mirror of
https://github.com/golang/go
synced 2024-09-15 22:20:06 +00:00
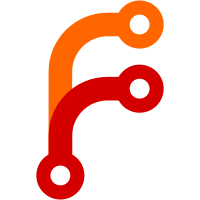
Added new struct instInfo for information about an instantiation (of a generic function/method with gcshapes or concrete types). We use this to remember the dictionary param node, the nodes where sub-dictionaries need to be used, etc. The instInfo map replaces the Stencil map in Package. Added code to access sub-dictionary entries at the appropriate call sites. We are currently still calculating the corresponding main dictionary, even when we really only need a sub-dictionary. I'll clean that up in a follow-up CL. Added code to deal with "generic" closures (closures that reference some generic variables/types). We decided that closures will share the same dictionary as the containing function (accessing the dictionary via a closure variable). So, the getGfInfo function now traverses all the nodes of each closure in a function that it is analyzing, so that a function's dictionary has all the entries needed for all its closures as well. Also, the instInfo of a closure is largely shared with its containing function. A good test for generic closures already exists with orderedmap.go. Other improvements: - Only create sub-dictionary entries when the function/method call/value or closure actually has type params in it. Added new test file subdict.go with an example where a generic method has an instantiated method call that does not depend not have type params. Change-Id: I691b9dc024a89d2305fcf1d8ba8540e53c9d103f Reviewed-on: https://go-review.googlesource.com/c/go/+/331516 Trust: Dan Scales <danscales@google.com> Run-TryBot: Dan Scales <danscales@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org>
43 lines
848 B
Go
43 lines
848 B
Go
// run -gcflags=-G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test cases where a main dictionary is needed inside a generic function/method, because
|
|
// we are calling a method on a fully-instantiated type or a fully-instantiated function.
|
|
// (probably not common situations, of course)
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
type value[T comparable] struct {
|
|
val T
|
|
}
|
|
|
|
func (v *value[T]) test(def T) bool {
|
|
return (v.val == def)
|
|
}
|
|
|
|
func (v *value[T]) get(def T) T {
|
|
var c value[int]
|
|
if c.test(32) {
|
|
return def
|
|
} else if v.test(def) {
|
|
return def
|
|
} else {
|
|
return v.val
|
|
}
|
|
}
|
|
|
|
|
|
func main() {
|
|
var s value[string]
|
|
if got, want := s.get("ab"), ""; got != want {
|
|
panic(fmt.Sprintf("get() == %d, want %d", got, want))
|
|
}
|
|
}
|