mirror of
https://github.com/golang/go
synced 2024-09-15 22:20:06 +00:00
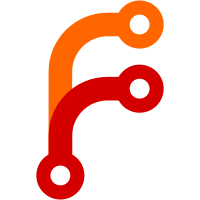
Right now we just prevent such types from being on the heap. This CL makes it so they cannot appear on the stack either. The distinction between heap and stack is pretty vague at the language level (e.g. it is affected by -N), and we don't need the flexibility anyway. Once go:notinheap types cannot be in either place, we don't need to consider pointers to such types to be pointers, at least according to the garbage collector and stack copying. (This is the big win of this CL, in my opinion.) The distinction between HasPointers and HasHeapPointer no longer exists. There is only HasPointers. This CL is cleanup before possible use of go:notinheap to fix #40954. Update #13386 Change-Id: Ibd895aadf001c0385078a6d4809c3f374991231a Reviewed-on: https://go-review.googlesource.com/c/go/+/249917 Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Matthew Dempsky <mdempsky@google.com> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com>
52 lines
935 B
Go
52 lines
935 B
Go
// errorcheck -+
|
|
|
|
// Copyright 2016 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test walk errors for go:notinheap.
|
|
|
|
package p
|
|
|
|
//go:notinheap
|
|
type nih struct {
|
|
next *nih
|
|
}
|
|
|
|
// Global variables are okay.
|
|
|
|
var x nih
|
|
|
|
// Stack variables are not okay.
|
|
|
|
func f() {
|
|
var y nih // ERROR "nih is go:notinheap; stack allocation disallowed"
|
|
x = y
|
|
}
|
|
|
|
// Heap allocation is not okay.
|
|
|
|
var y *nih
|
|
var z []nih
|
|
var w []nih
|
|
var n int
|
|
|
|
func g() {
|
|
y = new(nih) // ERROR "heap allocation disallowed"
|
|
z = make([]nih, 1) // ERROR "heap allocation disallowed"
|
|
z = append(z, x) // ERROR "heap allocation disallowed"
|
|
// Test for special case of OMAKESLICECOPY
|
|
x := make([]nih, n) // ERROR "heap allocation disallowed"
|
|
copy(x, z)
|
|
z = x
|
|
}
|
|
|
|
// Writes don't produce write barriers.
|
|
|
|
var p *nih
|
|
|
|
//go:nowritebarrier
|
|
func h() {
|
|
y.next = p.next
|
|
}
|