mirror of
https://github.com/minio/minio
synced 2024-09-29 20:54:33 +00:00
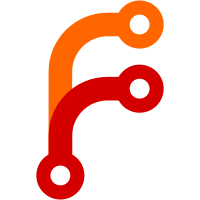
Use Walk(), which is a recursive listing with versioning, to check if the bucket has some objects before being removed. This is beneficial because the bucket can contain multiple dangling objects in multiple drives. Also, this will prevent a bug where a bucket is deleted in a deployment that has many erasure sets but the bucket contains one or few objects not spread to enough erasure sets.
338 lines
8.7 KiB
Go
338 lines
8.7 KiB
Go
package cmd
|
|
|
|
// Code generated by github.com/tinylib/msgp DO NOT EDIT.
|
|
|
|
import (
|
|
"github.com/tinylib/msgp/msgp"
|
|
)
|
|
|
|
// MarshalMsg implements msgp.Marshaler
|
|
func (z BucketOptions) MarshalMsg(b []byte) (o []byte, err error) {
|
|
o = msgp.Require(b, z.Msgsize())
|
|
// map header, size 3
|
|
// string "Deleted"
|
|
o = append(o, 0x83, 0xa7, 0x44, 0x65, 0x6c, 0x65, 0x74, 0x65, 0x64)
|
|
o = msgp.AppendBool(o, z.Deleted)
|
|
// string "Cached"
|
|
o = append(o, 0xa6, 0x43, 0x61, 0x63, 0x68, 0x65, 0x64)
|
|
o = msgp.AppendBool(o, z.Cached)
|
|
// string "NoMetadata"
|
|
o = append(o, 0xaa, 0x4e, 0x6f, 0x4d, 0x65, 0x74, 0x61, 0x64, 0x61, 0x74, 0x61)
|
|
o = msgp.AppendBool(o, z.NoMetadata)
|
|
return
|
|
}
|
|
|
|
// UnmarshalMsg implements msgp.Unmarshaler
|
|
func (z *BucketOptions) UnmarshalMsg(bts []byte) (o []byte, err error) {
|
|
var field []byte
|
|
_ = field
|
|
var zb0001 uint32
|
|
zb0001, bts, err = msgp.ReadMapHeaderBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
for zb0001 > 0 {
|
|
zb0001--
|
|
field, bts, err = msgp.ReadMapKeyZC(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
switch msgp.UnsafeString(field) {
|
|
case "Deleted":
|
|
z.Deleted, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "Deleted")
|
|
return
|
|
}
|
|
case "Cached":
|
|
z.Cached, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "Cached")
|
|
return
|
|
}
|
|
case "NoMetadata":
|
|
z.NoMetadata, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "NoMetadata")
|
|
return
|
|
}
|
|
default:
|
|
bts, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
}
|
|
}
|
|
o = bts
|
|
return
|
|
}
|
|
|
|
// Msgsize returns an upper bound estimate of the number of bytes occupied by the serialized message
|
|
func (z BucketOptions) Msgsize() (s int) {
|
|
s = 1 + 8 + msgp.BoolSize + 7 + msgp.BoolSize + 11 + msgp.BoolSize
|
|
return
|
|
}
|
|
|
|
// MarshalMsg implements msgp.Marshaler
|
|
func (z ExpirationOptions) MarshalMsg(b []byte) (o []byte, err error) {
|
|
o = msgp.Require(b, z.Msgsize())
|
|
// map header, size 1
|
|
// string "Expire"
|
|
o = append(o, 0x81, 0xa6, 0x45, 0x78, 0x70, 0x69, 0x72, 0x65)
|
|
o = msgp.AppendBool(o, z.Expire)
|
|
return
|
|
}
|
|
|
|
// UnmarshalMsg implements msgp.Unmarshaler
|
|
func (z *ExpirationOptions) UnmarshalMsg(bts []byte) (o []byte, err error) {
|
|
var field []byte
|
|
_ = field
|
|
var zb0001 uint32
|
|
zb0001, bts, err = msgp.ReadMapHeaderBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
for zb0001 > 0 {
|
|
zb0001--
|
|
field, bts, err = msgp.ReadMapKeyZC(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
switch msgp.UnsafeString(field) {
|
|
case "Expire":
|
|
z.Expire, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "Expire")
|
|
return
|
|
}
|
|
default:
|
|
bts, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
}
|
|
}
|
|
o = bts
|
|
return
|
|
}
|
|
|
|
// Msgsize returns an upper bound estimate of the number of bytes occupied by the serialized message
|
|
func (z ExpirationOptions) Msgsize() (s int) {
|
|
s = 1 + 7 + msgp.BoolSize
|
|
return
|
|
}
|
|
|
|
// MarshalMsg implements msgp.Marshaler
|
|
func (z *MakeBucketOptions) MarshalMsg(b []byte) (o []byte, err error) {
|
|
o = msgp.Require(b, z.Msgsize())
|
|
// map header, size 5
|
|
// string "LockEnabled"
|
|
o = append(o, 0x85, 0xab, 0x4c, 0x6f, 0x63, 0x6b, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, 0x64)
|
|
o = msgp.AppendBool(o, z.LockEnabled)
|
|
// string "VersioningEnabled"
|
|
o = append(o, 0xb1, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x69, 0x6e, 0x67, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, 0x64)
|
|
o = msgp.AppendBool(o, z.VersioningEnabled)
|
|
// string "ForceCreate"
|
|
o = append(o, 0xab, 0x46, 0x6f, 0x72, 0x63, 0x65, 0x43, 0x72, 0x65, 0x61, 0x74, 0x65)
|
|
o = msgp.AppendBool(o, z.ForceCreate)
|
|
// string "CreatedAt"
|
|
o = append(o, 0xa9, 0x43, 0x72, 0x65, 0x61, 0x74, 0x65, 0x64, 0x41, 0x74)
|
|
o = msgp.AppendTime(o, z.CreatedAt)
|
|
// string "NoLock"
|
|
o = append(o, 0xa6, 0x4e, 0x6f, 0x4c, 0x6f, 0x63, 0x6b)
|
|
o = msgp.AppendBool(o, z.NoLock)
|
|
return
|
|
}
|
|
|
|
// UnmarshalMsg implements msgp.Unmarshaler
|
|
func (z *MakeBucketOptions) UnmarshalMsg(bts []byte) (o []byte, err error) {
|
|
var field []byte
|
|
_ = field
|
|
var zb0001 uint32
|
|
zb0001, bts, err = msgp.ReadMapHeaderBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
for zb0001 > 0 {
|
|
zb0001--
|
|
field, bts, err = msgp.ReadMapKeyZC(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
switch msgp.UnsafeString(field) {
|
|
case "LockEnabled":
|
|
z.LockEnabled, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "LockEnabled")
|
|
return
|
|
}
|
|
case "VersioningEnabled":
|
|
z.VersioningEnabled, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "VersioningEnabled")
|
|
return
|
|
}
|
|
case "ForceCreate":
|
|
z.ForceCreate, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "ForceCreate")
|
|
return
|
|
}
|
|
case "CreatedAt":
|
|
z.CreatedAt, bts, err = msgp.ReadTimeBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "CreatedAt")
|
|
return
|
|
}
|
|
case "NoLock":
|
|
z.NoLock, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "NoLock")
|
|
return
|
|
}
|
|
default:
|
|
bts, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
}
|
|
}
|
|
o = bts
|
|
return
|
|
}
|
|
|
|
// Msgsize returns an upper bound estimate of the number of bytes occupied by the serialized message
|
|
func (z *MakeBucketOptions) Msgsize() (s int) {
|
|
s = 1 + 12 + msgp.BoolSize + 18 + msgp.BoolSize + 12 + msgp.BoolSize + 10 + msgp.TimeSize + 7 + msgp.BoolSize
|
|
return
|
|
}
|
|
|
|
// MarshalMsg implements msgp.Marshaler
|
|
func (z *WalkOptions) MarshalMsg(b []byte) (o []byte, err error) {
|
|
o = msgp.Require(b, z.Msgsize())
|
|
// map header, size 5
|
|
// string "Marker"
|
|
o = append(o, 0x85, 0xa6, 0x4d, 0x61, 0x72, 0x6b, 0x65, 0x72)
|
|
o = msgp.AppendString(o, z.Marker)
|
|
// string "LatestOnly"
|
|
o = append(o, 0xaa, 0x4c, 0x61, 0x74, 0x65, 0x73, 0x74, 0x4f, 0x6e, 0x6c, 0x79)
|
|
o = msgp.AppendBool(o, z.LatestOnly)
|
|
// string "AskDisks"
|
|
o = append(o, 0xa8, 0x41, 0x73, 0x6b, 0x44, 0x69, 0x73, 0x6b, 0x73)
|
|
o = msgp.AppendString(o, z.AskDisks)
|
|
// string "VersionsSort"
|
|
o = append(o, 0xac, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x53, 0x6f, 0x72, 0x74)
|
|
o = msgp.AppendUint8(o, uint8(z.VersionsSort))
|
|
// string "Limit"
|
|
o = append(o, 0xa5, 0x4c, 0x69, 0x6d, 0x69, 0x74)
|
|
o = msgp.AppendInt(o, z.Limit)
|
|
return
|
|
}
|
|
|
|
// UnmarshalMsg implements msgp.Unmarshaler
|
|
func (z *WalkOptions) UnmarshalMsg(bts []byte) (o []byte, err error) {
|
|
var field []byte
|
|
_ = field
|
|
var zb0001 uint32
|
|
zb0001, bts, err = msgp.ReadMapHeaderBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
for zb0001 > 0 {
|
|
zb0001--
|
|
field, bts, err = msgp.ReadMapKeyZC(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
switch msgp.UnsafeString(field) {
|
|
case "Marker":
|
|
z.Marker, bts, err = msgp.ReadStringBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "Marker")
|
|
return
|
|
}
|
|
case "LatestOnly":
|
|
z.LatestOnly, bts, err = msgp.ReadBoolBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "LatestOnly")
|
|
return
|
|
}
|
|
case "AskDisks":
|
|
z.AskDisks, bts, err = msgp.ReadStringBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "AskDisks")
|
|
return
|
|
}
|
|
case "VersionsSort":
|
|
{
|
|
var zb0002 uint8
|
|
zb0002, bts, err = msgp.ReadUint8Bytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "VersionsSort")
|
|
return
|
|
}
|
|
z.VersionsSort = WalkVersionsSortOrder(zb0002)
|
|
}
|
|
case "Limit":
|
|
z.Limit, bts, err = msgp.ReadIntBytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err, "Limit")
|
|
return
|
|
}
|
|
default:
|
|
bts, err = msgp.Skip(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
}
|
|
}
|
|
o = bts
|
|
return
|
|
}
|
|
|
|
// Msgsize returns an upper bound estimate of the number of bytes occupied by the serialized message
|
|
func (z *WalkOptions) Msgsize() (s int) {
|
|
s = 1 + 7 + msgp.StringPrefixSize + len(z.Marker) + 11 + msgp.BoolSize + 9 + msgp.StringPrefixSize + len(z.AskDisks) + 13 + msgp.Uint8Size + 6 + msgp.IntSize
|
|
return
|
|
}
|
|
|
|
// MarshalMsg implements msgp.Marshaler
|
|
func (z WalkVersionsSortOrder) MarshalMsg(b []byte) (o []byte, err error) {
|
|
o = msgp.Require(b, z.Msgsize())
|
|
o = msgp.AppendUint8(o, uint8(z))
|
|
return
|
|
}
|
|
|
|
// UnmarshalMsg implements msgp.Unmarshaler
|
|
func (z *WalkVersionsSortOrder) UnmarshalMsg(bts []byte) (o []byte, err error) {
|
|
{
|
|
var zb0001 uint8
|
|
zb0001, bts, err = msgp.ReadUint8Bytes(bts)
|
|
if err != nil {
|
|
err = msgp.WrapError(err)
|
|
return
|
|
}
|
|
(*z) = WalkVersionsSortOrder(zb0001)
|
|
}
|
|
o = bts
|
|
return
|
|
}
|
|
|
|
// Msgsize returns an upper bound estimate of the number of bytes occupied by the serialized message
|
|
func (z WalkVersionsSortOrder) Msgsize() (s int) {
|
|
s = msgp.Uint8Size
|
|
return
|
|
}
|