mirror of
https://github.com/golang/go
synced 2024-09-19 07:52:34 +00:00
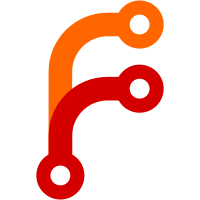
This CL adds a step to the build procedure for cgo programs. It uses 'ld -r' to combine all gcc compiled object file and generate a relocatable object file for our ld. Additionally, this linking step will combine some static linking gcc library into the relocatable object file, so that we can use libgcc, libmingwex and libmingw32 without problem. Fixes #3261. Fixes #1741. Added a testcase for linking in libgcc. TODO: 1. still need to fix the INDIRECT_SYMBOL_LOCAL problem on Darwin/386. 2. still need to enable the libgcc test on Linux/ARM, because 5l can't deal with thumb libgcc. Tested on Darwin/amd64, Darwin/386, FreeBSD/amd64, FreeBSD/386, Linux/amd64, Linux/386, Linux/ARM, Windows/amd64, Windows/386 R=iant, rsc, bradfitz, coldredlemur CC=golang-dev https://golang.org/cl/5822049
44 lines
991 B
Go
44 lines
991 B
Go
// Copyright 2012 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package cgotest
|
|
|
|
/*
|
|
// libgcc on ARM might be compiled as thumb code, but our 5l
|
|
// can't handle that, so we have to disable this test on arm.
|
|
#ifdef __ARMEL__
|
|
#include <stdio.h>
|
|
int vabs(int x) {
|
|
puts("testLibgcc is disabled on ARM because 5l cannot handle thumb library.");
|
|
return (x < 0) ? -x : x;
|
|
}
|
|
#else
|
|
int __absvsi2(int); // dummy prototype for libgcc function
|
|
// we shouldn't name the function abs, as gcc might use
|
|
// the builtin one.
|
|
int vabs(int x) { return __absvsi2(x); }
|
|
#endif
|
|
*/
|
|
import "C"
|
|
|
|
import "testing"
|
|
|
|
func testLibgcc(t *testing.T) {
|
|
var table = []struct {
|
|
in, out C.int
|
|
}{
|
|
{0, 0},
|
|
{1, 1},
|
|
{-42, 42},
|
|
{1000300, 1000300},
|
|
{1 - 1<<31, 1<<31 - 1},
|
|
}
|
|
for _, v := range table {
|
|
if o := C.vabs(v.in); o != v.out {
|
|
t.Fatalf("abs(%d) got %d, should be %d", v.in, o, v.out)
|
|
return
|
|
}
|
|
}
|
|
}
|