mirror of
https://github.com/golang/go
synced 2024-07-20 08:14:57 +00:00
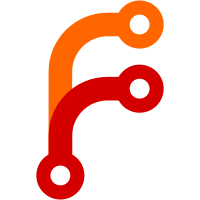
Normally, when moving Go values of type T from one location to another, we don't need to worry about partial overlaps. The two Ts must either be in disjoint (nonoverlapping) memory or in exactly the same location. There are 2 cases where this isn't true: 1) Using unsafe you can arrange partial overlaps. 2) Since Go 1.17, you can use a cast from a slice to a ptr-to-array. https://go.dev/ref/spec#Conversions_from_slice_to_array_pointer This feature can be used to construct partial overlaps of array types. var a [3]int p := (*[2]int)(a[:]) q := (*[2]int)(a[1:]) *p = *q We don't care about solving 1. Or at least, we haven't historically and no one has complained. For 2, we need to ensure that if there might be partial overlap, then we can't use OpMove; we must use memmove instead. (memmove handles partial overlap by copying in the correct direction. OpMove does not.) Note that we have to be careful here not to introduce a call when we're marshaling arguments to a call or unmarshaling results from a call. Fixes #54467 Change-Id: I1ca6aba8041576849c1d85f1fa33ae61b80a373d Reviewed-on: https://go-review.googlesource.com/c/go/+/425076 Reviewed-by: David Chase <drchase@google.com> Reviewed-by: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Run-TryBot: Keith Randall <khr@golang.org>
34 lines
865 B
Go
34 lines
865 B
Go
// errorcheck -0 -d=nil
|
|
|
|
//go:build !wasm && !aix
|
|
// +build !wasm,!aix
|
|
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test that nil checks are removed.
|
|
// Optimization is enabled.
|
|
|
|
package p
|
|
|
|
func f5(p *float32, q *float64, r *float32, s *float64) float64 {
|
|
x := float64(*p) // ERROR "removed nil check"
|
|
y := *q // ERROR "removed nil check"
|
|
*r = 7 // ERROR "removed nil check"
|
|
*s = 9 // ERROR "removed nil check"
|
|
return x + y
|
|
}
|
|
|
|
type T struct{ b [29]byte }
|
|
|
|
func f6(p, q *T) {
|
|
x := *p // ERROR "removed nil check"
|
|
*q = x // ERROR "removed nil check"
|
|
}
|
|
|
|
// make sure to remove nil check for memory move (issue #18003)
|
|
func f8(t *struct{ b [8]int }) struct{ b [8]int } {
|
|
return *t // ERROR "removed nil check"
|
|
}
|