mirror of
https://github.com/golang/go
synced 2024-09-15 22:20:06 +00:00
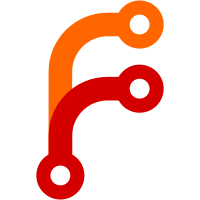
Where possible generate calls to runtime makemap with int hint argument during compile time instead of makemap with int64 hint argument. This eliminates converting the hint argument for calls to makemap with int64 hint argument for platforms where int64 values do not fit into an argument of type int. A similar optimization for makeslice was introduced in CL golang.org/cl/27851. 386: name old time/op new time/op delta NewEmptyMap 53.5ns ± 5% 41.9ns ± 5% -21.56% (p=0.000 n=10+10) NewSmallMap 182ns ± 1% 165ns ± 1% -8.92% (p=0.000 n=10+10) Change-Id: Ibd2b4c57b36f171b173bf7a0602b3a59771e6e44 Reviewed-on: https://go-review.googlesource.com/55142 Reviewed-by: Keith Randall <khr@golang.org>
35 lines
1.3 KiB
Go
35 lines
1.3 KiB
Go
// errorcheck
|
|
|
|
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Ensure that typed non-integer, negative and too large
|
|
// values are not accepted as size argument in make for
|
|
// maps.
|
|
|
|
package main
|
|
|
|
type T map[int]int
|
|
|
|
var sink T
|
|
|
|
func main() {
|
|
sink = make(T, -1) // ERROR "negative size argument in make.*"
|
|
sink = make(T, uint64(1<<63)) // ERROR "size argument too large in make.*"
|
|
|
|
sink = make(T, 0.5) // ERROR "constant 0.5 truncated to integer"
|
|
sink = make(T, 1.0)
|
|
sink = make(T, float32(1.0)) // ERROR "non-integer size argument in make.*"
|
|
sink = make(T, float64(1.0)) // ERROR "non-integer size argument in make.*"
|
|
sink = make(T, 1.0)
|
|
sink = make(T, float32(1.0)) // ERROR "non-integer size argument in make.*"
|
|
sink = make(T, float64(1.0)) // ERROR "non-integer size argument in make.*"
|
|
sink = make(T, 1+0i)
|
|
sink = make(T, complex64(1+0i)) // ERROR "non-integer size argument in make.*"
|
|
sink = make(T, complex128(1+0i)) // ERROR "non-integer size argument in make.*"
|
|
sink = make(T, 1+0i)
|
|
sink = make(T, complex64(1+0i)) // ERROR "non-integer size argument in make.*"
|
|
sink = make(T, complex128(1+0i)) // ERROR "non-integer size argument in make.*"
|
|
}
|