mirror of
https://github.com/golang/go
synced 2024-09-05 16:04:50 +00:00
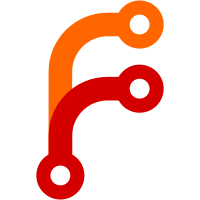
When casting an ideal to complex{64,128}, for example during the evaluation of var a = complex64(0) / 1e-50 we want the compiler to report a division-by-zero error if a divisor would be zero after the cast. We already do this for floats; for example var b = float32(0) / 1e-50 generates a 'division by zero' error at compile time (because float32(1e-50) is zero, and the cast is done before performing the division). There's no such check in the path for complex{64,128} expressions, and no cast is performed before the division in the evaluation of var a = complex64(0) / 1e-50 which compiles just fine. This patch changes the convlit1 function so that complex ideals components (real and imag) are correctly truncated to float{32,64} when doing an ideal -> complex{64, 128} cast. Fixes #11674 Change-Id: Ic5f8ee3c8cfe4c3bb0621481792c96511723d151 Reviewed-on: https://go-review.googlesource.com/37891 Run-TryBot: Alberto Donizetti <alb.donizetti@gmail.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Robert Griesemer <gri@golang.org>
41 lines
1.2 KiB
Go
41 lines
1.2 KiB
Go
// errorcheck
|
|
|
|
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Issue 11674: cmd/compile: does not diagnose constant division by
|
|
// zero
|
|
|
|
package p
|
|
|
|
const x complex64 = 0
|
|
const y complex128 = 0
|
|
|
|
var _ = x / 1e-20
|
|
var _ = x / 1e-50 // ERROR "complex division by zero"
|
|
var _ = x / 1e-1000 // ERROR "complex division by zero"
|
|
var _ = x / 1e-20i
|
|
var _ = x / 1e-50i // ERROR "complex division by zero"
|
|
var _ = x / 1e-1000i // ERROR "complex division by zero"
|
|
|
|
var _ = x / 1e-45 // smallest positive float32
|
|
|
|
var _ = x / (1e-20 + 1e-20i)
|
|
var _ = x / (1e-50 + 1e-20i)
|
|
var _ = x / (1e-20 + 1e-50i)
|
|
var _ = x / (1e-50 + 1e-50i) // ERROR "complex division by zero"
|
|
var _ = x / (1e-1000 + 1e-1000i) // ERROR "complex division by zero"
|
|
|
|
var _ = y / 1e-50
|
|
var _ = y / 1e-1000 // ERROR "complex division by zero"
|
|
var _ = y / 1e-50i
|
|
var _ = y / 1e-1000i // ERROR "complex division by zero"
|
|
|
|
var _ = y / 5e-324 // smallest positive float64
|
|
|
|
var _ = y / (1e-50 + 1e-50)
|
|
var _ = y / (1e-1000 + 1e-50i)
|
|
var _ = y / (1e-50 + 1e-1000i)
|
|
var _ = y / (1e-1000 + 1e-1000i) // ERROR "complex division by zero"
|