mirror of
https://github.com/freebsd/freebsd-src
synced 2024-07-22 02:37:15 +00:00
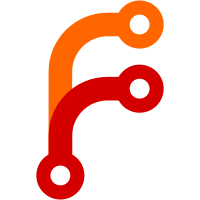
- Add support for queueing and executing NVMe admin and NVM commands via ctl_run and ctl_queue. This requires fixing a few places that were SCSI-specific to add NVME logic. - NVMe has much simpler command ordering requirements than SCSI. In particular, the HBA is not required to enforce any specific ordering for requests with overlapping LBAs. The host is required to manage that ordering. However, fused commands (currently only COMPARE and WRITE NVM commands can be fused) are required to be executed atomically. To support fused commands, make the second half of a fused command block on the first half, and have commands submitted after a fused command pair block on the second half. - Add handlers and command tables for admin and NVM commands that operate on individual namespaces and will be passed down from an NVMe over Fabrics controller to a CTL LUN. Reviewed by: ken, imp Sponsored by: Chelsio Communications Differential Revision: https://reviews.freebsd.org/D44720
36 lines
1.1 KiB
C
36 lines
1.1 KiB
C
/*-
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*
|
|
* Copyright (c) 2023 Chelsio Communications, Inc.
|
|
*/
|
|
|
|
#include <dev/nvme/nvme.h>
|
|
|
|
#include <cam/ctl/ctl.h>
|
|
#include <cam/ctl/ctl_ha.h>
|
|
#include <cam/ctl/ctl_io.h>
|
|
#include <cam/ctl/ctl_ioctl.h>
|
|
#include <cam/ctl/ctl_private.h>
|
|
|
|
/* Administrative Command Set (CTL_IO_NVME_ADMIN). */
|
|
const struct ctl_nvme_cmd_entry nvme_admin_cmd_table[256] =
|
|
{
|
|
[NVME_OPC_IDENTIFY] = { ctl_nvme_identify, CTL_FLAG_DATA_IN |
|
|
CTL_CMD_FLAG_OK_ON_NO_LUN },
|
|
};
|
|
|
|
/* NVM Command Set (CTL_IO_NVME). */
|
|
const struct ctl_nvme_cmd_entry nvme_nvm_cmd_table[256] =
|
|
{
|
|
[NVME_OPC_FLUSH] = { ctl_nvme_flush, CTL_FLAG_DATA_NONE },
|
|
[NVME_OPC_WRITE] = { ctl_nvme_read_write, CTL_FLAG_DATA_OUT },
|
|
[NVME_OPC_READ] = { ctl_nvme_read_write, CTL_FLAG_DATA_IN },
|
|
[NVME_OPC_WRITE_UNCORRECTABLE] = { ctl_nvme_write_uncorrectable,
|
|
CTL_FLAG_DATA_NONE },
|
|
[NVME_OPC_COMPARE] = { ctl_nvme_compare, CTL_FLAG_DATA_OUT },
|
|
[NVME_OPC_WRITE_ZEROES] = { ctl_nvme_write_zeroes, CTL_FLAG_DATA_NONE },
|
|
[NVME_OPC_DATASET_MANAGEMENT] = { ctl_nvme_dataset_management,
|
|
CTL_FLAG_DATA_OUT },
|
|
[NVME_OPC_VERIFY] = { ctl_nvme_verify, CTL_FLAG_DATA_NONE },
|
|
};
|