mirror of
https://github.com/golang/go
synced 2024-11-02 11:50:30 +00:00
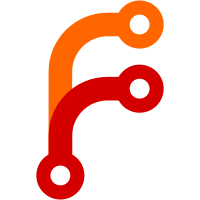
Current many architectures use a rule along the lines of // Canonicalize the order of arguments to comparisons - helps with CSE. ((CMP|CMPW) x y) && x.ID > y.ID => (InvertFlags ((CMP|CMPW) y x)) to normalize comparisons as much as possible for CSE. Replace the ID comparison with something less variable across compiler changes. This helps avoid spurious failures in some of the codegen-comparison tests (though the current choice of comparison is sensitive to Op ordering). Two tests changed to accommodate modified instruction choice. Change-Id: Ib35f450bd2bae9d4f9f7838ceaf7ec682bcf1e1a Reviewed-on: https://go-review.googlesource.com/c/go/+/280155 Trust: David Chase <drchase@google.com> Run-TryBot: David Chase <drchase@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Cherry Zhang <cherryyz@google.com>
38 lines
722 B
Go
38 lines
722 B
Go
// +build amd64
|
|
// asmcheck -gcflags=-spectre=index
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package codegen
|
|
|
|
func IndexArray(x *[10]int, i int) int {
|
|
// amd64:`CMOVQCC`
|
|
return x[i]
|
|
}
|
|
|
|
func IndexString(x string, i int) byte {
|
|
// amd64:`CMOVQLS`
|
|
return x[i]
|
|
}
|
|
|
|
func IndexSlice(x []float64, i int) float64 {
|
|
// amd64:`CMOVQLS`
|
|
return x[i]
|
|
}
|
|
|
|
func SliceArray(x *[10]int, i, j int) []int {
|
|
// amd64:`CMOVQHI`
|
|
return x[i:j]
|
|
}
|
|
|
|
func SliceString(x string, i, j int) string {
|
|
// amd64:`CMOVQHI`
|
|
return x[i:j]
|
|
}
|
|
|
|
func SliceSlice(x []float64, i, j int) []float64 {
|
|
// amd64:`CMOVQHI`
|
|
return x[i:j]
|
|
}
|