mirror of
https://github.com/rust-lang/rust
synced 2024-10-02 06:44:33 +00:00
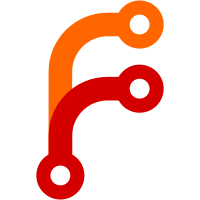
Currently, we assume that ScalarPair is always represented using a two-element struct, both as an immediate value and when stored in memory. This currently works fairly well, but runs into problems with https://github.com/rust-lang/rust/pull/116672, where a ScalarPair involving an i128 type can no longer be represented as a two-element struct in memory. For example, the tuple `(i32, i128)` needs to be represented in-memory as `{ i32, [3 x i32], i128 }` to satisfy alignment requirement. Using `{ i32, i128 }` instead will result in the second element being stored at the wrong offset (prior to LLVM 18). Resolve this issue by no longer requiring that the immediate and in-memory type for ScalarPair are the same. The in-memory type will now look the same as for normal struct types (and will include padding filler and similar), while the immediate type stays a simple two-element struct type. This also means that booleans in immediate ScalarPair are now represented as i1 rather than i8, just like we do everywhere else. The core change here is to llvm_type (which now treats ScalarPair as a normal struct) and immediate_llvm_type (which returns the two-element struct that llvm_type used to produce). The rest is fixing things up to no longer assume these are the same. In particular, this switches places that try to get pointers to the ScalarPair elements to use byte-geps instead of struct-geps.
23 lines
661 B
Rust
23 lines
661 B
Rust
// compile-flags: -C no-prepopulate-passes -Zmir-opt-level=0 -Copt-level=0
|
|
|
|
#![crate_type = "lib"]
|
|
|
|
// Hack to get the correct size for the length part in slices
|
|
// CHECK: @helper([[USIZE:i[0-9]+]] %_1)
|
|
#[no_mangle]
|
|
pub fn helper(_: usize) {
|
|
}
|
|
|
|
// CHECK-LABEL: @ref_dst
|
|
#[no_mangle]
|
|
pub fn ref_dst(s: &[u8]) {
|
|
// We used to generate an extra alloca and memcpy to ref the dst, so check that we copy
|
|
// directly to the alloca for "x"
|
|
// CHECK: store ptr %s.0, {{.*}} %x
|
|
// CHECK: [[X1:%[0-9]+]] = getelementptr inbounds i8, {{.*}} %x, {{i32 4|i64 8}}
|
|
// CHECK: store [[USIZE]] %s.1, {{.*}} [[X1]]
|
|
|
|
let x = &*s;
|
|
&x; // keep variable in an alloca
|
|
}
|