mirror of
https://github.com/rust-lang/rust
synced 2024-09-05 16:57:03 +00:00
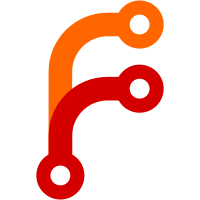
This section of code depends on `rustc_apfloat` rather than our internal types, so this is one potential ICE that we should be able to melt now. This also fixes some missing range and match handling in `rustc_middle`.
55 lines
1.3 KiB
Rust
55 lines
1.3 KiB
Rust
//@ run-pass
|
|
// Makes sure we use `==` (not bitwise) semantics for float comparison.
|
|
|
|
#![feature(f128)]
|
|
#![feature(f16)]
|
|
|
|
// FIXME(f16_f128): remove gates when ABI issues are resolved
|
|
|
|
#[cfg(all(target_arch = "aarch64", target_os = "linux"))]
|
|
fn check_f16() {
|
|
const F1: f16 = 0.0;
|
|
const F2: f16 = -0.0;
|
|
assert_eq!(F1, F2);
|
|
assert_ne!(F1.to_bits(), F2.to_bits());
|
|
assert!(matches!(F1, F2));
|
|
assert!(matches!(F2, F1));
|
|
}
|
|
|
|
fn check_f32() {
|
|
const F1: f32 = 0.0;
|
|
const F2: f32 = -0.0;
|
|
assert_eq!(F1, F2);
|
|
assert_ne!(F1.to_bits(), F2.to_bits());
|
|
assert!(matches!(F1, F2));
|
|
assert!(matches!(F2, F1));
|
|
}
|
|
|
|
fn check_f64() {
|
|
const F1: f64 = 0.0;
|
|
const F2: f64 = -0.0;
|
|
assert_eq!(F1, F2);
|
|
assert_ne!(F1.to_bits(), F2.to_bits());
|
|
assert!(matches!(F1, F2));
|
|
assert!(matches!(F2, F1));
|
|
}
|
|
|
|
#[cfg(all(target_arch = "aarch64", target_os = "linux"))]
|
|
fn check_f128() {
|
|
const F1: f128 = 0.0;
|
|
const F2: f128 = -0.0;
|
|
assert_eq!(F1, F2);
|
|
assert_ne!(F1.to_bits(), F2.to_bits());
|
|
assert!(matches!(F1, F2));
|
|
assert!(matches!(F2, F1));
|
|
}
|
|
|
|
fn main() {
|
|
#[cfg(all(target_arch = "aarch64", target_os = "linux"))]
|
|
check_f16();
|
|
check_f32();
|
|
check_f64();
|
|
#[cfg(all(target_arch = "aarch64", target_os = "linux"))]
|
|
check_f128();
|
|
}
|