mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
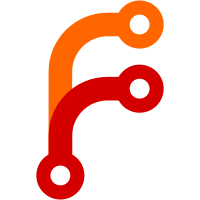
For #55326 Change-Id: I3d0ff7f820f7b2009d1b226abf701b2337fe8cbc Reviewed-on: https://go-review.googlesource.com/c/go/+/432635 TryBot-Result: Gopher Robot <gobot@golang.org> Auto-Submit: Robert Griesemer <gri@google.com> Run-TryBot: Robert Griesemer <gri@google.com> Reviewed-by: Robert Findley <rfindley@google.com> Run-TryBot: xie cui <523516579@qq.com> Reviewed-by: Robert Griesemer <gri@google.com>
46 lines
1.1 KiB
Go
46 lines
1.1 KiB
Go
// errorcheck
|
|
|
|
// Copyright 2016 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Check the compiler's switch handling that happens
|
|
// at typechecking time.
|
|
// This must be separate from other checks,
|
|
// because errors during typechecking
|
|
// prevent other errors from being discovered.
|
|
|
|
package main
|
|
|
|
// Verify that type switch statements with impossible cases are detected by the compiler.
|
|
func f0(e error) {
|
|
switch e.(type) {
|
|
case int: // ERROR "impossible type switch case: (int\n\t)?e \(.*type error\) cannot have dynamic type int \(missing method Error\)"
|
|
}
|
|
}
|
|
|
|
// Verify that the compiler rejects multiple default cases.
|
|
func f1(e interface{}) {
|
|
switch e {
|
|
default:
|
|
default: // ERROR "multiple defaults( in switch)?"
|
|
}
|
|
switch e.(type) {
|
|
default:
|
|
default: // ERROR "multiple defaults( in switch)?"
|
|
}
|
|
}
|
|
|
|
type I interface {
|
|
Foo()
|
|
}
|
|
|
|
type X int
|
|
|
|
func (*X) Foo() {}
|
|
func f2() {
|
|
var i I
|
|
switch i.(type) {
|
|
case X: // ERROR "impossible type switch case: (X\n\t)?i \(.*type I\) cannot have dynamic type X \(method Foo has pointer receiver\)"
|
|
}
|
|
}
|