mirror of
https://github.com/gravitational/teleport
synced 2024-10-21 17:53:28 +00:00
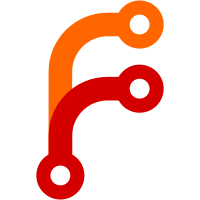
* Start breaking apart bot renewal loops * Refactor initial bot identity fetching * Tidy up some log messages * Add renewal loop for bot identity * Tidy up logging * Add channel broadcaster so multiple can listen * Fix compilation * Ensure template instructions include join-method * Fail harder for template failure to render * More graceful failure to describe identity * support partial renewals of bot identity * Move methods to helper functions to avoid potential state confusion * Simplify how template renderers access the bot * Simplify bot mock in tbot/config tests * Add integration test for whole bot * Further tidying of test * Fix operator sidecar bot invocations * Fix anonymous telemetry testing * Ensure we always return the unlock * Nicer error message * Use better naming for provider/organise impersonated_identity file * Ensure impersonated client closed on failure * Close testclient after initialize complete * Fix tests in main package * Missing license header * Allow join method to be omitted for `tbot init` * Use correct limit in log messages for bot identity renewal * Propagate new identity before persisting it to disk * Move warning about renewal interval * document unsubscribe * Support SIGHUP again
104 lines
2.7 KiB
Go
104 lines
2.7 KiB
Go
/*
|
|
Copyright 2022 Gravitational, Inc.
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
package main
|
|
|
|
import (
|
|
"bytes"
|
|
"os"
|
|
"path/filepath"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"github.com/gravitational/teleport/lib/utils/golden"
|
|
)
|
|
|
|
func TestRun_Configure(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
// This is slightly rubbish, but due to the global nature of `botfs`,
|
|
// it's difficult to configure the default acl and symlink values to be
|
|
// the same across dev laptops and GCB.
|
|
// If we switch to a more dependency injected model for botfs, we can
|
|
// ensure that the test one returns the same value across operating systems.
|
|
normalizeOSDependentValues := func(data []byte) []byte {
|
|
cpy := append([]byte{}, data...)
|
|
cpy = bytes.ReplaceAll(
|
|
cpy, []byte("symlinks: try-secure"), []byte("symlinks: secure"),
|
|
)
|
|
cpy = bytes.ReplaceAll(
|
|
cpy, []byte(`acls: "off"`), []byte("acls: try"),
|
|
)
|
|
return cpy
|
|
}
|
|
|
|
baseArgs := []string{"configure", "--join-method", "token"}
|
|
tests := []struct {
|
|
name string
|
|
args []string
|
|
}{
|
|
{
|
|
name: "no parameters provided",
|
|
args: baseArgs,
|
|
},
|
|
{
|
|
name: "all parameters provided",
|
|
args: append(baseArgs, []string{
|
|
"-a", "example.com",
|
|
"--token", "xxyzz",
|
|
"--ca-pin", "sha256:capindata",
|
|
"--data-dir", "/custom/data/dir",
|
|
"--join-method", "token",
|
|
"--oneshot",
|
|
"--certificate-ttl", "42m",
|
|
"--renewal-interval", "21m",
|
|
"--fips",
|
|
}...),
|
|
},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
tt := tt
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
t.Run("file", func(t *testing.T) {
|
|
path := filepath.Join(t.TempDir(), "config.yaml")
|
|
args := append(tt.args, []string{"-o", path}...)
|
|
err := Run(args, nil)
|
|
require.NoError(t, err)
|
|
|
|
data, err := os.ReadFile(path)
|
|
data = normalizeOSDependentValues(data)
|
|
require.NoError(t, err)
|
|
if golden.ShouldSet() {
|
|
golden.Set(t, data)
|
|
}
|
|
require.Equal(t, string(golden.Get(t)), string(data))
|
|
})
|
|
|
|
t.Run("stdout", func(t *testing.T) {
|
|
stdout := new(bytes.Buffer)
|
|
err := Run(tt.args, stdout)
|
|
require.NoError(t, err)
|
|
data := normalizeOSDependentValues(stdout.Bytes())
|
|
if golden.ShouldSet() {
|
|
golden.Set(t, data)
|
|
}
|
|
require.Equal(t, string(golden.Get(t)), string(data))
|
|
})
|
|
})
|
|
}
|
|
}
|