mirror of
https://github.com/rust-lang/rust
synced 2024-09-05 16:57:03 +00:00
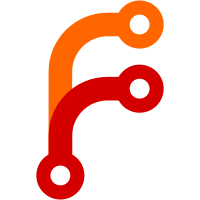
We have previously always relied upon an external tool, `ar`, to modify archives that the compiler produces (staticlibs, rlibs, etc). This approach, however, has a number of downsides: * Spawning a process is relatively expensive for small compilations * Encoding arguments across process boundaries often incurs unnecessary overhead or lossiness. For example `ar` has a tough time dealing with files that have the same name in archives, and the compiler copies many files around to ensure they can be passed to `ar` in a reasonable fashion. * Most `ar` programs found do **not** have the ability to target arbitrary platforms, so this is an extra tool which needs to be found/specified when cross compiling. The LLVM project has had a tool called `llvm-ar` for quite some time now, but it wasn't available in the standard LLVM libraries (it was just a standalone program). Recently, however, in LLVM 3.7, this functionality has been moved to a library and is now accessible by consumers of LLVM via the `writeArchive` function. This commit migrates our archive bindings to no longer invoke `ar` by default but instead make a library call to LLVM to do various operations. This solves all of the downsides listed above: * Archive management is now much faster, for example creating a "hello world" staticlib is now 6x faster (50ms => 8ms). Linking dynamic libraries also recently started requiring modification of rlibs, and linking a hello world dynamic library is now 2x faster. * The compiler is now one step closer to "hassle free" cross compilation because no external tool is needed for managing archives, LLVM does the right thing! This commit does not remove support for calling a system `ar` utility currently. We will continue to maintain compatibility with LLVM 3.5 and 3.6 looking forward (so the system LLVM can be used wherever possible), and in these cases we must shell out to a system utility. All nightly builds of Rust, however, will stop needing a system `ar`.
62 lines
2.5 KiB
Makefile
62 lines
2.5 KiB
Makefile
# Copyright 2012 The Rust Project Developers. See the COPYRIGHT
|
|
# file at the top-level directory of this distribution and at
|
|
# http://rust-lang.org/COPYRIGHT.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 <LICENSE-APACHE or
|
|
# http://www.apache.org/licenses/LICENSE-2.0> or the MIT license
|
|
# <LICENSE-MIT or http://opensource.org/licenses/MIT>, at your
|
|
# option. This file may not be copied, modified, or distributed
|
|
# except according to those terms.
|
|
|
|
######################################################################
|
|
# rustc LLVM-extensions (C++) library variables and rules
|
|
######################################################################
|
|
|
|
define DEF_RUSTLLVM_TARGETS
|
|
|
|
# FIXME: Lately, on windows, llvm-config --includedir is not enough
|
|
# to find the llvm includes (probably because we're not actually installing
|
|
# llvm, but using it straight out of the build directory)
|
|
ifdef CFG_WINDOWSY_$(1)
|
|
LLVM_EXTRA_INCDIRS_$(1)= $$(call CFG_CC_INCLUDE_$(1),$(S)src/llvm/include) \
|
|
$$(call CFG_CC_INCLUDE_$(1),\
|
|
$$(CFG_LLVM_BUILD_DIR_$(1))/include)
|
|
endif
|
|
|
|
RUSTLLVM_OBJS_CS_$(1) := $$(addprefix rustllvm/, \
|
|
ExecutionEngineWrapper.cpp RustWrapper.cpp PassWrapper.cpp \
|
|
ArchiveWrapper.cpp)
|
|
|
|
RUSTLLVM_INCS_$(1) = $$(LLVM_EXTRA_INCDIRS_$(1)) \
|
|
$$(call CFG_CC_INCLUDE_$(1),$$(LLVM_INCDIR_$(1))) \
|
|
$$(call CFG_CC_INCLUDE_$(1),$$(S)src/rustllvm/include)
|
|
RUSTLLVM_OBJS_OBJS_$(1) := $$(RUSTLLVM_OBJS_CS_$(1):rustllvm/%.cpp=$(1)/rustllvm/%.o)
|
|
|
|
# Note that we appease `cl.exe` and its need for some sort of exception
|
|
# handling flag with the `EHsc` argument here as well.
|
|
ifeq ($$(findstring msvc,$(1)),msvc)
|
|
EXTRA_RUSTLLVM_CXXFLAGS_$(1) := //EHsc
|
|
endif
|
|
|
|
$$(RT_OUTPUT_DIR_$(1))/$$(call CFG_STATIC_LIB_NAME_$(1),rustllvm): \
|
|
$$(RUSTLLVM_OBJS_OBJS_$(1))
|
|
@$$(call E, link: $$@)
|
|
$$(Q)$$(call CFG_CREATE_ARCHIVE_$(1),$$@) $$^
|
|
|
|
# On MSVC we need to double-escape arguments that llvm-config printed which
|
|
# start with a '/'. The shell we're running in will auto-translate the argument
|
|
# `/foo` to `C:/msys64/foo` but we really want it to be passed through as `/foo`
|
|
# so the argument passed to our shell must be `//foo`.
|
|
$(1)/rustllvm/%.o: $(S)src/rustllvm/%.cpp $$(MKFILE_DEPS) $$(LLVM_CONFIG_$(1))
|
|
@$$(call E, compile: $$@)
|
|
$$(Q)$$(call CFG_COMPILE_CXX_$(1), $$@,) \
|
|
$$(subst /,//,$$(LLVM_CXXFLAGS_$(1))) \
|
|
$$(EXTRA_RUSTLLVM_CXXFLAGS_$(1)) \
|
|
$$(RUSTLLVM_INCS_$(1)) \
|
|
$$<
|
|
endef
|
|
|
|
# Instantiate template for all stages
|
|
$(foreach host,$(CFG_HOST), \
|
|
$(eval $(call DEF_RUSTLLVM_TARGETS,$(host))))
|