mirror of
https://github.com/rust-lang/rust
synced 2024-11-05 20:45:15 +00:00
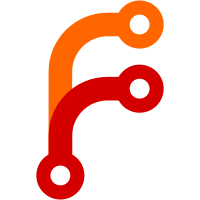
`trait_object_ty` assumed that associated types would be fully determined by the trait. This is *almost* true - const parameters and type parameters are no longer allowed, but lifetime parameters are. Since we erase all lifetime parameters anyways, instantiate it with as many erased regions as it needs. Fixes: #123053
38 lines
959 B
Rust
38 lines
959 B
Rust
// Regression test for issue 123053, where associated types with lifetimes caused generation of the
|
|
// trait object type to fail, causing an ICE.
|
|
//
|
|
//@ needs-sanitizer-cfi
|
|
//@ compile-flags: -Ccodegen-units=1 -Clto -Ctarget-feature=-crt-static -Zsanitizer=cfi --edition=2021
|
|
//@ no-prefer-dynamic
|
|
//@ only-x86_64-unknown-linux-gnu
|
|
//@ build-pass
|
|
|
|
trait Iterable {
|
|
type Item<'a>
|
|
where
|
|
Self: 'a;
|
|
type Iter<'a>: Iterator<Item = Self::Item<'a>>
|
|
where
|
|
Self: 'a;
|
|
|
|
fn iter<'a>(&'a self) -> Self::Iter<'a>;
|
|
}
|
|
|
|
impl<T> Iterable for [T] {
|
|
type Item<'a> = <std::slice::Iter<'a, T> as Iterator>::Item where T: 'a;
|
|
type Iter<'a> = std::slice::Iter<'a, T> where T: 'a;
|
|
|
|
fn iter<'a>(&'a self) -> Self::Iter<'a> {
|
|
self.iter()
|
|
}
|
|
}
|
|
|
|
fn get_first<'a, I: Iterable + ?Sized>(it: &'a I) -> Option<I::Item<'a>> {
|
|
it.iter().next()
|
|
}
|
|
|
|
fn main() {
|
|
let v = vec![1, 2, 3];
|
|
|
|
assert_eq!(Some(&1), get_first(&*v));
|
|
}
|