mirror of
https://gitlab.freedesktop.org/NetworkManager/NetworkManager
synced 2024-07-22 02:35:25 +00:00
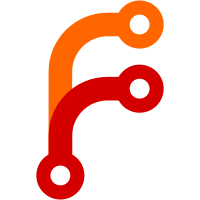
In practice, this should only matter when there are multiple header files with the same name. That is something we try to avoid already, by giving headers a distinct name. When building NetworkManager itself, we clearly want to use double-quotes for including our own headers. But we also want to do that in our public headers. For example: ./a.c #include <stdio.h> #include <nm-1.h> void main() { printf ("INCLUDED %s/nm-2.h\n", SYMB); } ./1/nm-1.h #include <nm-2.h> ./1/nm-2.h #define SYMB "1" ./2/nm-2.h #define SYMB "2" $ cc -I./2 -I./1 ./a.c $ ./a.out INCLUDED 2/nm-2.h Exceptions to this are - headers in "shared/nm-utils" that include <NetworkManager.h>. These headers are copied into projects and hence used like headers owned by those projects. - examples/C
121 lines
5.1 KiB
C
121 lines
5.1 KiB
C
/* -*- Mode: C; tab-width: 4; indent-tabs-mode: t; c-basic-offset: 4 -*- */
|
|
|
|
/*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
* Boston, MA 02110-1301 USA.
|
|
*
|
|
* Copyright 2011 - 2013 Red Hat, Inc.
|
|
*/
|
|
|
|
#ifndef NM_SETTING_BOND_H
|
|
#define NM_SETTING_BOND_H
|
|
|
|
#include "nm-setting.h"
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define NM_TYPE_SETTING_BOND (nm_setting_bond_get_type ())
|
|
#define NM_SETTING_BOND(obj) (G_TYPE_CHECK_INSTANCE_CAST ((obj), NM_TYPE_SETTING_BOND, NMSettingBond))
|
|
#define NM_SETTING_BOND_CLASS(klass) (G_TYPE_CHECK_CLASS_CAST ((klass), NM_TYPE_SETTING_BOND, NMSettingBondClass))
|
|
#define NM_IS_SETTING_BOND(obj) (G_TYPE_CHECK_INSTANCE_TYPE ((obj), NM_TYPE_SETTING_BOND))
|
|
#define NM_IS_SETTING_BOND_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE ((klass), NM_TYPE_SETTING_BOND))
|
|
#define NM_SETTING_BOND_GET_CLASS(obj) (G_TYPE_INSTANCE_GET_CLASS ((obj), NM_TYPE_SETTING_BOND, NMSettingBondClass))
|
|
|
|
#define NM_SETTING_BOND_SETTING_NAME "bond"
|
|
|
|
/**
|
|
* NMSettingBondError:
|
|
* @NM_SETTING_BOND_ERROR_UNKNOWN: unknown or unclassified error
|
|
* @NM_SETTING_BOND_ERROR_INVALID_PROPERTY: the property was invalid
|
|
* @NM_SETTING_BOND_ERROR_MISSING_PROPERTY: the property was missing and is
|
|
* @NM_SETTING_BOND_ERROR_INVALID_OPTION: the option was invalid
|
|
* @NM_SETTING_BOND_ERROR_MISSING_OPTION: the option was missing
|
|
* required
|
|
*/
|
|
typedef enum {
|
|
NM_SETTING_BOND_ERROR_UNKNOWN = 0, /*< nick=UnknownError >*/
|
|
NM_SETTING_BOND_ERROR_INVALID_PROPERTY, /*< nick=InvalidProperty >*/
|
|
NM_SETTING_BOND_ERROR_MISSING_PROPERTY, /*< nick=MissingProperty >*/
|
|
NM_SETTING_BOND_ERROR_INVALID_OPTION, /*< nick=InvalidOption >*/
|
|
NM_SETTING_BOND_ERROR_MISSING_OPTION, /*< nick=MissingOption >*/
|
|
} NMSettingBondError;
|
|
|
|
#define NM_SETTING_BOND_ERROR nm_setting_bond_error_quark ()
|
|
GQuark nm_setting_bond_error_quark (void);
|
|
|
|
#define NM_SETTING_BOND_INTERFACE_NAME "interface-name"
|
|
#define NM_SETTING_BOND_OPTIONS "options"
|
|
|
|
/* Valid options for the 'options' property */
|
|
#define NM_SETTING_BOND_OPTION_MODE "mode"
|
|
#define NM_SETTING_BOND_OPTION_MIIMON "miimon"
|
|
#define NM_SETTING_BOND_OPTION_DOWNDELAY "downdelay"
|
|
#define NM_SETTING_BOND_OPTION_UPDELAY "updelay"
|
|
#define NM_SETTING_BOND_OPTION_ARP_INTERVAL "arp_interval"
|
|
#define NM_SETTING_BOND_OPTION_ARP_IP_TARGET "arp_ip_target"
|
|
#define NM_SETTING_BOND_OPTION_ARP_VALIDATE "arp_validate"
|
|
#define NM_SETTING_BOND_OPTION_PRIMARY "primary"
|
|
#define NM_SETTING_BOND_OPTION_PRIMARY_RESELECT "primary_reselect"
|
|
#define NM_SETTING_BOND_OPTION_FAIL_OVER_MAC "fail_over_mac"
|
|
#define NM_SETTING_BOND_OPTION_USE_CARRIER "use_carrier"
|
|
#define NM_SETTING_BOND_OPTION_AD_SELECT "ad_select"
|
|
#define NM_SETTING_BOND_OPTION_XMIT_HASH_POLICY "xmit_hash_policy"
|
|
#define NM_SETTING_BOND_OPTION_RESEND_IGMP "resend_igmp"
|
|
#define NM_SETTING_BOND_OPTION_LACP_RATE "lacp_rate"
|
|
|
|
typedef struct {
|
|
NMSetting parent;
|
|
} NMSettingBond;
|
|
|
|
typedef struct {
|
|
NMSettingClass parent;
|
|
|
|
/* Padding for future expansion */
|
|
void (*_reserved1) (void);
|
|
void (*_reserved2) (void);
|
|
void (*_reserved3) (void);
|
|
void (*_reserved4) (void);
|
|
} NMSettingBondClass;
|
|
|
|
GType nm_setting_bond_get_type (void);
|
|
|
|
NMSetting * nm_setting_bond_new (void);
|
|
const char * nm_setting_bond_get_interface_name (NMSettingBond *setting);
|
|
guint32 nm_setting_bond_get_num_options (NMSettingBond *setting);
|
|
gboolean nm_setting_bond_get_option (NMSettingBond *setting,
|
|
guint32 idx,
|
|
const char **out_name,
|
|
const char **out_value);
|
|
const char * nm_setting_bond_get_option_by_name (NMSettingBond *setting,
|
|
const char *name);
|
|
gboolean nm_setting_bond_add_option (NMSettingBond *setting,
|
|
const char *name,
|
|
const char *value);
|
|
gboolean nm_setting_bond_remove_option (NMSettingBond *setting,
|
|
const char *name);
|
|
|
|
NM_AVAILABLE_IN_0_9_10
|
|
gboolean nm_setting_bond_validate_option (const char *name,
|
|
const char *value);
|
|
|
|
const char **nm_setting_bond_get_valid_options (NMSettingBond *setting);
|
|
|
|
const char * nm_setting_bond_get_option_default (NMSettingBond *setting,
|
|
const char *name);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* NM_SETTING_BOND_H */
|