mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
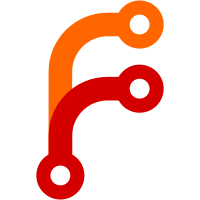
It was supposed to be testing SSA, not amd64. For #18024 Change-Id: Ibe65d7eb6bed9bc4b3eda68e1eaec5fa39fe8f76 Reviewed-on: https://go-review.googlesource.com/33491 Reviewed-by: Keith Randall <khr@golang.org> Run-TryBot: Brad Fitzpatrick <bradfitz@golang.org>
38 lines
608 B
Go
38 lines
608 B
Go
// run
|
|
|
|
// Copyright 2016 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
var out int
|
|
var zero int
|
|
|
|
func main() {
|
|
wantPanic("test1", func() {
|
|
out = 1 / zero
|
|
})
|
|
wantPanic("test2", func() {
|
|
_ = 1 / zero
|
|
})
|
|
wantPanic("test3", func() {
|
|
v := 0
|
|
_ = 1 / v
|
|
})
|
|
wantPanic("test4", func() { divby(0) })
|
|
}
|
|
|
|
func wantPanic(test string, fn func()) {
|
|
defer func() {
|
|
if e := recover(); e == nil {
|
|
panic(test + ": expected panic")
|
|
}
|
|
}()
|
|
fn()
|
|
}
|
|
|
|
//go:noinline
|
|
func divby(v int) {
|
|
_ = 1 / v
|
|
}
|