mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
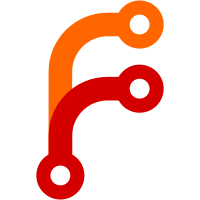
I structured the test for issue54343.go after issue46725.go, where I was careful to use `[4]int`, which is a type large enough to avoid the tiny object allocator (which interferes with finalizer semantics). But in that test, I didn't note the importance of that type, so I mistakenly used just `int` in issue54343.go. This CL switches issue54343.go to use `[4]int` too, and then adds comments to both pointing out the significance of this type. Updates #54343. Change-Id: I699b3e64b844ff6d8438bbcb4d1935615a6d8cc4 Reviewed-on: https://go-review.googlesource.com/c/go/+/423115 TryBot-Result: Gopher Robot <gobot@golang.org> Run-TryBot: Matthew Dempsky <mdempsky@google.com> Reviewed-by: David Chase <drchase@google.com>
48 lines
818 B
Go
48 lines
818 B
Go
// run
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import "runtime"
|
|
|
|
type T [4]int // N.B., [4]int avoids runtime's tiny object allocator
|
|
|
|
//go:noinline
|
|
func g(x []*T) ([]*T, []*T) { return x, x }
|
|
|
|
func main() {
|
|
const Jenny = 8675309
|
|
s := [10]*T{{Jenny}}
|
|
|
|
done := make(chan struct{})
|
|
runtime.SetFinalizer(s[0], func(p *T) { close(done) })
|
|
|
|
var h, _ interface{} = g(s[:])
|
|
|
|
if wait(done) {
|
|
panic("GC'd early")
|
|
}
|
|
|
|
if h.([]*T)[0][0] != Jenny {
|
|
panic("lost Jenny's number")
|
|
}
|
|
|
|
if !wait(done) {
|
|
panic("never GC'd")
|
|
}
|
|
}
|
|
|
|
func wait(done <-chan struct{}) bool {
|
|
for i := 0; i < 10; i++ {
|
|
runtime.GC()
|
|
select {
|
|
case <-done:
|
|
return true
|
|
default:
|
|
}
|
|
}
|
|
return false
|
|
}
|