mirror of
https://github.com/golang/go
synced 2024-07-20 17:48:31 +00:00
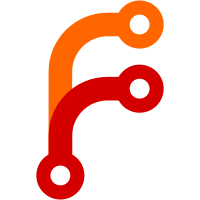
This is the last failed test in Unified IR, since it can inline f5 and f6 but the old frontend can not. So marking them as //go:noinline, with a TODO for re-enable once GOEXPERIMENT=nounified is gone. Fixes #53058 Change-Id: Ifbbc49c87997a53e1b323048f0067f0257655fad Reviewed-on: https://go-review.googlesource.com/c/go/+/437217 Reviewed-by: Dmitri Shuralyov <dmitshur@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Matthew Dempsky <mdempsky@google.com> Auto-Submit: Cuong Manh Le <cuong.manhle.vn@gmail.com> Run-TryBot: Cuong Manh Le <cuong.manhle.vn@gmail.com>
63 lines
1.5 KiB
Go
63 lines
1.5 KiB
Go
// errorcheck -0 -m
|
|
|
|
// Copyright 2010 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test, using compiler diagnostic flags, that the escape analysis is working.
|
|
// Compiles but does not run. Inlining is enabled.
|
|
|
|
package foo
|
|
|
|
var p *int
|
|
|
|
func alloc(x int) *int { // ERROR "can inline alloc" "moved to heap: x"
|
|
return &x
|
|
}
|
|
|
|
var f func()
|
|
|
|
func f1() {
|
|
p = alloc(2) // ERROR "inlining call to alloc" "moved to heap: x"
|
|
|
|
// Escape analysis used to miss inlined code in closures.
|
|
|
|
func() { // ERROR "can inline f1.func1"
|
|
p = alloc(3) // ERROR "inlining call to alloc"
|
|
}() // ERROR "inlining call to f1.func1" "inlining call to alloc" "moved to heap: x"
|
|
|
|
f = func() { // ERROR "func literal escapes to heap" "can inline f1.func2"
|
|
p = alloc(3) // ERROR "inlining call to alloc" "moved to heap: x"
|
|
}
|
|
f()
|
|
}
|
|
|
|
func f2() {} // ERROR "can inline f2"
|
|
|
|
// No inline for recover; panic now allowed to inline.
|
|
func f3() { panic(1) } // ERROR "can inline f3" "1 escapes to heap"
|
|
func f4() { recover() }
|
|
|
|
// TODO(cuonglm): remove f5, f6 //go:noinline and update the error message
|
|
// once GOEXPERIMENT=nounified is gone.
|
|
|
|
//go:noinline
|
|
func f5() *byte {
|
|
type T struct {
|
|
x [1]byte
|
|
}
|
|
t := new(T) // ERROR "new.T. escapes to heap"
|
|
return &t.x[0]
|
|
}
|
|
|
|
//go:noinline
|
|
func f6() *byte {
|
|
type T struct {
|
|
x struct {
|
|
y byte
|
|
}
|
|
}
|
|
t := new(T) // ERROR "new.T. escapes to heap"
|
|
return &t.x.y
|
|
}
|