mirror of
https://github.com/golang/go
synced 2024-07-20 06:47:01 +00:00
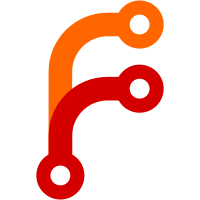
Before, an unnamed return value turned into an ONAME node n with n->sym named ~anon%d, and n->orig == n. A blank-named return value turned into an ONAME node n with n->sym named ~anon%d but n->orig == the original blank n. Code generation and printing uses n->orig, so that this node formatted as _. But some code does not use n->orig. In particular the liveness code does not know about the n->orig convention and so mishandles blank identifiers. It is possible to fix but seemed better to avoid the confusion entirely. Now the first kind of node is named ~r%d and the second ~b%d; both have n->orig == n, so that it doesn't matter whether code uses n or n->orig. After this change the ->orig field is only used for other kinds of expressions, not for ONAME nodes. This requires distinguishing ~b from ~r names in a few places that care. It fixes a liveness analysis bug without actually changing the liveness code. TBR=ken2 CC=golang-codereviews https://golang.org/cl/63630043
89 lines
1.8 KiB
Go
89 lines
1.8 KiB
Go
// errorcheck -0 -l -live
|
|
|
|
// Copyright 2014 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
func f1() {
|
|
var x *int
|
|
print(&x) // ERROR "live at call to printpointer: x$"
|
|
print(&x) // ERROR "live at call to printpointer: x$"
|
|
}
|
|
|
|
func f2(b bool) {
|
|
if b {
|
|
print(0) // nothing live here
|
|
return
|
|
}
|
|
var x *int
|
|
print(&x) // ERROR "live at call to printpointer: x$"
|
|
print(&x) // ERROR "live at call to printpointer: x$"
|
|
}
|
|
|
|
func f3(b bool) {
|
|
print(0)
|
|
if b == false {
|
|
print(0) // nothing live here
|
|
return
|
|
}
|
|
|
|
if b {
|
|
var x *int
|
|
print(&x) // ERROR "live at call to printpointer: x$"
|
|
print(&x) // ERROR "live at call to printpointer: x$"
|
|
} else {
|
|
var y *int
|
|
print(&y) // ERROR "live at call to printpointer: y$"
|
|
print(&y) // ERROR "live at call to printpointer: y$"
|
|
}
|
|
print(0) // ERROR "live at call to printint: x y$"
|
|
}
|
|
|
|
// The old algorithm treated x as live on all code that
|
|
// could flow to a return statement, so it included the
|
|
// function entry and code above the declaration of x
|
|
// but would not include an indirect use of x in an infinite loop.
|
|
// Check that these cases are handled correctly.
|
|
|
|
func f4(b1, b2 bool) { // x not live here
|
|
if b2 {
|
|
print(0) // x not live here
|
|
return
|
|
}
|
|
var z **int
|
|
x := new(int)
|
|
*x = 42
|
|
z = &x
|
|
print(**z) // ERROR "live at call to printint: x z$"
|
|
if b2 {
|
|
print(1) // ERROR "live at call to printint: x$"
|
|
return
|
|
}
|
|
for {
|
|
print(**z) // ERROR "live at call to printint: x z$"
|
|
}
|
|
}
|
|
|
|
func f5(b1 bool) {
|
|
var z **int
|
|
if b1 {
|
|
x := new(int)
|
|
*x = 42
|
|
z = &x
|
|
} else {
|
|
y := new(int)
|
|
*y = 54
|
|
z = &y
|
|
}
|
|
print(**z) // ERROR "live at call to printint: x y$"
|
|
}
|
|
|
|
// confusion about the _ result used to cause spurious "live at entry to f6: _".
|
|
|
|
func f6() (_, y string) {
|
|
y = "hello"
|
|
return
|
|
}
|