mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
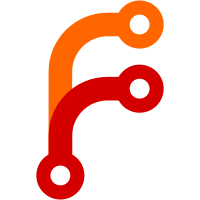
In this test, traditionally the comparison `*l == r[0]` was left as a comparison between `*l` (type `any`) and `r[0]` (type `*int`), and the rest of the compiler needed to handle mixed-typed comparisons. However, this means more complexity for wiring up explicit rtypes. To simplify rtype handling, the next CL will change unified IR to instead handle the expression as `*l == any(r[0])`. However, a consequence of this currently is that walk will now sequence the `any(r[0])` expression first, because it involves a concrete-to-interface conversion. And in turn, this means the `r[0]` panic ("index out of bounds") will take priority over the `*l` panic ("nil pointer dereference"). This is a change in user-visible semantics in some cases, but the Go spec leaves this unspecified, so it shouldn't be an issue. Note also: gccgo has the same behavior (i.e., panicking on index out of bounds, not nil pointer dereference), and cmd/compile also already has the same behavior when the interface conversion is explicit (as in the added "nil pointer dereference #3" test case). Updates #23735. Updates #32187. Change-Id: I49e5dcca85b4680f9c8780ef0013e64254d38fe5 Reviewed-on: https://go-review.googlesource.com/c/go/+/418097 Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com> Reviewed-by: David Chase <drchase@google.com>
62 lines
1.5 KiB
Go
62 lines
1.5 KiB
Go
// run
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// short-circuiting interface-to-concrete comparisons
|
|
// will not miss panics
|
|
|
|
package main
|
|
|
|
import (
|
|
"log"
|
|
"strings"
|
|
)
|
|
|
|
func main() {
|
|
var (
|
|
x interface{}
|
|
p *int
|
|
s []int
|
|
l *interface{}
|
|
r []*int
|
|
)
|
|
tests := []struct {
|
|
name string
|
|
errStr string
|
|
f func()
|
|
}{
|
|
{"switch case", "", func() {
|
|
switch x {
|
|
case x.(*int):
|
|
}
|
|
}},
|
|
{"interface conversion", "", func() { _ = x == x.(error) }},
|
|
{"type assertion", "", func() { _ = x == x.(*int) }},
|
|
{"out of bounds", "", func() { _ = x == s[1] }},
|
|
{"nil pointer dereference #1", "", func() { _ = x == *p }},
|
|
// TODO(mdempsky): Restore "nil pointer dereference" check. The Go
|
|
// spec doesn't mandate an order for panics (or even panic
|
|
// messages), but left-to-right is less confusing to users.
|
|
{"nil pointer dereference #2", "", func() { _ = *l == r[0] }},
|
|
{"nil pointer dereference #3", "", func() { _ = *l == any(r[0]) }},
|
|
}
|
|
|
|
for _, tc := range tests {
|
|
testFuncShouldPanic(tc.name, tc.errStr, tc.f)
|
|
}
|
|
}
|
|
|
|
func testFuncShouldPanic(name, want string, f func()) {
|
|
defer func() {
|
|
e := recover()
|
|
if e == nil {
|
|
log.Fatalf("%s: comparison did not panic\n", name)
|
|
}
|
|
if have := e.(error).Error(); !strings.Contains(have, want) {
|
|
log.Fatalf("%s: wrong panic message: have %q, want %q\n", name, have, want)
|
|
}
|
|
}()
|
|
f()
|
|
}
|