mirror of
https://github.com/golang/go
synced 2024-11-02 15:31:08 +00:00
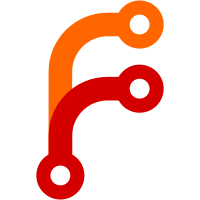
The compiler renames anonymous and blank result parameters to ~rN or ~bN, but the current semantics for computing N are rather annoying and difficult to reproduce cleanly. They also lead to difficult to read escape analysis results in tests. This CL changes N to always be calculated as the parameter's index within the function's result parameter tuple. E.g., if a function has a single result, it will now always be named "~r0". The normative change to this CL is fairly simple, but it requires updating a lot of test expectations. Change-Id: I58a3c94de00cb822cb94efe52d115531193c993c Reviewed-on: https://go-review.googlesource.com/c/go/+/323010 Trust: Matthew Dempsky <mdempsky@google.com> Trust: Dan Scales <danscales@google.com> Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Dan Scales <danscales@google.com>
54 lines
1.3 KiB
Go
54 lines
1.3 KiB
Go
// errorcheck -0 -m -l
|
|
|
|
// Copyright 2015 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test escape analysis for function parameters.
|
|
|
|
// In this test almost everything is BAD except the simplest cases
|
|
// where input directly flows to output.
|
|
|
|
package foo
|
|
|
|
func f(buf []byte) []byte { // ERROR "leaking param: buf to result ~r0 level=0$"
|
|
return buf
|
|
}
|
|
|
|
func g(*byte) string
|
|
|
|
func h(e int) {
|
|
var x [32]byte // ERROR "moved to heap: x$"
|
|
g(&f(x[:])[0])
|
|
}
|
|
|
|
type Node struct {
|
|
s string
|
|
left, right *Node
|
|
}
|
|
|
|
func walk(np **Node) int { // ERROR "leaking param content: np"
|
|
n := *np
|
|
w := len(n.s)
|
|
if n == nil {
|
|
return 0
|
|
}
|
|
wl := walk(&n.left)
|
|
wr := walk(&n.right)
|
|
if wl < wr {
|
|
n.left, n.right = n.right, n.left // ERROR "ignoring self-assignment"
|
|
wl, wr = wr, wl
|
|
}
|
|
*np = n
|
|
return w + wl + wr
|
|
}
|
|
|
|
// Test for bug where func var f used prototype's escape analysis results.
|
|
func prototype(xyz []string) {} // ERROR "xyz does not escape"
|
|
func bar() {
|
|
var got [][]string
|
|
f := prototype
|
|
f = func(ss []string) { got = append(got, ss) } // ERROR "leaking param: ss" "func literal does not escape"
|
|
s := "string"
|
|
f([]string{s}) // ERROR "\[\]string{...} escapes to heap"
|
|
}
|