mirror of
https://github.com/golang/go
synced 2024-07-20 05:14:32 +00:00
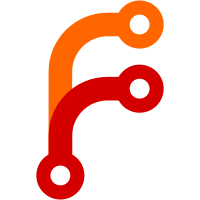
This commit allows the runtime to handle 64bits addresses returned by mmap syscall on AIX. Mmap syscall returns addresses on 59bits on AIX. But the Arena implementation only allows addresses with less than 48 bits. This commit increases the arena size up to 1<<60 for aix/ppc64. Update: #25893 Change-Id: Iea72e8a944d10d4f00be915785e33ae82dd6329e Reviewed-on: https://go-review.googlesource.com/c/138736 Reviewed-by: Austin Clements <austin@google.com>
72 lines
1.6 KiB
Go
72 lines
1.6 KiB
Go
// run
|
|
|
|
// Copyright 2009 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test the cap predeclared function applied to channels.
|
|
|
|
package main
|
|
|
|
import (
|
|
"strings"
|
|
"unsafe"
|
|
)
|
|
|
|
type T chan int
|
|
|
|
const ptrSize = unsafe.Sizeof((*byte)(nil))
|
|
|
|
func main() {
|
|
c := make(T, 10)
|
|
if len(c) != 0 || cap(c) != 10 {
|
|
println("chan len/cap ", len(c), cap(c), " want 0 10")
|
|
panic("fail")
|
|
}
|
|
|
|
for i := 0; i < 3; i++ {
|
|
c <- i
|
|
}
|
|
if len(c) != 3 || cap(c) != 10 {
|
|
println("chan len/cap ", len(c), cap(c), " want 3 10")
|
|
panic("fail")
|
|
}
|
|
|
|
c = make(T)
|
|
if len(c) != 0 || cap(c) != 0 {
|
|
println("chan len/cap ", len(c), cap(c), " want 0 0")
|
|
panic("fail")
|
|
}
|
|
|
|
n := -1
|
|
shouldPanic("makechan: size out of range", func() { _ = make(T, n) })
|
|
shouldPanic("makechan: size out of range", func() { _ = make(T, int64(n)) })
|
|
if ptrSize == 8 {
|
|
// Test mem > maxAlloc
|
|
var n2 int64 = 1 << 59
|
|
shouldPanic("makechan: size out of range", func() { _ = make(T, int(n2)) })
|
|
// Test elem.size*cap overflow
|
|
n2 = 1<<63 - 1
|
|
shouldPanic("makechan: size out of range", func() { _ = make(T, int(n2)) })
|
|
} else {
|
|
n = 1<<31 - 1
|
|
shouldPanic("makechan: size out of range", func() { _ = make(T, n) })
|
|
shouldPanic("makechan: size out of range", func() { _ = make(T, int64(n)) })
|
|
}
|
|
}
|
|
|
|
func shouldPanic(str string, f func()) {
|
|
defer func() {
|
|
err := recover()
|
|
if err == nil {
|
|
panic("did not panic")
|
|
}
|
|
s := err.(error).Error()
|
|
if !strings.Contains(s, str) {
|
|
panic("got panic " + s + ", want " + str)
|
|
}
|
|
}()
|
|
|
|
f()
|
|
}
|