mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
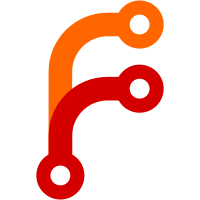
Currently, the types package has IsRuntimePkg and IsReflectPkg predicates for testing if a Pkg is the runtime or reflect packages. IsRuntimePkg returns "true" for any "CompilingRuntime" package, which includes all of the packages imported by the runtime. This isn't inherently wrong, except that all but one use of it is of the form "is this Sym a specific runtime.X symbol?" for which we clearly only want the package "runtime" itself. IsRuntimePkg was introduced (as isRuntime) in CL 37538 as part of separating the real runtime package from the compiler built-in fake runtime package. As of that CL, the "runtime" package couldn't import any other packages, so this was adequate at the time. We could fix this by just changing the implementation of IsRuntimePkg, but the meaning of this API is clearly somewhat ambiguous. Instead, we replace it with a new RuntimeSymName function that returns the name of a symbol if it's in package "runtime", or "" if not. This is what every call site (except one) actually wants, which lets us simplify the callers, and also more clearly addresses the ambiguity between package "runtime" and the general concept of a runtime package. IsReflectPkg doesn't have the same issue of ambiguity, but it parallels IsRuntimePkg and is used in the same way, so we replace it with a new ReflectSymName for consistency. Change-Id: If3a81d7d11732a9ab2cac9488d17508415cfb597 Reviewed-on: https://go-review.googlesource.com/c/go/+/521696 Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com> Reviewed-by: Matthew Dempsky <mdempsky@google.com> Run-TryBot: Austin Clements <austin@google.com> TryBot-Result: Gopher Robot <gobot@golang.org>
96 lines
1.3 KiB
Go
96 lines
1.3 KiB
Go
// errorcheck -+ -p=runtime
|
|
|
|
// Copyright 2016 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test go:nowritebarrier and related directives.
|
|
// This must appear to be in package runtime so the compiler
|
|
// recognizes "systemstack".
|
|
|
|
package runtime
|
|
|
|
type t struct {
|
|
f *t
|
|
}
|
|
|
|
var x t
|
|
var y *t
|
|
|
|
//go:nowritebarrier
|
|
func a1() {
|
|
x.f = y // ERROR "write barrier prohibited"
|
|
a2() // no error
|
|
}
|
|
|
|
//go:noinline
|
|
func a2() {
|
|
x.f = y
|
|
}
|
|
|
|
//go:nowritebarrierrec
|
|
func b1() {
|
|
b2()
|
|
}
|
|
|
|
//go:noinline
|
|
func b2() {
|
|
x.f = y // ERROR "write barrier prohibited by caller"
|
|
}
|
|
|
|
// Test recursive cycles through nowritebarrierrec and yeswritebarrierrec.
|
|
|
|
//go:nowritebarrierrec
|
|
func c1() {
|
|
c2()
|
|
}
|
|
|
|
//go:yeswritebarrierrec
|
|
func c2() {
|
|
c3()
|
|
}
|
|
|
|
func c3() {
|
|
x.f = y
|
|
c4()
|
|
}
|
|
|
|
//go:nowritebarrierrec
|
|
func c4() {
|
|
c2()
|
|
}
|
|
|
|
//go:nowritebarrierrec
|
|
func d1() {
|
|
d2()
|
|
}
|
|
|
|
func d2() {
|
|
d3()
|
|
}
|
|
|
|
//go:noinline
|
|
func d3() {
|
|
x.f = y // ERROR "write barrier prohibited by caller"
|
|
d4()
|
|
}
|
|
|
|
//go:yeswritebarrierrec
|
|
func d4() {
|
|
d2()
|
|
}
|
|
|
|
//go:noinline
|
|
func systemstack(func()) {}
|
|
|
|
//go:nowritebarrierrec
|
|
func e1() {
|
|
systemstack(e2)
|
|
systemstack(func() {
|
|
x.f = y // ERROR "write barrier prohibited by caller"
|
|
})
|
|
}
|
|
|
|
func e2() {
|
|
x.f = y // ERROR "write barrier prohibited by caller"
|
|
}
|