mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
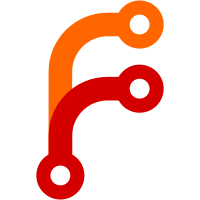
If closure in a global assignment and has a method receiver. We should assign receiver as a global variable, not a local variable. Fixes #48225 Change-Id: I8f65dd6e8baf66a5eff24028d28ad0a594091add Reviewed-on: https://go-review.googlesource.com/c/go/+/348512 Run-TryBot: Dan Scales <danscales@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Dan Scales <danscales@google.com> Reviewed-by: Keith Randall <khr@golang.org> Trust: Dan Scales <danscales@google.com>
37 lines
626 B
Go
37 lines
626 B
Go
// run -gcflags="-G=3"
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import "reflect"
|
|
|
|
type Foo[T any] struct {
|
|
val int
|
|
}
|
|
|
|
func (foo Foo[T]) Get() *T {
|
|
if foo.val != 1 {
|
|
panic("bad val field in Foo receiver")
|
|
}
|
|
return new(T)
|
|
}
|
|
|
|
var (
|
|
newInt = Foo[int]{val: 1}.Get
|
|
newString = Foo[string]{val: 1}.Get
|
|
)
|
|
|
|
func main() {
|
|
i := newInt()
|
|
s := newString()
|
|
|
|
if t := reflect.TypeOf(i).String(); t != "*int" {
|
|
panic(t)
|
|
}
|
|
if t := reflect.TypeOf(s).String(); t != "*string" {
|
|
panic(t)
|
|
}
|
|
}
|