mirror of
https://invent.kde.org/graphics/okular
synced 2024-10-01 05:33:33 +00:00
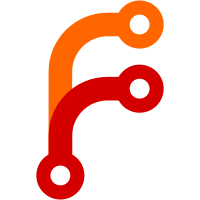
Previously the `<del>` tag that Discount emits would be removed by Qt
since it's not in the subset of HTML that it understands. It does know
about `<s>` though, so replace `<del>` with `<s>` to render strikethroughs
correctly.
Note that I couldn't get Okular to build locally (PEBCAK issue) so I split out this file to debug it. I've tested against http://daringfireball.net/projects/downloads/MarkdownTest_1.0.zip and ff866f73d4/tests/data
and the only difference is that trailing whitespace is now trimmed from the end of a line:
```diff
8c8
< <p style=" margin-top:12px; margin-bottom:12px; margin-left:0px; margin-right:0px; -qt-block-indent:0; text-indent:0px;">Dashes:</p>
---
> <p style=" margin-top:12px; margin-bottom:12px; margin-left:0px; margin-right:0px; -qt-block-indent:0; text-indent:0px;">Dashes: </p>
```
A quick search suggests this isn't a problem though https://stackoverflow.com/a/27026403:
> Within block tags (i.e. `<p>`, `<h1>`, `<div>`, ...) spaces as well as line breaks at the beginning or end of the tags should always be ignored (i.e. `<p>test</p>` should look the same as `<p> test </p>`).
I also tried https://doc.qt.io/qt-5/qtextdocument.html#setMarkdown which solves the strikethrough problem, though there are many visible differences with that approach. Most notably, everything is squashed together and code blocks lose their formatting.
63 lines
1.5 KiB
C++
63 lines
1.5 KiB
C++
/*
|
|
SPDX-FileCopyrightText: 2017 Julian Wolff <wolff@julianwolff.de>
|
|
|
|
SPDX-License-Identifier: GPL-2.0-or-later
|
|
*/
|
|
|
|
#ifndef MARKDOWN_CONVERTER_H
|
|
#define MARKDOWN_CONVERTER_H
|
|
|
|
#include <core/textdocumentgenerator.h>
|
|
|
|
#include <QDir>
|
|
#include <QTextFragment>
|
|
|
|
class QTextBlock;
|
|
class QTextFrame;
|
|
|
|
namespace Markdown
|
|
{
|
|
class Converter : public Okular::TextDocumentConverter
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
Converter();
|
|
~Converter() override;
|
|
|
|
QTextDocument *convert(const QString &fileName) override;
|
|
|
|
void convertAgain();
|
|
|
|
void setFancyPantsEnabled(bool b)
|
|
{
|
|
m_isFancyPantsEnabled = b;
|
|
}
|
|
bool isFancyPantsEnabled() const
|
|
{
|
|
return m_isFancyPantsEnabled;
|
|
}
|
|
|
|
QTextDocument *convertOpenFile();
|
|
|
|
private:
|
|
void extractLinks(QTextFrame *parent, QHash<QString, QTextFragment> &internalLinks, QHash<QString, QTextBlock> &documentAnchors);
|
|
void extractLinks(const QTextBlock &parent, QHash<QString, QTextFragment> &internalLinks, QHash<QString, QTextBlock> &documentAnchors);
|
|
void convertImages(QTextFrame *parent, const QDir &dir, QTextDocument *textDocument);
|
|
void convertImages(const QTextBlock &parent, const QDir &dir, QTextDocument *textDocument);
|
|
void setImageSize(QTextImageFormat &format, const qreal specifiedWidth, const qreal specifiedHeight, const qreal originalWidth, const qreal originalHeight);
|
|
|
|
FILE *m_markdownFile;
|
|
QDir m_fileDir;
|
|
bool m_isFancyPantsEnabled;
|
|
};
|
|
|
|
namespace detail
|
|
{
|
|
QString fixupHtmlTags(QString &&html);
|
|
}
|
|
|
|
}
|
|
|
|
#endif
|