mirror of
https://invent.kde.org/graphics/okular
synced 2024-10-28 19:28:38 +00:00
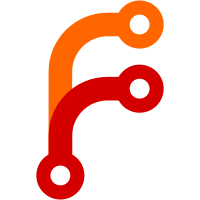
- 0..n-1 are the page indexes again - -1 is for the fonts not reall belonging to a single page (or when no selective page extraction can be done) svn path=/trunk/KDE/kdegraphics/okular/; revision=753866
128 lines
2.7 KiB
C++
128 lines
2.7 KiB
C++
/***************************************************************************
|
|
* Copyright (C) 2007 Tobias Koenig <tokoe@kde.org> *
|
|
* *
|
|
* This program is free software; you can redistribute it and/or modify *
|
|
* it under the terms of the GNU General Public License as published by *
|
|
* the Free Software Foundation; either version 2 of the License, or *
|
|
* (at your option) any later version. *
|
|
***************************************************************************/
|
|
|
|
#include "generator_p.h"
|
|
|
|
#include <kdebug.h>
|
|
|
|
#include "fontinfo.h"
|
|
#include "generator.h"
|
|
|
|
using namespace Okular;
|
|
|
|
PixmapGenerationThread::PixmapGenerationThread( Generator *generator )
|
|
: mGenerator( generator ), mRequest( 0 )
|
|
{
|
|
}
|
|
|
|
void PixmapGenerationThread::startGeneration( PixmapRequest *request )
|
|
{
|
|
mRequest = request;
|
|
|
|
start( QThread::InheritPriority );
|
|
}
|
|
|
|
void PixmapGenerationThread::endGeneration()
|
|
{
|
|
mRequest = 0;
|
|
}
|
|
|
|
PixmapRequest *PixmapGenerationThread::request() const
|
|
{
|
|
return mRequest;
|
|
}
|
|
|
|
QImage PixmapGenerationThread::image() const
|
|
{
|
|
return mImage;
|
|
}
|
|
|
|
void PixmapGenerationThread::run()
|
|
{
|
|
mImage = QImage();
|
|
|
|
if ( mRequest )
|
|
mImage = mGenerator->image( mRequest );
|
|
}
|
|
|
|
|
|
TextPageGenerationThread::TextPageGenerationThread( Generator *generator )
|
|
: mGenerator( generator ), mPage( 0 )
|
|
{
|
|
}
|
|
|
|
void TextPageGenerationThread::startGeneration( Page *page )
|
|
{
|
|
mPage = page;
|
|
|
|
start( QThread::InheritPriority );
|
|
}
|
|
|
|
void TextPageGenerationThread::endGeneration()
|
|
{
|
|
mPage = 0;
|
|
}
|
|
|
|
Page *TextPageGenerationThread::page() const
|
|
{
|
|
return mPage;
|
|
}
|
|
|
|
TextPage* TextPageGenerationThread::textPage() const
|
|
{
|
|
return mTextPage;
|
|
}
|
|
|
|
void TextPageGenerationThread::run()
|
|
{
|
|
mTextPage = 0;
|
|
|
|
if ( mPage )
|
|
mTextPage = mGenerator->textPage( mPage );
|
|
}
|
|
|
|
|
|
FontExtractionThread::FontExtractionThread( Generator *generator, int pages )
|
|
: mGenerator( generator ), mNumOfPages( pages ), mGoOn( true )
|
|
{
|
|
}
|
|
|
|
void FontExtractionThread::startExtraction( bool async )
|
|
{
|
|
if ( async )
|
|
{
|
|
connect( this, SIGNAL( finished() ), this, SLOT( deleteLater() ) );
|
|
start( QThread::InheritPriority );
|
|
}
|
|
else
|
|
{
|
|
run();
|
|
deleteLater();
|
|
}
|
|
}
|
|
|
|
void FontExtractionThread::stopExtraction()
|
|
{
|
|
mGoOn = false;
|
|
}
|
|
|
|
void FontExtractionThread::run()
|
|
{
|
|
for ( int i = -1; i < mNumOfPages && mGoOn; ++i )
|
|
{
|
|
FontInfo::List list = mGenerator->fontsForPage( i );
|
|
foreach ( const FontInfo& fi, list )
|
|
{
|
|
emit gotFont( fi );
|
|
}
|
|
emit progress( i );
|
|
}
|
|
}
|
|
|
|
#include "generator_p.moc"
|