mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
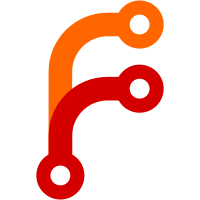
Except unsafe.Pointer. It has a different Kind, which makes it trickier. Change-Id: I12582afb6e591bea35da9e43ac8d141ed19532a3 Reviewed-on: https://go-review.googlesource.com/c/go/+/338749 Trust: Keith Randall <khr@golang.org> Trust: Dan Scales <danscales@google.com> Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Dan Scales <danscales@google.com>
50 lines
869 B
Go
50 lines
869 B
Go
// run -gcflags=-G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
type I interface {
|
|
foo() int
|
|
}
|
|
|
|
// There should be one instantiation of f for both squarer and doubler.
|
|
// Similarly, there should be one instantiation of f for both *incrementer and *decrementer.
|
|
func f[T I](x T) int {
|
|
return x.foo()
|
|
}
|
|
|
|
type squarer int
|
|
|
|
func (x squarer) foo() int {
|
|
return int(x*x)
|
|
}
|
|
|
|
type doubler int
|
|
|
|
func (x doubler) foo() int {
|
|
return int(2*x)
|
|
}
|
|
|
|
type incrementer int16
|
|
|
|
func (x *incrementer) foo() int {
|
|
return int(*x+1)
|
|
}
|
|
|
|
type decrementer int32
|
|
|
|
func (x *decrementer) foo() int{
|
|
return int(*x-1)
|
|
}
|
|
|
|
func main() {
|
|
println(f(squarer(5)))
|
|
println(f(doubler(5)))
|
|
var i incrementer = 5
|
|
println(f(&i))
|
|
var d decrementer = 5
|
|
println(f(&d))
|
|
}
|