use FFLOOR*() macro to get integer part of |x|. * lib/msun/src/s_tanpif.c: * lib/msun/src/s_tanpi.c: * lib/msun/ld80/s_tanpil.c: . Update Copyright years. . For +-0.5, return +-inf. Previously, tanpi[fl]() returned an NaN. . Use FFLOOR*() to get integer part of |x|. Need to determine if the integer part is even or odd. This is used to set +-0 for |x| integral and +-inf for (n+1/2). . For 0x1p(N-1) <= |x| < 0x1pN need to determine if x is an even or odd integer to select +0 or -0. For |x| >= 0x1pN, it is always an even integer, select 0. . Note, tanpi[fl](x) is an odd function, so one needs to consider tanpi[fl](-|x|) = - tanpi[fl](|x|). * lib/msun/ld128/s_cospil.c: * lib/msun/ld128/s_sinpil.c: * lib/msun/ld128/s_tanpil.c: . Update Copyright years. . These routines use an FFLOOR128 macros, which likely should be replaced by a bit twiddling algorithm. . The same considerations above are applied to 0x1p112 <= |x| < 0x1p113, and |x| >= 0x1p113 cases. . Note, even and odd determination used fmodl(x,2.), which is likely slow. PR: 272742 MFC after: 1 week
134 lines
3.6 KiB
C
134 lines
3.6 KiB
C
/*-
|
|
* Copyright (c) 2017, 2023 Steven G. Kargl
|
|
* All rights reserved.
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions
|
|
* are met:
|
|
* 1. Redistributions of source code must retain the above copyright
|
|
* notice unmodified, this list of conditions, and the following
|
|
* disclaimer.
|
|
* 2. Redistributions in binary form must reproduce the above copyright
|
|
* notice, this list of conditions and the following disclaimer in the
|
|
* documentation and/or other materials provided with the distribution.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
|
|
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
|
|
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
|
|
* IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
|
|
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
|
|
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
|
|
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
|
|
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
|
|
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
|
|
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
|
*/
|
|
|
|
/*
|
|
* See ../src/s_tanpi.c for implementation details.
|
|
*/
|
|
|
|
#ifdef __i386__
|
|
#include <ieeefp.h>
|
|
#endif
|
|
#include <stdint.h>
|
|
|
|
#include "fpmath.h"
|
|
#include "math.h"
|
|
#include "math_private.h"
|
|
|
|
static const double
|
|
pi_hi = 3.1415926814079285e+00, /* 0x400921fb 0x58000000 */
|
|
pi_lo = -2.7818135228334233e-08; /* 0xbe5dde97 0x3dcb3b3a */
|
|
|
|
static inline long double
|
|
__kernel_tanpil(long double x)
|
|
{
|
|
long double hi, lo, t;
|
|
|
|
if (x < 0.25) {
|
|
hi = (float)x;
|
|
lo = x - hi;
|
|
lo = lo * (pi_lo + pi_hi) + hi * pi_lo;
|
|
hi *= pi_hi;
|
|
_2sumF(hi, lo);
|
|
t = __kernel_tanl(hi, lo, -1);
|
|
} else if (x > 0.25) {
|
|
x = 0.5 - x;
|
|
hi = (float)x;
|
|
lo = x - hi;
|
|
lo = lo * (pi_lo + pi_hi) + hi * pi_lo;
|
|
hi *= pi_hi;
|
|
_2sumF(hi, lo);
|
|
t = - __kernel_tanl(hi, lo, 1);
|
|
} else
|
|
t = 1;
|
|
|
|
return (t);
|
|
}
|
|
|
|
volatile static const double vzero = 0;
|
|
|
|
long double
|
|
tanpil(long double x)
|
|
{
|
|
long double ax, hi, lo, odd, t;
|
|
uint64_t lx, m;
|
|
uint32_t j0;
|
|
uint16_t hx, ix;
|
|
|
|
EXTRACT_LDBL80_WORDS(hx, lx, x);
|
|
ix = hx & 0x7fff;
|
|
INSERT_LDBL80_WORDS(ax, ix, lx);
|
|
|
|
ENTERI();
|
|
|
|
if (ix < 0x3fff) { /* |x| < 1 */
|
|
if (ix < 0x3ffe) { /* |x| < 0.5 */
|
|
if (ix < 0x3fdd) { /* |x| < 0x1p-34 */
|
|
if (x == 0)
|
|
RETURNI(x);
|
|
INSERT_LDBL80_WORDS(hi, hx,
|
|
lx & 0xffffffff00000000ull);
|
|
hi *= 0x1p63L;
|
|
lo = x * 0x1p63L - hi;
|
|
t = (pi_lo + pi_hi) * lo + pi_lo * hi +
|
|
pi_hi * hi;
|
|
RETURNI(t * 0x1p-63L);
|
|
}
|
|
t = __kernel_tanpil(ax);
|
|
} else if (ax == 0.5)
|
|
t = 1 / vzero;
|
|
else
|
|
t = -__kernel_tanpil(1 - ax);
|
|
RETURNI((hx & 0x8000) ? -t : t);
|
|
}
|
|
|
|
if (ix < 0x403e) { /* 1 <= |x| < 0x1p63 */
|
|
FFLOORL80(x, j0, ix, lx); /* Integer part of ax. */
|
|
odd = (uint64_t)x & 1 ? -1 : 1;
|
|
ax -= x;
|
|
EXTRACT_LDBL80_WORDS(ix, lx, ax);
|
|
|
|
if (ix < 0x3ffe) /* |x| < 0.5 */
|
|
t = ix == 0 ? copysignl(0, odd) : __kernel_tanpil(ax);
|
|
else if (ax == 0.5L)
|
|
t = odd / vzero;
|
|
else
|
|
t = -__kernel_tanpil(1 - ax);
|
|
RETURNI((hx & 0x8000) ? -t : t);
|
|
}
|
|
|
|
/* x = +-inf or nan. */
|
|
if (ix >= 0x7fff)
|
|
RETURNI(vzero / vzero);
|
|
|
|
/*
|
|
* For 0x1p63 <= |x| < 0x1p64 need to determine if x is an even
|
|
* or odd integer to set t = +0 or -0.
|
|
* For |x| >= 0x1p64, it is always an even integer, so t = 0.
|
|
*/
|
|
t = ix >= 0x403f ? 0 : (copysignl(0, (lx & 1) ? -1 : 1));
|
|
RETURNI((hx & 0x8000) ? -t : t);
|
|
}
|