mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
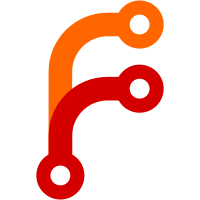
Raising an out-of-bounds panic is confusing. There's no indication that the underlying problem is a race. The runtime already does a pretty good job of detecting this kind of race (modification while iterating). We might as well just reorganize a bit to avoid the out-of-bounds panic. Fixes #33275 Change-Id: Icdd337ad2eb3c84f999db0850ec1d2ff2c146b6e Reviewed-on: https://go-review.googlesource.com/c/go/+/191197 Reviewed-by: Martin Möhrmann <moehrmann@google.com>
34 lines
542 B
Go
34 lines
542 B
Go
// skip
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"time"
|
|
)
|
|
|
|
func main() {
|
|
// Make a big map.
|
|
m := map[int]int{}
|
|
for i := 0; i < 100000; i++ {
|
|
m[i] = i
|
|
}
|
|
c := make(chan string)
|
|
go func() {
|
|
// Print the map.
|
|
s := fmt.Sprintln(m)
|
|
c <- s
|
|
}()
|
|
go func() {
|
|
time.Sleep(1 * time.Millisecond)
|
|
// Add an extra item to the map while iterating.
|
|
m[-1] = -1
|
|
c <- ""
|
|
}()
|
|
<-c
|
|
<-c
|
|
}
|