mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
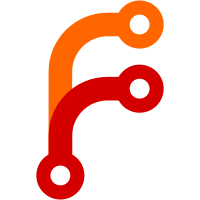
CALLPART of STRUCTLIT did not check for incomplete initialization of struct; modify PTRLIT treatment to force zeroing. Test for structlit, believe this might have also failed for arraylit. Fixes #18410. Change-Id: I511abf8ef850e300996d40568944665714efe1fc Reviewed-on: https://go-review.googlesource.com/34622 Run-TryBot: David Chase <drchase@google.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org>
40 lines
686 B
Go
40 lines
686 B
Go
// run
|
|
|
|
// Copyright 2016 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// This checks partially initialized structure literals
|
|
// used to create value.method functions have their
|
|
// non-initialized fields properly zeroed/nil'd
|
|
|
|
package main
|
|
|
|
type X struct {
|
|
A, B, C *int
|
|
}
|
|
|
|
//go:noinline
|
|
func (t X) Print() {
|
|
if t.B != nil {
|
|
panic("t.B must be nil")
|
|
}
|
|
}
|
|
|
|
//go:noinline
|
|
func caller(f func()) {
|
|
f()
|
|
}
|
|
|
|
//go:noinline
|
|
func test() {
|
|
var i, j int
|
|
x := X{A: &i, C: &j}
|
|
caller(func() { X{A: &i, C: &j}.Print() })
|
|
caller(X{A: &i, C: &j}.Print)
|
|
caller(x.Print)
|
|
}
|
|
|
|
func main() {
|
|
test()
|
|
}
|