mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
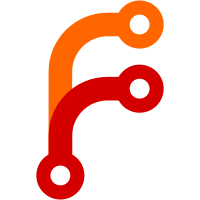
Unlike function calls, when processing instructions that directly fault we must not subtract 1 from the pc before looking up the file/line information. Since the file/line lookup unconditionally subtracts 1, add 1 to the faulting instruction PCs to compensate. Fixes #34123 Change-Id: Ie7361e3d2f84a0d4f48d97e5a9e74f6291ba7a8b Reviewed-on: https://go-review.googlesource.com/c/go/+/196962 Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Emmanuel Odeke <emm.odeke@gmail.com>
43 lines
735 B
Go
43 lines
735 B
Go
// run
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Make sure that the line number is reported correctly
|
|
// for faulting instructions.
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"runtime"
|
|
)
|
|
|
|
var x byte
|
|
var p *byte
|
|
|
|
//go:noinline
|
|
func f() {
|
|
q := p
|
|
x = 11 // line 23
|
|
*q = 12 // line 24
|
|
}
|
|
func main() {
|
|
defer func() {
|
|
recover()
|
|
var pcs [10]uintptr
|
|
n := runtime.Callers(1, pcs[:])
|
|
frames := runtime.CallersFrames(pcs[:n])
|
|
for {
|
|
f, more := frames.Next()
|
|
if f.Function == "main.f" && f.Line != 24 {
|
|
panic(fmt.Errorf("expected line 24, got line %d", f.Line))
|
|
}
|
|
if !more {
|
|
break
|
|
}
|
|
}
|
|
}()
|
|
f()
|
|
}
|