mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
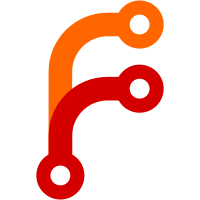
The String method is n-squared and overwrites its receiver. Fix both issues, with only a slight loss of clarity. Fixes #28773 Change-Id: I588f69d4cbd72931b28b984671512834473bd466 Reviewed-on: https://go-review.googlesource.com/c/151217 Reviewed-by: Brad Fitzpatrick <bradfitz@golang.org>
49 lines
995 B
Go
49 lines
995 B
Go
// Copyright 2009 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"sort"
|
|
)
|
|
|
|
func main() {
|
|
seq := Sequence{6, 2, -1, 44, 16}
|
|
sort.Sort(seq)
|
|
fmt.Println(seq)
|
|
}
|
|
|
|
type Sequence []int
|
|
|
|
// Methods required by sort.Interface.
|
|
func (s Sequence) Len() int {
|
|
return len(s)
|
|
}
|
|
func (s Sequence) Less(i, j int) bool {
|
|
return s[i] < s[j]
|
|
}
|
|
func (s Sequence) Swap(i, j int) {
|
|
s[i], s[j] = s[j], s[i]
|
|
}
|
|
|
|
// Copy returns a copy of the Sequence.
|
|
func (s Sequence) Copy() Sequence {
|
|
copy := make(Sequence, 0, len(s))
|
|
return append(copy, s...)
|
|
}
|
|
|
|
// Method for printing - sorts the elements before printing.
|
|
func (s Sequence) String() string {
|
|
s = s.Copy() // Make a copy; don't overwrite argument.
|
|
sort.Sort(s)
|
|
str := "["
|
|
for i, elem := range s { // Loop is O(N²); will fix that in next example.
|
|
if i > 0 {
|
|
str += " "
|
|
}
|
|
str += fmt.Sprint(elem)
|
|
}
|
|
return str + "]"
|
|
}
|