mirror of
https://github.com/rust-lang/rust
synced 2024-09-05 16:57:03 +00:00
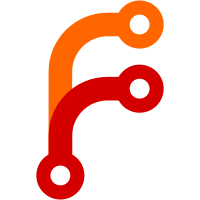
When encountering a move error on a value within a loop of any kind, identify if the moved value belongs to a call expression that should not be cloned and avoid the semantically incorrect suggestion. Also try to suggest moving the call expression outside of the loop instead. ``` error[E0382]: use of moved value: `vec` --> $DIR/recreating-value-in-loop-condition.rs:6:33 | LL | let vec = vec!["one", "two", "three"]; | --- move occurs because `vec` has type `Vec<&str>`, which does not implement the `Copy` trait LL | while let Some(item) = iter(vec).next() { | ----------------------------^^^-------- | | | | | value moved here, in previous iteration of loop | inside of this loop | note: consider changing this parameter type in function `iter` to borrow instead if owning the value isn't necessary --> $DIR/recreating-value-in-loop-condition.rs:1:17 | LL | fn iter<T>(vec: Vec<T>) -> impl Iterator<Item = T> { | ---- ^^^^^^ this parameter takes ownership of the value | | | in this function help: consider moving the expression out of the loop so it is only moved once | LL ~ let mut value = iter(vec); LL ~ while let Some(item) = value.next() { | ``` We use the presence of a `break` in the loop that would be affected by the moved value as a heuristic for "shouldn't be cloned". Fix #121466.
35 lines
785 B
Rust
35 lines
785 B
Rust
#![allow(dead_code)]
|
|
|
|
struct Events<R>(R);
|
|
|
|
struct Other;
|
|
|
|
pub trait Trait<T> {
|
|
fn handle(value: T) -> Self;
|
|
}
|
|
|
|
// Blanket impl. (If you comment this out, compiler figures out that it
|
|
// is passing an `&mut` to a method that must be expecting an `&mut`,
|
|
// and injects an auto-reborrow.)
|
|
impl<T, U> Trait<U> for T where T: From<U> {
|
|
fn handle(_: U) -> Self { unimplemented!() }
|
|
}
|
|
|
|
impl<'a, R> Trait<&'a mut Events<R>> for Other {
|
|
fn handle(_: &'a mut Events<R>) -> Self { unimplemented!() }
|
|
}
|
|
|
|
fn this_compiles<'a, R>(value: &'a mut Events<R>) {
|
|
for _ in 0..3 {
|
|
Other::handle(&mut *value);
|
|
}
|
|
}
|
|
|
|
fn this_does_not<'a, R>(value: &'a mut Events<R>) {
|
|
for _ in 0..3 {
|
|
Other::handle(value); //~ ERROR use of moved value: `value`
|
|
}
|
|
}
|
|
|
|
fn main() {}
|