mirror of
https://invent.kde.org/graphics/okular
synced 2024-08-25 02:45:38 +00:00
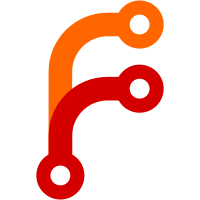
PixmapRequest class. When requesting pixmaps, one or multiple requests are sent to the Document that (frees memory as in current policy) and send each PixmapRequest to the current Generator. Added a signal in generators to notify the Document when a pixmap generation has finished. PageView, ThumbnailsList, PreviewWidget have been unbroken after the memory management commit. (mem management seems in pretty good shape..it's smart.) Added 'visible widgets' list to those classes to speed up searching and processing on visible widgets only. Note: asyncronous pixmap requests can now be queued and we're getting very close to the threaded generator. Note2: Leakfixes and memory improvements. Final NOTE: head merging is possible now, as all remaining work can be considered bugfixes.. API is getting final. It will only change in xpdf dep stuff, the already undefined Viewport object and some bits in Generators. svn path=/branches/kpdf_experiments/kdegraphics/kpdf/; revision=372787
90 lines
2.9 KiB
C++
90 lines
2.9 KiB
C++
/***************************************************************************
|
|
* Copyright (C) 2004 by Enrico Ros <eros.kde@email.it> *
|
|
* *
|
|
* This program is free software; you can redistribute it and/or modify *
|
|
* it under the terms of the GNU General Public License as published by *
|
|
* the Free Software Foundation; either version 2 of the License, or *
|
|
* (at your option) any later version. *
|
|
***************************************************************************/
|
|
|
|
#ifndef _KPDF_PRESENTATIONWIDGET_H_
|
|
#define _KPDF_PRESENTATIONWIDGET_H_
|
|
|
|
#include <qwidget.h>
|
|
#include <qpixmap.h>
|
|
#include <qstringlist.h>
|
|
#include <qvaluevector.h>
|
|
#include "document.h"
|
|
|
|
class PresentationFrame;
|
|
class KToolBar;
|
|
class QTimer;
|
|
|
|
/**
|
|
* @short A widget that shows pages as fullscreen slides (with transitions fx).
|
|
*
|
|
* This is a fullscreen widget that displays
|
|
*/
|
|
class PresentationWidget : public QWidget, public KPDFDocumentObserver
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
PresentationWidget( KPDFDocument * doc );
|
|
~PresentationWidget();
|
|
|
|
// define type of transitions used by presentation frames
|
|
enum TransitionType { NoTrans, BoxIn, BoxOut, Glitter, GlitterDir,
|
|
FuseDir, SplitDir, WipeDir };
|
|
enum TransitionDirection { Left, Top, Right, Bottom };
|
|
|
|
// inherited from KPDFDocumentObserver
|
|
uint observerId() const { return PRESENTATION_ID; }
|
|
void pageSetup( const QValueVector<KPDFPage*> & pages, bool documentChanged );
|
|
bool canUnloadPixmap( int pageNumber );
|
|
void notifyPixmapChanged( int pageNumber );
|
|
|
|
protected:
|
|
// widget events
|
|
void keyPressEvent( QKeyEvent * e );
|
|
void wheelEvent( QWheelEvent * e );
|
|
void mousePressEvent( QMouseEvent * e );
|
|
void mouseMoveEvent( QMouseEvent * e );
|
|
void paintEvent( QPaintEvent * e );
|
|
|
|
private:
|
|
void generatePage();
|
|
void generateIntroPage( QPainter & p );
|
|
void generateContentsPage( int page, QPainter & p );
|
|
void generateOverlay();
|
|
void initTransition( TransitionType type );
|
|
|
|
// cache stuff
|
|
int m_width;
|
|
int m_height;
|
|
QPixmap m_lastRenderedPixmap;
|
|
QPixmap m_lastRenderedOverlay;
|
|
QRect m_overlayGeometry;
|
|
|
|
// transition related
|
|
QTimer * m_transitionTimer;
|
|
QTimer * m_overlayHideTimer;
|
|
int m_transitionDelay;
|
|
int m_transitionMul;
|
|
QValueList< QRect > m_transitionRects;
|
|
|
|
// misc stuff
|
|
KPDFDocument * m_document;
|
|
QValueVector< PresentationFrame * > m_frames;
|
|
int m_frameIndex;
|
|
QStringList m_metaStrings;
|
|
KToolBar * m_topBar;
|
|
|
|
private slots:
|
|
void slotNextPage();
|
|
void slotPrevPage();
|
|
void slotHideOverlay();
|
|
void slotTransitionStep();
|
|
};
|
|
|
|
#endif
|