mirror of
https://github.com/gravitational/teleport
synced 2024-10-22 02:03:24 +00:00
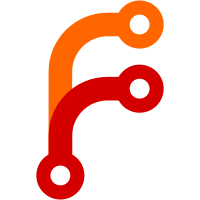
* Remove JSON schema validation Removing JSON schema validation from all resource unmarshalers. --- what JSON schema gets us Looking at the JSON schema spec and our usage, here are the supposed benefits: - type validation - make sure incoming data uses the right types for the right fields - required fields - make sure that mandatory fields are set - defaulting - set defaults for fields - documentation - schema definition for our API objects Note that it does _not_ do: - fail on unknown fields in data - fail on a required field with an empty value --- what replaces it Based on the above, it may seem like JSON schema provides value. But it's not the case, let's break it down one by one: - type validation - unmarshaling JSON into a typed Go struct does this - required fields - only checks that the field was provided, doesn't actually check that a value is set (e.g. `"name": ""` will pass the `required` check) - so it's pretty useless for any real validation - and we already have a separate place for proper validation - `CheckAndSetDefaults` methods - defaulting - done in `CheckAndSetDefaults` methods - `Version` is the only annoying field, had to add it in a bunch of objects - documentation - protobuf definitions are the source of truth for our API schema --- the benefits - performance - schema validation does a few rounds of `json.Marshal/Unmarshal` in addition to actual validation; now we simply skip all that - maintenance - no need to keep protobuf and JSON schema definitions in sync anymore - creating new API objects - one error-prone step removed - (future) fewer dependencies - we can _almost_ remove the Go libraries for schema validation (one transient dependency keeping them around) * Remove services.SkipValidation No more JSON schema validation so this option is a noop.
146 lines
4 KiB
Go
146 lines
4 KiB
Go
/*
|
|
Copyright 2021 Gravitational, Inc.
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
package services
|
|
|
|
import (
|
|
"encoding/json"
|
|
|
|
"github.com/gravitational/teleport/api/types"
|
|
"github.com/gravitational/teleport/lib/utils"
|
|
|
|
"github.com/gravitational/trace"
|
|
)
|
|
|
|
// UnmarshalWebSession unmarshals the WebSession resource from JSON.
|
|
func UnmarshalWebSession(bytes []byte, opts ...MarshalOption) (types.WebSession, error) {
|
|
cfg, err := CollectOptions(opts)
|
|
if err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
|
|
var h ResourceHeader
|
|
err = json.Unmarshal(bytes, &h)
|
|
if err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
switch h.Version {
|
|
case V2:
|
|
var ws types.WebSessionV2
|
|
if err := utils.FastUnmarshal(bytes, &ws); err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
utils.UTC(&ws.Spec.BearerTokenExpires)
|
|
utils.UTC(&ws.Spec.Expires)
|
|
|
|
if err := ws.CheckAndSetDefaults(); err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
if cfg.ID != 0 {
|
|
ws.SetResourceID(cfg.ID)
|
|
}
|
|
if !cfg.Expires.IsZero() {
|
|
ws.SetExpiry(cfg.Expires)
|
|
}
|
|
|
|
return &ws, nil
|
|
}
|
|
|
|
return nil, trace.BadParameter("web session resource version %v is not supported", h.Version)
|
|
}
|
|
|
|
// MarshalWebSession marshals the WebSession resource to JSON.
|
|
func MarshalWebSession(webSession types.WebSession, opts ...MarshalOption) ([]byte, error) {
|
|
cfg, err := CollectOptions(opts)
|
|
if err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
|
|
switch webSession := webSession.(type) {
|
|
case *WebSessionV2:
|
|
if version := webSession.GetVersion(); version != V2 {
|
|
return nil, trace.BadParameter("mismatched web session version %v and type %T", version, webSession)
|
|
}
|
|
if !cfg.PreserveResourceID {
|
|
// avoid modifying the original object
|
|
// to prevent unexpected data races
|
|
copy := *webSession
|
|
copy.SetResourceID(0)
|
|
webSession = ©
|
|
}
|
|
return utils.FastMarshal(webSession)
|
|
default:
|
|
return nil, trace.BadParameter("unrecognized web session version %T", webSession)
|
|
}
|
|
}
|
|
|
|
// MarshalWebToken serializes the web token as JSON-encoded payload
|
|
func MarshalWebToken(webToken types.WebToken, opts ...MarshalOption) ([]byte, error) {
|
|
cfg, err := CollectOptions(opts)
|
|
if err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
|
|
switch webToken := webToken.(type) {
|
|
case *types.WebTokenV3:
|
|
if version := webToken.GetVersion(); version != V3 {
|
|
return nil, trace.BadParameter("mismatched web token version %v and type %T", version, webToken)
|
|
}
|
|
if !cfg.PreserveResourceID {
|
|
// avoid modifying the original object
|
|
// to prevent unexpected data races
|
|
copy := *webToken
|
|
copy.SetResourceID(0)
|
|
webToken = ©
|
|
}
|
|
return utils.FastMarshal(webToken)
|
|
default:
|
|
return nil, trace.BadParameter("unrecognized web token version %T", webToken)
|
|
}
|
|
}
|
|
|
|
// UnmarshalWebToken interprets bytes as JSON-encoded web token value
|
|
func UnmarshalWebToken(bytes []byte, opts ...MarshalOption) (types.WebToken, error) {
|
|
config, err := CollectOptions(opts)
|
|
if err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
var hdr ResourceHeader
|
|
err = json.Unmarshal(bytes, &hdr)
|
|
if err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
switch hdr.Version {
|
|
case V3:
|
|
var token types.WebTokenV3
|
|
if err := utils.FastUnmarshal(bytes, &token); err != nil {
|
|
return nil, trace.BadParameter("invalid web token: %v", err.Error())
|
|
}
|
|
if err := token.CheckAndSetDefaults(); err != nil {
|
|
return nil, trace.Wrap(err)
|
|
}
|
|
if config.ID != 0 {
|
|
token.SetResourceID(config.ID)
|
|
}
|
|
if !config.Expires.IsZero() {
|
|
token.Metadata.SetExpiry(config.Expires)
|
|
}
|
|
utils.UTC(token.Metadata.Expires)
|
|
return &token, nil
|
|
}
|
|
return nil, trace.BadParameter("web token resource version %v is not supported", hdr.Version)
|
|
}
|