mirror of
https://github.com/golang/go
synced 2024-07-20 09:44:05 +00:00
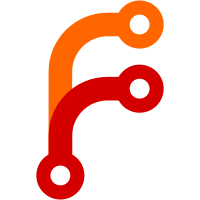
When being used by the compiler, fix up types2 error messages to be more like Go 1.17 compiler errors. In particular: - add information about which method is missing when a type is not assignable/convertible/etc. to an interface. - add information about any existing method which has the same name, but wrong type. - add extra hint in the case that the source or destination type is a pointer to an interface, rather than an interface. - add extra hint "need type assertion" in the case that the source is an interface that is implemented by the destination. - the following change in the CL stack also adds information about any existing method with a different name that only differs in case. Include much of the new logic in a new common function (*Checker).missingMethodReason(). types2 still adds a little more information in some cases then the Go 1.17 compiler. For example, it typically says "(value of type T)", rather than "(type T)", where "value" could also be "constant", "variable", etc. I kept the types2 error messages almost all the same when types2 is not used by the compiler. The only change (to reduce amount of compatibility code) was to change "M method" phrasing in one case to "method M" phrasing in one error message (which is the phrasing it uses in all other cases). That is the reason that there are a few small changes in types2/testdata/check/*.src. Added new test test/fixedbugs/issue48471.go to test that the added information is appearing correctly. Also adjusted the pattern matching in a bunch of other test/fixedbugs/*.go, now that types2 is producing error messages closer to Go 1.17. Was able to remove a couple test files from the types2 exception list in run.go. Updated #48471 Change-Id: I8af1eae6eb8a5541d8ea20b66f494e2e795e1956 Reviewed-on: https://go-review.googlesource.com/c/go/+/363436 Trust: Dan Scales <danscales@google.com> Run-TryBot: Dan Scales <danscales@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Robert Griesemer <gri@golang.org>
105 lines
2.8 KiB
Go
105 lines
2.8 KiB
Go
// errorcheck
|
|
|
|
// Copyright 2016 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test basic restrictions on type aliases.
|
|
|
|
package p
|
|
|
|
import (
|
|
"reflect"
|
|
. "reflect"
|
|
)
|
|
|
|
type T0 struct{}
|
|
|
|
// Valid type alias declarations.
|
|
|
|
type _ = T0
|
|
type _ = int
|
|
type _ = struct{}
|
|
type _ = reflect.Value
|
|
type _ = Value
|
|
|
|
type (
|
|
A0 = T0
|
|
A1 = int
|
|
A2 = struct{}
|
|
A3 = reflect.Value
|
|
A4 = Value
|
|
A5 = Value
|
|
|
|
N0 A0
|
|
)
|
|
|
|
// Methods can be declared on the original named type and the alias.
|
|
func (T0) m1() {} // GCCGO_ERROR "previous"
|
|
func (*T0) m1() {} // ERROR "method redeclared: T0\.m1|T0\.m1 redeclared in this block|redefinition of .m1."
|
|
func (A0) m1() {} // ERROR "T0\.m1 redeclared in this block|redefinition of .m1."
|
|
func (A0) m1() {} // ERROR "T0\.m1 redeclared in this block|redefinition of .m1."
|
|
func (A0) m2() {}
|
|
|
|
// Type aliases and the original type name can be used interchangeably.
|
|
var _ A0 = T0{}
|
|
var _ T0 = A0{}
|
|
|
|
// But aliases and original types cannot be used with new types based on them.
|
|
var _ N0 = T0{} // ERROR "cannot use T0{} \(type T0\) as type N0 in assignment|cannot use T0{} \(value of type T0\) as type N0 in variable declaration"
|
|
var _ N0 = A0{} // ERROR "cannot use T0{} \(type T0\) as type N0 in assignment|cannot use A0{} \(value of type T0\) as type N0 in variable declaration"
|
|
|
|
var _ A5 = Value{}
|
|
|
|
var _ interface {
|
|
m1()
|
|
m2()
|
|
} = T0{}
|
|
|
|
var _ interface {
|
|
m1()
|
|
m2()
|
|
} = A0{}
|
|
|
|
func _() {
|
|
type _ = T0
|
|
type _ = int
|
|
type _ = struct{}
|
|
type _ = reflect.Value
|
|
type _ = Value
|
|
|
|
type (
|
|
A0 = T0
|
|
A1 = int
|
|
A2 = struct{}
|
|
A3 = reflect.Value
|
|
A4 = Value
|
|
A5 Value
|
|
|
|
N0 A0
|
|
)
|
|
|
|
var _ A0 = T0{}
|
|
var _ T0 = A0{}
|
|
|
|
var _ N0 = T0{} // ERROR "cannot use T0{} \(type T0\) as type N0 in assignment|cannot use T0{} \(value of type T0\) as type N0 in variable declaration"
|
|
var _ N0 = A0{} // ERROR "cannot use T0{} \(type T0\) as type N0 in assignment|cannot use A0{} \(value of type T0\) as type N0 in variable declaration"
|
|
|
|
var _ A5 = Value{} // ERROR "cannot use reflect\.Value{} \(type reflect.Value\) as type A5 in assignment|cannot use Value{} \(value of type reflect.Value\) as type A5 in variable declaration"
|
|
}
|
|
|
|
// Invalid type alias declarations.
|
|
|
|
type _ = reflect.ValueOf // ERROR "reflect.ValueOf .*is not a type|expected type"
|
|
|
|
func (A1) m() {} // ERROR "cannot define new methods on non-local type int|may not define methods on non-local type"
|
|
func (A2) m() {} // ERROR "invalid receiver type"
|
|
func (A3) m() {} // ERROR "cannot define new methods on non-local type reflect.Value|may not define methods on non-local type"
|
|
func (A4) m() {} // ERROR "cannot define new methods on non-local type reflect.Value|may not define methods on non-local type"
|
|
|
|
type B1 = struct{}
|
|
|
|
func (B1) m() {} // ERROR "invalid receiver type"
|
|
|
|
// TODO(gri) expand
|