mirror of
https://github.com/minio/minio
synced 2024-07-21 18:35:07 +00:00
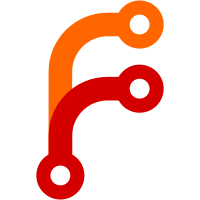
to track the replication transfer rate across different nodes, number of active workers in use and in-queue stats to get an idea of the current workload. This PR also adds replication metrics to the site replication status API. For site replication, prometheus metrics are no longer at the bucket level - but at the cluster level. Add prometheus metric to track credential errors since uptime
689 lines
13 KiB
Go
689 lines
13 KiB
Go
package cmd
|
|
|
|
// Code generated by github.com/tinylib/msgp DO NOT EDIT.
|
|
|
|
import (
|
|
"bytes"
|
|
"testing"
|
|
|
|
"github.com/tinylib/msgp/msgp"
|
|
)
|
|
|
|
func TestMarshalUnmarshalRStat(t *testing.T) {
|
|
v := RStat{}
|
|
bts, err := v.MarshalMsg(nil)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
left, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after UnmarshalMsg(): %q", len(left), left)
|
|
}
|
|
|
|
left, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after Skip(): %q", len(left), left)
|
|
}
|
|
}
|
|
|
|
func BenchmarkMarshalMsgRStat(b *testing.B) {
|
|
v := RStat{}
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.MarshalMsg(nil)
|
|
}
|
|
}
|
|
|
|
func BenchmarkAppendMsgRStat(b *testing.B) {
|
|
v := RStat{}
|
|
bts := make([]byte, 0, v.Msgsize())
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
}
|
|
}
|
|
|
|
func BenchmarkUnmarshalRStat(b *testing.B) {
|
|
v := RStat{}
|
|
bts, _ := v.MarshalMsg(nil)
|
|
b.ReportAllocs()
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
_, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestEncodeDecodeRStat(t *testing.T) {
|
|
v := RStat{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
|
|
m := v.Msgsize()
|
|
if buf.Len() > m {
|
|
t.Log("WARNING: TestEncodeDecodeRStat Msgsize() is inaccurate")
|
|
}
|
|
|
|
vn := RStat{}
|
|
err := msgp.Decode(&buf, &vn)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
buf.Reset()
|
|
msgp.Encode(&buf, &v)
|
|
err = msgp.NewReader(&buf).Skip()
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
}
|
|
|
|
func BenchmarkEncodeRStat(b *testing.B) {
|
|
v := RStat{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
en := msgp.NewWriter(msgp.Nowhere)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.EncodeMsg(en)
|
|
}
|
|
en.Flush()
|
|
}
|
|
|
|
func BenchmarkDecodeRStat(b *testing.B) {
|
|
v := RStat{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
rd := msgp.NewEndlessReader(buf.Bytes(), b)
|
|
dc := msgp.NewReader(rd)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
err := v.DecodeMsg(dc)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestMarshalUnmarshalRTimedMetrics(t *testing.T) {
|
|
v := RTimedMetrics{}
|
|
bts, err := v.MarshalMsg(nil)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
left, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after UnmarshalMsg(): %q", len(left), left)
|
|
}
|
|
|
|
left, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after Skip(): %q", len(left), left)
|
|
}
|
|
}
|
|
|
|
func BenchmarkMarshalMsgRTimedMetrics(b *testing.B) {
|
|
v := RTimedMetrics{}
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.MarshalMsg(nil)
|
|
}
|
|
}
|
|
|
|
func BenchmarkAppendMsgRTimedMetrics(b *testing.B) {
|
|
v := RTimedMetrics{}
|
|
bts := make([]byte, 0, v.Msgsize())
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
}
|
|
}
|
|
|
|
func BenchmarkUnmarshalRTimedMetrics(b *testing.B) {
|
|
v := RTimedMetrics{}
|
|
bts, _ := v.MarshalMsg(nil)
|
|
b.ReportAllocs()
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
_, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestEncodeDecodeRTimedMetrics(t *testing.T) {
|
|
v := RTimedMetrics{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
|
|
m := v.Msgsize()
|
|
if buf.Len() > m {
|
|
t.Log("WARNING: TestEncodeDecodeRTimedMetrics Msgsize() is inaccurate")
|
|
}
|
|
|
|
vn := RTimedMetrics{}
|
|
err := msgp.Decode(&buf, &vn)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
buf.Reset()
|
|
msgp.Encode(&buf, &v)
|
|
err = msgp.NewReader(&buf).Skip()
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
}
|
|
|
|
func BenchmarkEncodeRTimedMetrics(b *testing.B) {
|
|
v := RTimedMetrics{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
en := msgp.NewWriter(msgp.Nowhere)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.EncodeMsg(en)
|
|
}
|
|
en.Flush()
|
|
}
|
|
|
|
func BenchmarkDecodeRTimedMetrics(b *testing.B) {
|
|
v := RTimedMetrics{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
rd := msgp.NewEndlessReader(buf.Bytes(), b)
|
|
dc := msgp.NewReader(rd)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
err := v.DecodeMsg(dc)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestMarshalUnmarshalSRMetric(t *testing.T) {
|
|
v := SRMetric{}
|
|
bts, err := v.MarshalMsg(nil)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
left, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after UnmarshalMsg(): %q", len(left), left)
|
|
}
|
|
|
|
left, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after Skip(): %q", len(left), left)
|
|
}
|
|
}
|
|
|
|
func BenchmarkMarshalMsgSRMetric(b *testing.B) {
|
|
v := SRMetric{}
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.MarshalMsg(nil)
|
|
}
|
|
}
|
|
|
|
func BenchmarkAppendMsgSRMetric(b *testing.B) {
|
|
v := SRMetric{}
|
|
bts := make([]byte, 0, v.Msgsize())
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
}
|
|
}
|
|
|
|
func BenchmarkUnmarshalSRMetric(b *testing.B) {
|
|
v := SRMetric{}
|
|
bts, _ := v.MarshalMsg(nil)
|
|
b.ReportAllocs()
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
_, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestEncodeDecodeSRMetric(t *testing.T) {
|
|
v := SRMetric{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
|
|
m := v.Msgsize()
|
|
if buf.Len() > m {
|
|
t.Log("WARNING: TestEncodeDecodeSRMetric Msgsize() is inaccurate")
|
|
}
|
|
|
|
vn := SRMetric{}
|
|
err := msgp.Decode(&buf, &vn)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
buf.Reset()
|
|
msgp.Encode(&buf, &v)
|
|
err = msgp.NewReader(&buf).Skip()
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
}
|
|
|
|
func BenchmarkEncodeSRMetric(b *testing.B) {
|
|
v := SRMetric{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
en := msgp.NewWriter(msgp.Nowhere)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.EncodeMsg(en)
|
|
}
|
|
en.Flush()
|
|
}
|
|
|
|
func BenchmarkDecodeSRMetric(b *testing.B) {
|
|
v := SRMetric{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
rd := msgp.NewEndlessReader(buf.Bytes(), b)
|
|
dc := msgp.NewReader(rd)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
err := v.DecodeMsg(dc)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestMarshalUnmarshalSRMetricsSummary(t *testing.T) {
|
|
v := SRMetricsSummary{}
|
|
bts, err := v.MarshalMsg(nil)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
left, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after UnmarshalMsg(): %q", len(left), left)
|
|
}
|
|
|
|
left, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after Skip(): %q", len(left), left)
|
|
}
|
|
}
|
|
|
|
func BenchmarkMarshalMsgSRMetricsSummary(b *testing.B) {
|
|
v := SRMetricsSummary{}
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.MarshalMsg(nil)
|
|
}
|
|
}
|
|
|
|
func BenchmarkAppendMsgSRMetricsSummary(b *testing.B) {
|
|
v := SRMetricsSummary{}
|
|
bts := make([]byte, 0, v.Msgsize())
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
}
|
|
}
|
|
|
|
func BenchmarkUnmarshalSRMetricsSummary(b *testing.B) {
|
|
v := SRMetricsSummary{}
|
|
bts, _ := v.MarshalMsg(nil)
|
|
b.ReportAllocs()
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
_, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestEncodeDecodeSRMetricsSummary(t *testing.T) {
|
|
v := SRMetricsSummary{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
|
|
m := v.Msgsize()
|
|
if buf.Len() > m {
|
|
t.Log("WARNING: TestEncodeDecodeSRMetricsSummary Msgsize() is inaccurate")
|
|
}
|
|
|
|
vn := SRMetricsSummary{}
|
|
err := msgp.Decode(&buf, &vn)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
buf.Reset()
|
|
msgp.Encode(&buf, &v)
|
|
err = msgp.NewReader(&buf).Skip()
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
}
|
|
|
|
func BenchmarkEncodeSRMetricsSummary(b *testing.B) {
|
|
v := SRMetricsSummary{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
en := msgp.NewWriter(msgp.Nowhere)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.EncodeMsg(en)
|
|
}
|
|
en.Flush()
|
|
}
|
|
|
|
func BenchmarkDecodeSRMetricsSummary(b *testing.B) {
|
|
v := SRMetricsSummary{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
rd := msgp.NewEndlessReader(buf.Bytes(), b)
|
|
dc := msgp.NewReader(rd)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
err := v.DecodeMsg(dc)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestMarshalUnmarshalSRStats(t *testing.T) {
|
|
v := SRStats{}
|
|
bts, err := v.MarshalMsg(nil)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
left, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after UnmarshalMsg(): %q", len(left), left)
|
|
}
|
|
|
|
left, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after Skip(): %q", len(left), left)
|
|
}
|
|
}
|
|
|
|
func BenchmarkMarshalMsgSRStats(b *testing.B) {
|
|
v := SRStats{}
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.MarshalMsg(nil)
|
|
}
|
|
}
|
|
|
|
func BenchmarkAppendMsgSRStats(b *testing.B) {
|
|
v := SRStats{}
|
|
bts := make([]byte, 0, v.Msgsize())
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
}
|
|
}
|
|
|
|
func BenchmarkUnmarshalSRStats(b *testing.B) {
|
|
v := SRStats{}
|
|
bts, _ := v.MarshalMsg(nil)
|
|
b.ReportAllocs()
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
_, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestEncodeDecodeSRStats(t *testing.T) {
|
|
v := SRStats{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
|
|
m := v.Msgsize()
|
|
if buf.Len() > m {
|
|
t.Log("WARNING: TestEncodeDecodeSRStats Msgsize() is inaccurate")
|
|
}
|
|
|
|
vn := SRStats{}
|
|
err := msgp.Decode(&buf, &vn)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
buf.Reset()
|
|
msgp.Encode(&buf, &v)
|
|
err = msgp.NewReader(&buf).Skip()
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
}
|
|
|
|
func BenchmarkEncodeSRStats(b *testing.B) {
|
|
v := SRStats{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
en := msgp.NewWriter(msgp.Nowhere)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.EncodeMsg(en)
|
|
}
|
|
en.Flush()
|
|
}
|
|
|
|
func BenchmarkDecodeSRStats(b *testing.B) {
|
|
v := SRStats{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
rd := msgp.NewEndlessReader(buf.Bytes(), b)
|
|
dc := msgp.NewReader(rd)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
err := v.DecodeMsg(dc)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestMarshalUnmarshalSRStatus(t *testing.T) {
|
|
v := SRStatus{}
|
|
bts, err := v.MarshalMsg(nil)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
left, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after UnmarshalMsg(): %q", len(left), left)
|
|
}
|
|
|
|
left, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(left) > 0 {
|
|
t.Errorf("%d bytes left over after Skip(): %q", len(left), left)
|
|
}
|
|
}
|
|
|
|
func BenchmarkMarshalMsgSRStatus(b *testing.B) {
|
|
v := SRStatus{}
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.MarshalMsg(nil)
|
|
}
|
|
}
|
|
|
|
func BenchmarkAppendMsgSRStatus(b *testing.B) {
|
|
v := SRStatus{}
|
|
bts := make([]byte, 0, v.Msgsize())
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
bts, _ = v.MarshalMsg(bts[0:0])
|
|
}
|
|
}
|
|
|
|
func BenchmarkUnmarshalSRStatus(b *testing.B) {
|
|
v := SRStatus{}
|
|
bts, _ := v.MarshalMsg(nil)
|
|
b.ReportAllocs()
|
|
b.SetBytes(int64(len(bts)))
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
_, err := v.UnmarshalMsg(bts)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestEncodeDecodeSRStatus(t *testing.T) {
|
|
v := SRStatus{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
|
|
m := v.Msgsize()
|
|
if buf.Len() > m {
|
|
t.Log("WARNING: TestEncodeDecodeSRStatus Msgsize() is inaccurate")
|
|
}
|
|
|
|
vn := SRStatus{}
|
|
err := msgp.Decode(&buf, &vn)
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
|
|
buf.Reset()
|
|
msgp.Encode(&buf, &v)
|
|
err = msgp.NewReader(&buf).Skip()
|
|
if err != nil {
|
|
t.Error(err)
|
|
}
|
|
}
|
|
|
|
func BenchmarkEncodeSRStatus(b *testing.B) {
|
|
v := SRStatus{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
en := msgp.NewWriter(msgp.Nowhere)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
v.EncodeMsg(en)
|
|
}
|
|
en.Flush()
|
|
}
|
|
|
|
func BenchmarkDecodeSRStatus(b *testing.B) {
|
|
v := SRStatus{}
|
|
var buf bytes.Buffer
|
|
msgp.Encode(&buf, &v)
|
|
b.SetBytes(int64(buf.Len()))
|
|
rd := msgp.NewEndlessReader(buf.Bytes(), b)
|
|
dc := msgp.NewReader(rd)
|
|
b.ReportAllocs()
|
|
b.ResetTimer()
|
|
for i := 0; i < b.N; i++ {
|
|
err := v.DecodeMsg(dc)
|
|
if err != nil {
|
|
b.Fatal(err)
|
|
}
|
|
}
|
|
}
|