mirror of
https://github.com/freebsd/freebsd-src
synced 2024-07-21 18:27:22 +00:00
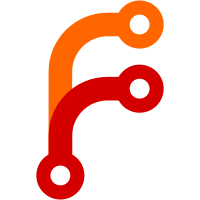
getnetgrent.c: - Catch one bogon that snuck by: in _listmatch(), check for '\0' rather than '\n'; strings returned from yp_match() are terminated with a nul, not a newline. getpwent.c: - Rip out all of the +inclusion/-exclusion stuff from before and replace it with something a little less grotty. The main problem with the old mechanism was that it wasted many cycles processing NIS entries even after it already knew they were to be exlcuded (or not included, depending on your pointof view). The highlights of these changes include: o Uses an in-memory hash database table to keep track of all the -@netgroup, -user, and -@group exclusions. o Tries harder to duplicate the behavior normally obtained when using NIS inclusions/exclusions on a flat /etc/passwd file (meaning things come out in much the same order). o Uses seperate methods for handling getpwent() and getpwnam()/getpwuid() operations instead of trying to do everything with one general function, which didn't work as well as I thought it would. o Uses both getnetgrent() and innetgr() to try to save time where possible. o Use only one special token in the local password database (_PW_KEYYPBYNUM) instead of seperate tokens to mark + and - entries (and stop using the counter tokens too). If this new token doesn't exist, the code will make due with the standard _PW_KEYBYNUM token in order to support older databases that won't have the new token in them. All this is an attempt to make this stuff work better in environments with large NIS passwd databases.
112 lines
4 KiB
C
112 lines
4 KiB
C
/*-
|
|
* Copyright (c) 1989, 1993
|
|
* The Regents of the University of California. All rights reserved.
|
|
* (c) UNIX System Laboratories, Inc.
|
|
* All or some portions of this file are derived from material licensed
|
|
* to the University of California by American Telephone and Telegraph
|
|
* Co. or Unix System Laboratories, Inc. and are reproduced herein with
|
|
* the permission of UNIX System Laboratories, Inc.
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions
|
|
* are met:
|
|
* 1. Redistributions of source code must retain the above copyright
|
|
* notice, this list of conditions and the following disclaimer.
|
|
* 2. Redistributions in binary form must reproduce the above copyright
|
|
* notice, this list of conditions and the following disclaimer in the
|
|
* documentation and/or other materials provided with the distribution.
|
|
* 3. All advertising materials mentioning features or use of this software
|
|
* must display the following acknowledgement:
|
|
* This product includes software developed by the University of
|
|
* California, Berkeley and its contributors.
|
|
* 4. Neither the name of the University nor the names of its contributors
|
|
* may be used to endorse or promote products derived from this software
|
|
* without specific prior written permission.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE REGENTS AND CONTRIBUTORS ``AS IS'' AND
|
|
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
|
|
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
|
|
* ARE DISCLAIMED. IN NO EVENT SHALL THE REGENTS OR CONTRIBUTORS BE LIABLE
|
|
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
|
|
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS
|
|
* OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
|
|
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
|
|
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY
|
|
* OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
|
|
* SUCH DAMAGE.
|
|
*
|
|
* @(#)pwd.h 8.2 (Berkeley) 1/21/94
|
|
*/
|
|
|
|
#ifndef _PWD_H_
|
|
#define _PWD_H_
|
|
|
|
#include <sys/types.h>
|
|
|
|
#ifndef _POSIX_SOURCE
|
|
#define _PATH_PWD "/etc"
|
|
#define _PATH_PASSWD "/etc/passwd"
|
|
#define _PASSWD "passwd"
|
|
#define _PATH_MASTERPASSWD "/etc/master.passwd"
|
|
#define _MASTERPASSWD "master.passwd"
|
|
|
|
#define _PATH_MP_DB "/etc/pwd.db"
|
|
#define _MP_DB "pwd.db"
|
|
#define _PATH_SMP_DB "/etc/spwd.db"
|
|
#define _SMP_DB "spwd.db"
|
|
|
|
#define _PATH_PWD_MKDB "/usr/sbin/pwd_mkdb"
|
|
|
|
#define _PW_KEYBYNAME '1' /* stored by name */
|
|
#define _PW_KEYBYNUM '2' /* stored by entry in the "file" */
|
|
#define _PW_KEYBYUID '3' /* stored by uid */
|
|
#define _PW_KEYYPENABLED '4' /* YP is enabled */
|
|
#define _PW_KEYYPBYNUM '5' /* special +@netgroup entries */
|
|
|
|
#define _PASSWORD_EFMT1 '_' /* extended encryption format */
|
|
|
|
#define _PASSWORD_LEN 128 /* max length, not counting NULL */
|
|
#endif
|
|
|
|
struct passwd {
|
|
char *pw_name; /* user name */
|
|
char *pw_passwd; /* encrypted password */
|
|
int pw_uid; /* user uid */
|
|
int pw_gid; /* user gid */
|
|
time_t pw_change; /* password change time */
|
|
char *pw_class; /* user access class */
|
|
char *pw_gecos; /* Honeywell login info */
|
|
char *pw_dir; /* home directory */
|
|
char *pw_shell; /* default shell */
|
|
time_t pw_expire; /* account expiration */
|
|
int pw_fields; /* internal: fields filled in */
|
|
};
|
|
|
|
/* Mapping from fields to bits for pw_fields. */
|
|
#define _PWF(x) (1 << x)
|
|
#define _PWF_NAME _PWF(0)
|
|
#define _PWF_PASSWD _PWF(1)
|
|
#define _PWF_UID _PWF(2)
|
|
#define _PWF_GID _PWF(3)
|
|
#define _PWF_CHANGE _PWF(4)
|
|
#define _PWF_CLASS _PWF(5)
|
|
#define _PWF_GECOS _PWF(6)
|
|
#define _PWF_DIR _PWF(7)
|
|
#define _PWF_SHELL _PWF(8)
|
|
#define _PWF_EXPIRE _PWF(9)
|
|
|
|
#include <sys/cdefs.h>
|
|
|
|
__BEGIN_DECLS
|
|
struct passwd *getpwuid __P((uid_t));
|
|
struct passwd *getpwnam __P((const char *));
|
|
#ifndef _POSIX_SOURCE
|
|
struct passwd *getpwent __P((void));
|
|
int setpassent __P((int));
|
|
int setpwent __P((void));
|
|
void endpwent __P((void));
|
|
#endif
|
|
__END_DECLS
|
|
|
|
#endif /* !_PWD_H_ */
|